How to Take Column-Slices of DataFrame in Pandas
-
Use
loc()
to Slice Columns in Pandas DataFrame -
Use
iloc()
to Slice Columns in Pandas DataFrame -
Use
redindex()
to Slice Columns in Pandas DataFrame
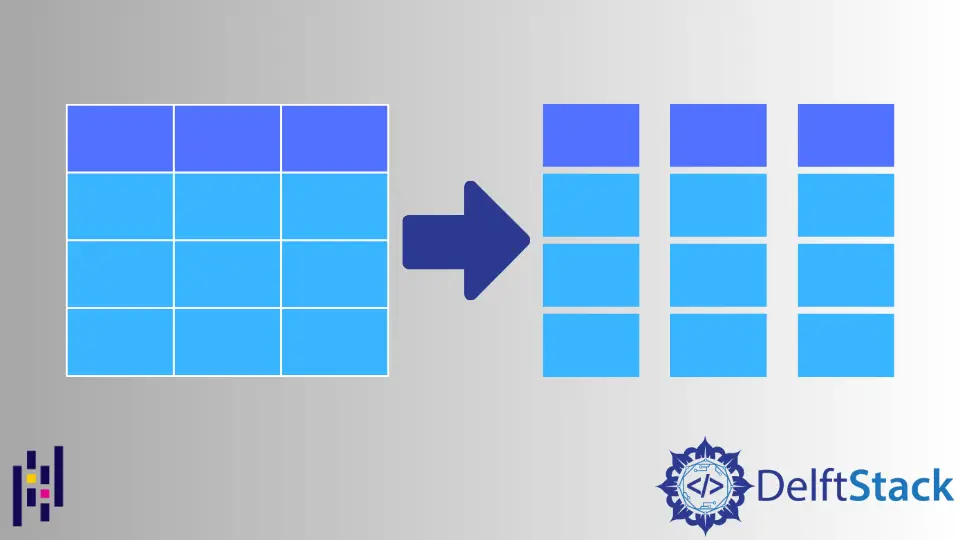
Column-slicing in Pandas allows us to slice the dataframe into subsets, which means it creates a new Pandas dataframe from the original with only the required columns. We will work with the following dataframe as an example for column-slicing.
import pandas as pd
import numpy as np
df = pd.DataFrame(np.random.rand(4, 4), columns=["a", "b", "c", "d"])
print(df)
Output:
a b c d
0 0.797321 0.468894 0.335781 0.956516
1 0.546303 0.567301 0.955228 0.557812
2 0.385315 0.706735 0.058784 0.578468
3 0.751037 0.248284 0.172229 0.493763
Use loc()
to Slice Columns in Pandas DataFrame
Pandas library provides us with more than one method to carry out column-slicing. The first one is using the loc()
function.
Pandas loc()
function allows us to access the elements of a dataframe using column names or index labels. The syntax for column slicing using loc()
:
dataframe.loc[:, [columns]]
Example:
import pandas as pd
import numpy as np
df = pd.DataFrame(np.random.rand(4, 4), columns=["a", "b", "c", "d"])
df1 = df.loc[:, "a":"c"] # Returns a new dataframe with columns a,b and c
print(df1)
Output:
a b c
0 0.344952 0.611792 0.213331
1 0.907322 0.992097 0.080447
2 0.471611 0.625846 0.348778
3 0.656921 0.999646 0.976743
Use iloc()
to Slice Columns in Pandas DataFrame
We can also use the iloc()
function to access elements of a dataframe using the integer index of rows and columns. The syntax for column slicing using iloc()
:
dataframe.iloc[:, [column - index]]
Example:
import pandas as pd
import numpy as np
df = pd.DataFrame(np.random.rand(4, 4), columns=["a", "b", "c", "d"])
df1 = df.iloc[:, 0:2] # Returns a new dataframe with first two columns
print(df1)
Output:
a b
0 0.034587 0.070249
1 0.648231 0.721517
2 0.485168 0.548045
3 0.377612 0.310408
Use redindex()
to Slice Columns in Pandas DataFrame
The reindex()
function can also be used for altering the indexes of the dataframe and can be used for column slicing. The reindex()
function can take in many arguments but for column-slicing, we will only need to provide the function with the column names.
The syntax for column-slicing using reindex()
:
dataframe.reindex(columns=[column_names])
Example:
import pandas as pd
import numpy as np
df = pd.DataFrame(np.random.rand(4, 4), columns=["a", "b", "c", "d"])
# Returns a new dataframe with c and b columns
df1 = df.reindex(columns=["c", "b"])
print(df1)
Output:
c b
0 0.429790 0.962838
1 0.605381 0.463617
2 0.922489 0.733338
3 0.741352 0.118478
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn