How to Subtract Two Columns of a Pandas DataFrame
-
Use the
__getitem__
Syntax ([]
) to Subtract Two Columns in Pandas - Use a Function to Subtract Two Columns in Pandas
-
Use the
assign()
Method to Subtract Two Columns in Pandas
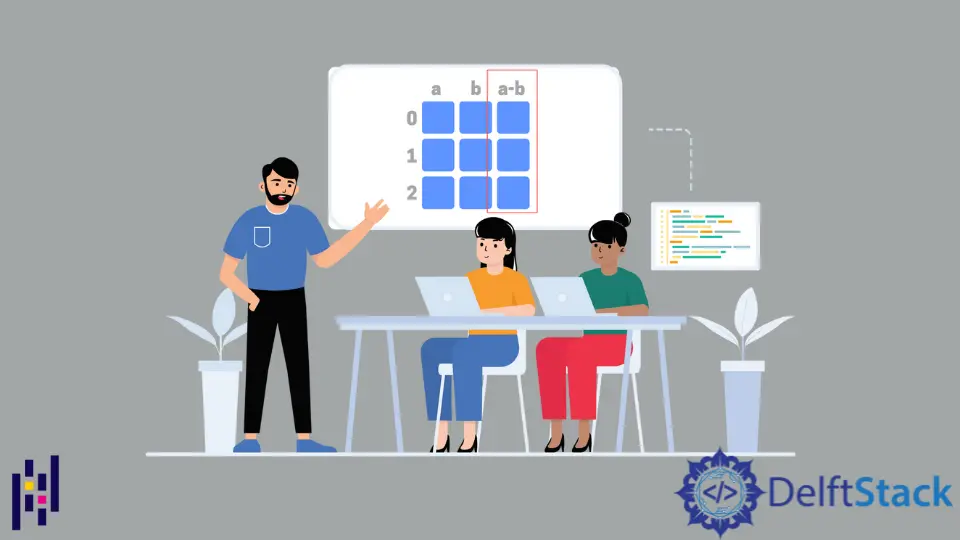
Pandas can handle large datasets and have a variety of features and operations that can be applied to the data.
One such simple operation is the subtraction of two columns and storing the result in a new column, which will be discussed in this tutorial. This simple task can be done in many ways. We will be calculating the difference between column 'a'
and 'd'
of the following DataFrame.
import pandas as pd
df = pd.DataFrame(
[[10, 6, 7, 8], [1, 9, 12, 14], [5, 8, 10, 6]], columns=["a", "b", "c", "d"]
)
print(df)
Output:
a b c d
0 10 6 7 8
1 1 9 12 14
2 5 8 10 6
Use the __getitem__
Syntax ([]
) to Subtract Two Columns in Pandas
The simplest way to subtract two columns is to access the required columns and create a new column using the __getitem__
syntax([]
). Example:
import pandas as pd
df = pd.DataFrame(
[[10, 6, 7, 8], [1, 9, 12, 14], [5, 8, 10, 6]], columns=["a", "b", "c", "d"]
)
df["d - a"] = df["d"] - df["a"]
print(df)
Output:
a b c d d - a
0 10 6 7 8 -2
1 1 9 12 14 13
2 5 8 10 6 1
Use a Function to Subtract Two Columns in Pandas
We can easily create a function to subtract two columns in Pandas and apply it to the specified columns of the DataFrame using the apply()
function. We will provide the apply()
function with the parameter axis
and set it to 1, which indicates that the function is applied to the columns.
import pandas as pd
df = pd.DataFrame(
[[10, 6, 7, 8], [1, 9, 12, 14], [5, 8, 10, 6]], columns=["a", "b", "c", "d"]
)
def x(a, b):
return a - b
df["d - a"] = df.apply(lambda f: x(f["d"], f["a"]), axis=1)
print(df)
Output:
a b c d d - a
0 10 6 7 8 -2
1 1 9 12 14 13
2 5 8 10 6 1
Since the subtraction of columns is a relatively easy operation, so we can directly use the lambda
keyword to create simple one-line functions in the apply()
function.
import pandas as pd
df = pd.DataFrame(
[[10, 6, 7, 8], [1, 9, 12, 14], [5, 8, 10, 6]], columns=["a", "b", "c", "d"]
)
df["d - a"] = df.apply(lambda x: x["d"] - x["a"], axis=1)
print(df)
Output:
a b c d d - a
0 10 6 7 8 -2
1 1 9 12 14 13
2 5 8 10 6 1
Use the assign()
Method to Subtract Two Columns in Pandas
The DataFrame assign()
method is used to add a column to the DataFrame after performing some operation. It returns a new DataFrame with all the original as well as the new columns. The following example will show how to subtract two columns using the assign()
method.
import pandas as pd
df = pd.DataFrame(
[[10, 6, 7, 8], [1, 9, 12, 14], [5, 8, 10, 6]], columns=["a", "b", "c", "d"]
)
df = df.assign(d_minus_a=df["d"] - df["a"])
print(df)
Output:
a b c d d_minus_a
0 10 6 7 8 -2
1 1 9 12 14 13
2 5 8 10 6 1
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn