How to Lookup From One of Multiple Columns Based on Value in Pandas
-
Method 1: Using the
apply
Function - Method 2: Boolean Indexing
-
Method 3: Using the
melt
Method - Conclusion
- FAQ
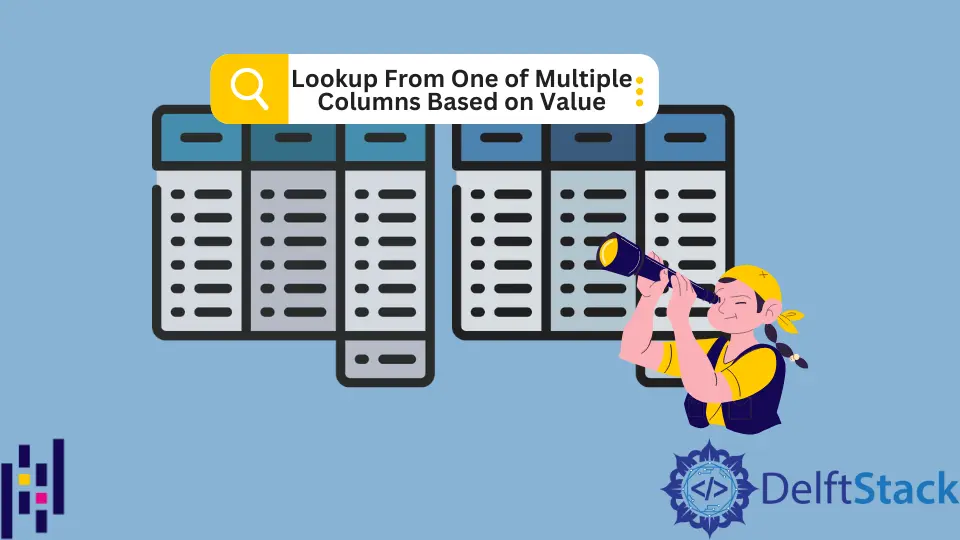
When working with data in Python, particularly with the Pandas library, you often find yourself needing to extract information from various columns based on specific criteria. This can be particularly useful when you have multiple columns that may contain the same type of information but are structured differently.
In this tutorial, we will explore how to perform lookups from multiple columns based on a specific value in Pandas. We will cover different methods, including using the apply
function, boolean indexing, and the melt
method. By the end of this article, you’ll be equipped with the skills to efficiently retrieve data from your DataFrame based on your requirements.
Method 1: Using the apply
Function
The apply
function in Pandas is a powerful tool that allows you to apply a function along the axis of a DataFrame. This method is particularly useful when you want to perform a lookup based on a condition that spans multiple columns.
Here’s how you can use the apply
function to achieve this:
import pandas as pd
data = {
'A': [1, 2, 3],
'B': [4, 5, 6],
'C': [7, 8, 9],
}
df = pd.DataFrame(data)
def lookup_value(row):
if row['A'] == 2:
return row['B']
elif row['A'] == 3:
return row['C']
return None
df['Result'] = df.apply(lookup_value, axis=1)
print(df)
Output:
A B C Result
0 1 4 7 None
1 2 5 8 5.0
2 3 6 9 9.0
In this example, we define a DataFrame df
with three columns: A, B, and C. The lookup_value
function checks the value in column A and returns the corresponding value from either column B or C. The apply
function is then used to apply this logic across each row of the DataFrame. The results are stored in a new column named Result
.
This method is particularly useful when you have complex conditions to check across multiple columns. However, it may not be the most efficient for very large DataFrames due to the overhead of applying a function row-wise.
Method 2: Boolean Indexing
Boolean indexing is another effective way to filter and retrieve data from a DataFrame based on conditions. This method is particularly useful when you want to look up values based on direct comparisons across multiple columns.
Here’s how you can implement boolean indexing for lookups:
import pandas as pd
data = {
'A': [1, 2, 3],
'B': [4, 5, 6],
'C': [7, 8, 9],
}
df = pd.DataFrame(data)
result = df[(df['A'] == 2) | (df['A'] == 3)]
print(result[['B', 'C']])
Output:
B C
1 5 8
2 6 9
In this example, we create a DataFrame df
with columns A, B, and C. We then use boolean indexing to filter the DataFrame based on the condition that column A is either 2 or 3. The resulting DataFrame contains only the rows that meet this condition, and we display only columns B and C. This method is efficient and straightforward, especially when dealing with larger datasets.
Boolean indexing can be combined with other operations, such as aggregations or further transformations, making it a versatile option for data manipulation in Pandas.
Method 3: Using the melt
Method
The melt
method in Pandas is a powerful way to reshape your DataFrame, and it can be particularly useful when you want to perform lookups across multiple columns. By melting the DataFrame, you can transform it from a wide format to a long format, which makes it easier to filter and retrieve data based on specific values.
Let’s see how to use the melt
method for lookups:
import pandas as pd
data = {
'ID': [1, 2, 3],
'A': [4, 5, 6],
'B': [7, 8, 9],
}
df = pd.DataFrame(data)
melted_df = df.melt(id_vars=['ID'], value_vars=['A', 'B'], var_name='Variable', value_name='Value')
result = melted_df[melted_df['Value'] > 5]
print(result)
Output:
ID Variable Value
2 3 A 6
3 2 B 8
4 3 B 9
In this example, we start with a DataFrame df
that has columns ID, A, and B. We then use the melt
method to reshape the DataFrame into a long format, where each value in columns A and B is paired with its corresponding ID. After melting, we filter the DataFrame to find values greater than 5. The result shows the ID, variable (which column the value came from), and the value itself.
The melt
method is particularly useful when you have a dataset where you want to analyze or visualize data across multiple columns, as it simplifies the structure and allows for easier filtering.
Conclusion
In this tutorial, we explored various methods to perform lookups from one of multiple columns based on a value in Pandas. We covered the use of the apply
function, boolean indexing, and the melt
method. Each method has its own strengths and can be chosen based on the specific requirements of your data analysis tasks. Whether you’re working with small or large datasets, these techniques will help you efficiently retrieve and manipulate data in your DataFrames. Mastering these methods will enhance your data manipulation skills and make your analyses more effective.
FAQ
- what is Pandas?
Pandas is a popular data manipulation and analysis library for Python, providing data structures like DataFrames and Series for handling structured data.
-
how do I install Pandas?
You can install Pandas using pip by running the commandpip install pandas
in your terminal or command prompt. -
can I use these methods on large datasets?
Yes, but be mindful of performance. Methods like boolean indexing are generally more efficient for larger datasets compared to row-wise operations likeapply
. -
what is the difference between wide and long format in data?
Wide format has multiple columns for different variables, while long format consolidates these variables into key-value pairs, making it easier to analyze. -
can I apply these methods to other data types?
Yes, these methods can be applied to any data types in a DataFrame, as long as the operations are compatible with the data type.