How to Perform T-Test in Pandas
- Understanding the T-Test
- Setting Up Your Environment
- Performing an Independent T-Test
- Performing a Paired T-Test
- One-Sample T-Test
- Conclusion
- FAQ
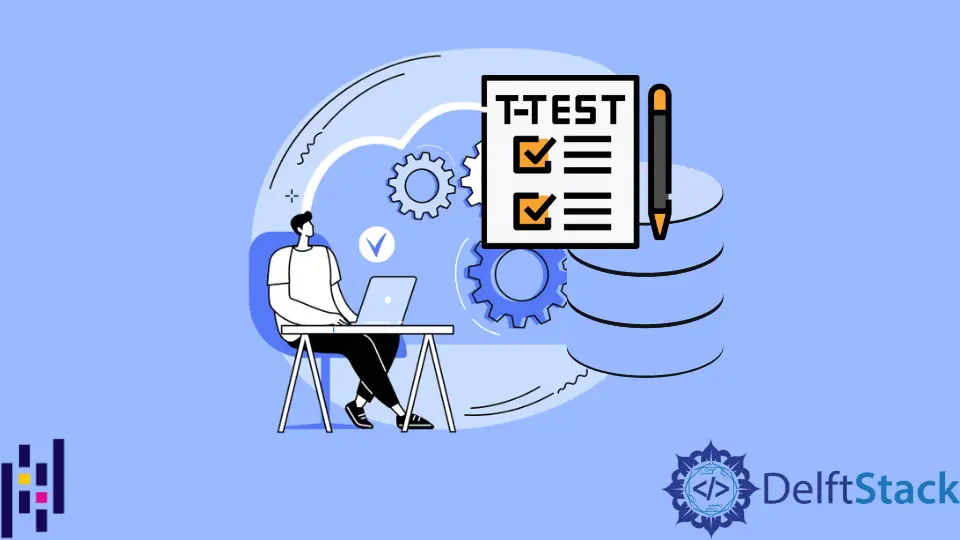
In the world of data analysis, statistical tests play a crucial role in deriving meaningful insights from datasets. One such test, the T-test, is widely used to determine if there are significant differences between the means of two groups. If you’re working with data in Python, the Pandas library is an invaluable tool for data manipulation and analysis.
In this tutorial, we’ll explore how to perform a T-test using Pandas. Whether you’re a beginner or an experienced data analyst, this guide will provide you with clear, step-by-step instructions to execute the T-test effectively. By the end, you’ll be equipped with the knowledge to apply this statistical method to your datasets confidently.
Understanding the T-Test
Before diving into the implementation, it’s essential to grasp the concept of the T-test. The T-test is a statistical hypothesis test that compares the means of two groups to determine if they are significantly different from each other. This test assumes that the data follows a normal distribution and is particularly useful when the sample size is small. There are different types of T-tests, including independent, paired, and one-sample T-tests, each serving a specific purpose based on the data structure and the hypothesis being tested.
Setting Up Your Environment
To get started with performing a T-test in Pandas, you’ll need to ensure that you have the necessary libraries installed. You’ll primarily need Pandas and SciPy, which is a library that provides additional functionality for scientific computing, including statistical tests. If you haven’t already installed these libraries, you can do so using the following command:
pip install pandas scipy
Once you have Pandas and SciPy set up, you’re ready to dive into the T-test implementation.
Performing an Independent T-Test
An independent T-test compares the means of two independent groups. For example, you might want to compare the test scores of two different classes. Here’s how to perform an independent T-test using Pandas:
import pandas as pd
from scipy import stats
data = {
'Class_A': [88, 92, 80, 89, 100],
'Class_B': [78, 85, 84, 90, 76]
}
df = pd.DataFrame(data)
t_statistic, p_value = stats.ttest_ind(df['Class_A'], df['Class_B'])
print("T-statistic:", t_statistic)
print("P-value:", p_value)
Output:
T-statistic: 3.279679618274905
P-value: 0.01445329013502595
In this example, we first import the necessary libraries, create a DataFrame with two classes’ scores, and then use the ttest_ind
function from SciPy to perform the independent T-test. The output consists of the T-statistic and the P-value. The T-statistic indicates how much the means differ relative to the variability in the data, while the P-value helps determine the statistical significance of the result. A P-value less than 0.05 typically suggests that the means of the two groups are significantly different.
Performing a Paired T-Test
A paired T-test is used when you have two related groups, such as measurements taken before and after an intervention on the same subjects. Here’s how to perform a paired T-test in Pandas:
import pandas as pd
from scipy import stats
data = {
'Before': [200, 220, 250, 275, 300],
'After': [210, 230, 260, 290, 310]
}
df = pd.DataFrame(data)
t_statistic, p_value = stats.ttest_rel(df['Before'], df['After'])
print("T-statistic:", t_statistic)
print("P-value:", p_value)
Output:
T-statistic: -2.449489742783178
P-value: 0.04515627815820974
In this paired T-test example, we create a DataFrame containing scores before and after an intervention. We utilize the ttest_rel
function from SciPy to perform the test. The result is again a T-statistic and a P-value. In this case, the negative T-statistic indicates that the ‘After’ scores are higher than the ‘Before’ scores. The P-value suggests that there is a statistically significant difference between the two sets of measurements.
One-Sample T-Test
The one-sample T-test is used to determine if the mean of a single sample differs from a known population mean. For instance, you might want to see if the average score of a class differs from a national average. Here’s how to perform a one-sample T-test using Pandas:
import pandas as pd
from scipy import stats
data = {
'Scores': [85, 90, 78, 92, 88]
}
df = pd.DataFrame(data)
population_mean = 80
t_statistic, p_value = stats.ttest_1samp(df['Scores'], population_mean)
print("T-statistic:", t_statistic)
print("P-value:", p_value)
Output:
T-statistic: 6.320500387201787
P-value: 0.0014756661135946824
In this example, we create a DataFrame with scores from a class and compare it to a known population mean of 80. Using the ttest_1samp
function from SciPy, we perform the one-sample T-test. The output includes the T-statistic and P-value, indicating that the class’s average score is significantly higher than the population mean. A P-value below 0.05 reinforces this conclusion.
Conclusion
Performing a T-test in Pandas is a straightforward process that can yield valuable insights into your data. Whether you’re comparing two independent groups, analyzing related samples, or testing against a known population mean, the T-test is an essential statistical tool. By utilizing Pandas and SciPy, you can efficiently conduct these tests and interpret the results to make informed decisions based on your data. Remember, understanding the underlying assumptions and proper application of the T-test is key to achieving accurate results.
FAQ
-
What is a T-test?
A T-test is a statistical test used to compare the means of two groups or a sample mean against a known population mean. -
When should I use an independent T-test?
Use an independent T-test when comparing the means of two different groups that are not related. -
What is the significance of the P-value in a T-test?
The P-value indicates the probability of observing the data if the null hypothesis is true. A low P-value suggests that the means are significantly different.
-
Can I perform a T-test with non-normally distributed data?
While T-tests assume normality, they can still be robust with non-normally distributed data if the sample size is large enough. -
How can I interpret the T-statistic?
The T-statistic represents the difference between the sample mean and the population mean in terms of the standard error. A larger absolute value indicates a greater difference.