How to Rename Column by Index Using Pandas
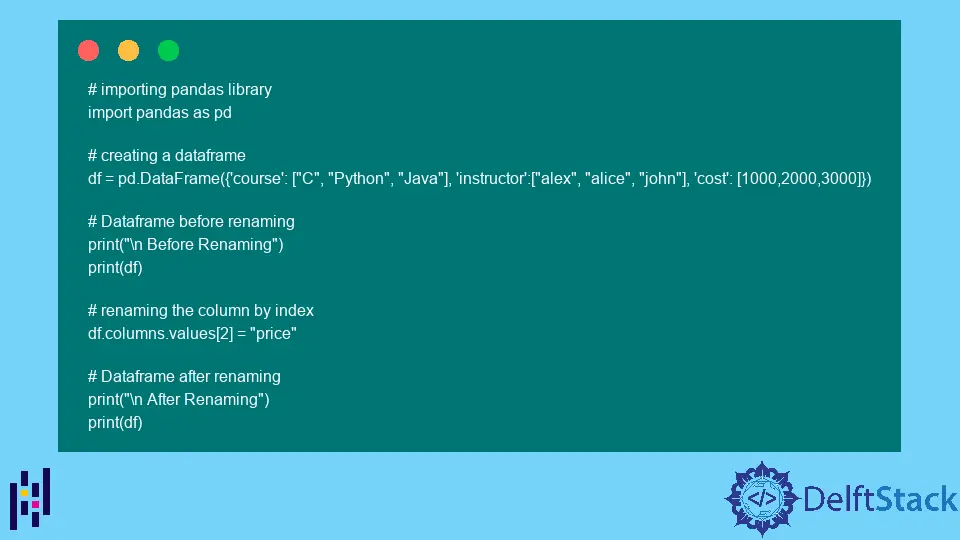
DataFrame is a 2D labeled data structure; It is a size-mutable and heterogeneous data structure. The DataFrame contains labeled axes called rows and columns.
This tutorial will tackle different methods to rename columns of a DataFrame by index using Pandas.
Use the rename()
Function in Pandas
The Pandas library provides a rename()
function that is used to rename the columns of a DataFrame. The rename()
function takes a mapper parameter.
A mapper is a dictionary-like data structure that contains the renaming column as key and name as the value that returns a DataFrame; in-place modification can be done by setting inplace = True
.
Syntax:
pandas.rename(mapper)
In this tutorial’s example, let’s use the rename()
method. First, follow the steps below.
- Import the
pandas
library. - Pass the Mapper to the
rename()
method. - The
rename()
method will return a data frame with that column renamed. - Print the DataFrame.
The following code is the implementation of the above approach.
Code:
# importing pandas library
import pandas as pd
# creating a dataframe
df = pd.DataFrame(
{
"course": ["C", "Python", "Java"],
"instructor": ["alex", "alice", "john"],
"cost": [1000, 2000, 3000],
}
)
# Dataframe before renaming
print("\n Before Renaming")
print(df)
# renaming the column by index
df = df.rename(columns={df.columns[2]: "price"})
# Dataframe after renaming
print("\n After Renaming")
print(df)
Output:
Before Renaming:
course | Mentor | cost |
---|---|---|
C | alex | 1000 |
Python | alice | 2000 |
Java | john | 3000 |
After Renaming:
course | Mentor | price |
---|---|---|
C | alex | 1000 |
Python | alice | 2000 |
Java | john | 3000 |
Use the DataFrame.column.values
in Pandas
The DataFrame.column.values
will return all the column names, and we can use the index to modify the column names. The column.values
will return an array of an index.
The following approach is the implementation of the code in this next example.
- Import the
pandas
library. - Retrieve the array of column names using
DataFrame.column.values
. - Change the name of the column by passing the index.
- Print the DataFrame.
Code:
# importing pandas library
import pandas as pd
# creating a dataframe
df = pd.DataFrame(
{
"course": ["C", "Python", "Java"],
"instructor": ["alex", "alice", "john"],
"cost": [1000, 2000, 3000],
}
)
# Dataframe before renaming
print("\n Before Renaming")
print(df)
# renaming the column by index
df.columns.values[2] = "price"
# Dataframe after renaming
print("\n After Renaming")
print(df)
Output:
Before Renaming:
course | Mentor | cost |
---|---|---|
C | alex | 1000 |
Python | alice | 2000 |
Java | john | 3000 |
After Renaming:
course | Mentor | price |
---|---|---|
C | alex | 1000 |
Python | alice | 2000 |
Java | john | 3000 |