How to Show All Columns of a Pandas DataFrame
- Use a List to Show All Columns of a Pandas DataFrame
- Use a NumPy Array to Show All Columns of a Pandas DataFrame
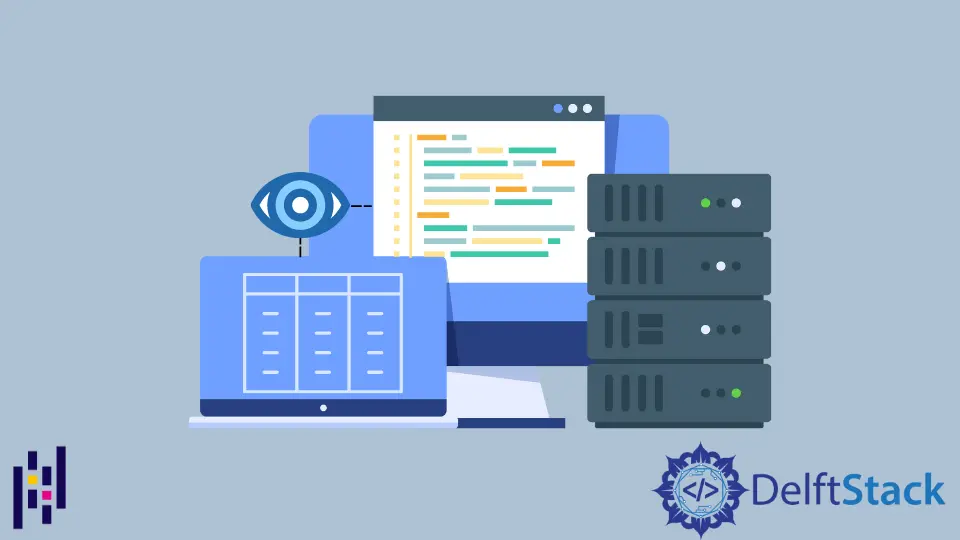
In real-life examples, we encounter large datasets containing hundreds and thousands of rows and columns. To work on such large chunks of data, we need to be familiar with the rows, columns, and type of the data. In many cases, we also need to store the names of columns for ease in extracting elements later on or for other use.
In normal situations, we usually use dataframe.columns
to extract the names of the columns of a DataFrame. This can work for small datasets, but if we are handling a DataFrame with more than a hundred columns, this method can prove to be not so efficient.
The following example will illustrate the problem with dataframe.columns
:
import pandas as pd
import numpy as np
df = pd.DataFrame(columns=np.arange(150))
print(df.columns)
type(df.columns)
Output:
Int64Index([ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9,
...
140, 141, 142, 143, 144, 145, 146, 147, 148, 149],
dtype='int64', length=150)
pandas.core.indexes.numeric.Int64Index
In the above example, we generate an empty DataFrame with 150 columns from 0-149, and as seen in the output, we are not able to view all the columns. We can only see the first few and last few column names, and the output is not a list or Series
that we can store and access easily for further use.
There is a simple fix to the above problem; we can simply convert the result of dataframe.columns
to a list or a NumPy array.
Use a List to Show All Columns of a Pandas DataFrame
For this we can use two methods, tolist()
or list()
. Both of these functions convert the column names to a list and give the same output.
Example using tolist()
:
import pandas as pd
import numpy as np
df = pd.DataFrame(columns=np.arange(150))
print(df.columns.tolist())
type(df.columns.tolist())
Output:
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22, 23, 24, 25, 26, 27, 28, 29, 30, 31, 32, 33, 34, 35, 36, 37, 38, 39, 40, 41, 42, 43, 44, 45, 46, 47, 48, 49, 50, 51, 52, 53, 54, 55, 56, 57, 58, 59, 60, 61, 62, 63, 64, 65, 66, 67, 68, 69, 70, 71, 72, 73, 74, 75, 76, 77, 78, 79, 80, 81, 82, 83, 84, 85, 86, 87, 88, 89, 90, 91, 92, 93, 94, 95, 96, 97, 98, 99, 100, 101, 102, 103, 104, 105, 106, 107, 108, 109, 110, 111, 112, 113, 114, 115, 116, 117, 118, 119, 120, 121, 122, 123, 124, 125, 126, 127, 128, 129, 130, 131, 132, 133, 134, 135, 136, 137, 138, 139, 140, 141, 142, 143, 144, 145, 146, 147, 148, 149]
list
Example using list()
:
import pandas as pd
import numpy as np
df = pd.DataFrame(columns=np.arange(150))
print(list(df.columns))
type(list(df.columns))
Output:
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22, 23, 24, 25, 26, 27, 28, 29, 30, 31, 32, 33, 34, 35, 36, 37, 38, 39, 40, 41, 42, 43, 44, 45, 46, 47, 48, 49, 50, 51, 52, 53, 54, 55, 56, 57, 58, 59, 60, 61, 62, 63, 64, 65, 66, 67, 68, 69, 70, 71, 72, 73, 74, 75, 76, 77, 78, 79, 80, 81, 82, 83, 84, 85, 86, 87, 88, 89, 90, 91, 92, 93, 94, 95, 96, 97, 98, 99, 100, 101, 102, 103, 104, 105, 106, 107, 108, 109, 110, 111, 112, 113, 114, 115, 116, 117, 118, 119, 120, 121, 122, 123, 124, 125, 126, 127, 128, 129, 130, 131, 132, 133, 134, 135, 136, 137, 138, 139, 140, 141, 142, 143, 144, 145, 146, 147, 148, 149]
list
Use a NumPy Array to Show All Columns of a Pandas DataFrame
We can use the values()
function to convert the result of dataframe.columns
to a NumPy array.
Example:
import pandas as pd
import numpy as np
df = pd.DataFrame(columns=np.arange(150))
print(df.columns.values)
type(df.columns.values)
Output:
[ 0 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35
36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53
54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71
72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89
90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107
108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125
126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143
144 145 146 147 148 149]
numpy.ndarray
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn