How to Get Keyboard Input in Pygame
-
Use the
event
Loop to Get Keyboard Input in Pygame -
Use
pygame.key.get_pressed()
to Get Keyboard Input in Pygame
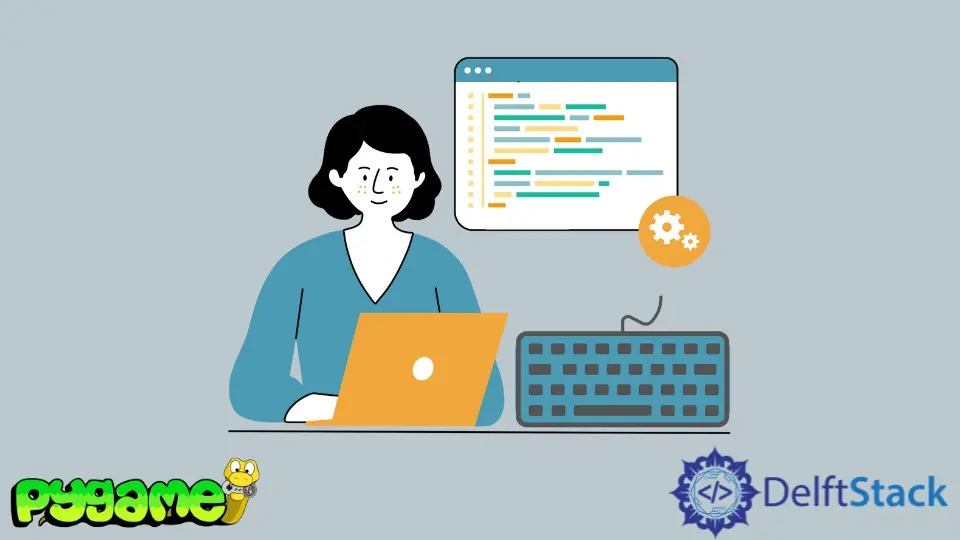
This tutorial teaches you how to get keyboard input in Pygame.
The code displayed here is not the complete code for a valid Pygame window. If you are interested in a bare-bones framework, consult this article.
There are two ways to get keyboard input in Pygame: the event
loop and pygame.key.get_pressed()
. They have one significant difference, which will be explained later.
Use the event
Loop to Get Keyboard Input in Pygame
We already used the event
loop if you read the article to get yourself a Pygame framework because we handled the exit request of the window there.
We loop over an array returned by the pygame.event.get()
method. It contains all events that happened in this frame.
In the following line, we check for its type. There are different types, and the most used are KEYDOWN
and KEYUP
.
As you see below, we also check for the KEYDOWN
event. After that, we checked whether the key was E or not.
If it was, then it will print E Pressed
. This will only evaluate to true on the frame the key was pressed down, not when it is held down.
To do that, we need pygame.key.get_pressed()
. And in the next part, its usage is described.
Code snippet:
# Main Loop
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_e:
print("E Pressed")
Use pygame.key.get_pressed()
to Get Keyboard Input in Pygame
Using this, we get the state of the key at the current frame. This means we also get a signal when the key is held down and not only when it is pressed for the first time.
Below you will find a simple example of this. First, we get an array with all the pressed keys at this frame with the pygame.key.get_pressed
method, and then we will see whether the key was pressed.
We get this value with one of the key constants in Pygame. This will print W Pressed
if the W key is pressed down.
Code snippet:
keys = pygame.key.get_pressed()
if keys[pygame.K_w]:
print("W Pressed")
Complete Example Code
# Imports
import sys
import pygame
# Configuration
pygame.init()
fps = 60
fpsClock = pygame.time.Clock()
width, height = 640, 480
screen = pygame.display.set_mode((width, height))
# Game loop.
while True:
screen.fill((20, 20, 20))
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_e:
print("E Pressed")
keys = pygame.key.get_pressed()
if keys[pygame.K_w]:
print("W Pressed")
pygame.display.flip()
fpsClock.tick(fps)
Hi, my name is Maxim Maeder, I am a young programming enthusiast looking to have fun coding and teaching you some things about programming.
GitHub