How to Calculate Exponential Moving Average Values in Pandas
-
Method 1: Using the
ewm()
Function - Method 2: Customizing the Span
- Method 3: Visualizing the EMA
- Conclusion
- FAQ
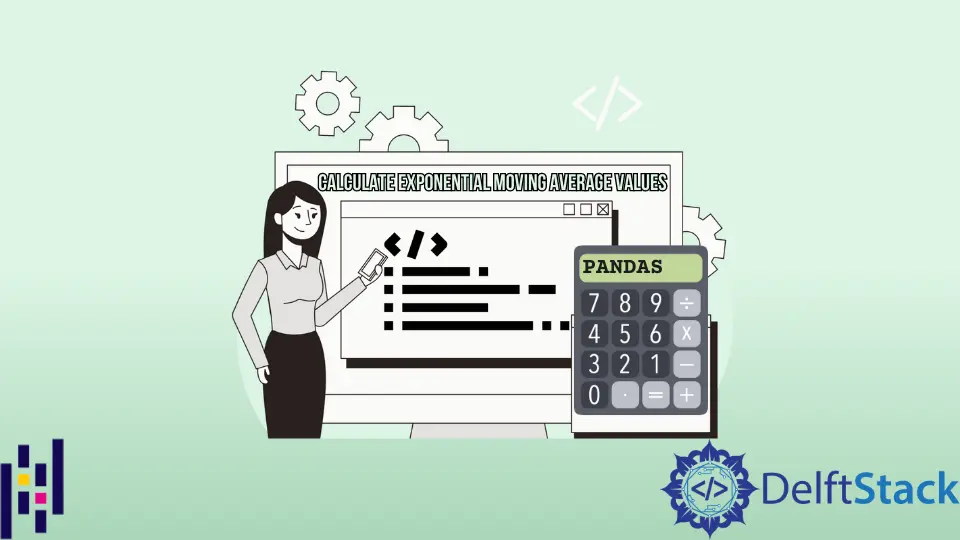
Calculating the Exponential Moving Average (EMA) is a crucial technique in data analysis, particularly in financial markets. It provides a smoothed average of data points over a specific time period, giving more weight to recent observations. This makes EMA particularly useful for identifying trends and making predictions.
In this tutorial, we will walk you through the steps to calculate EMA values in Pandas, a powerful data manipulation library in Python. Whether you’re analyzing stock prices or any time series data, understanding how to compute EMA will enhance your analytical skills. Let’s dive into the methods you can use to find these values efficiently.
Method 1: Using the ewm()
Function
One of the simplest ways to calculate the Exponential Moving Average in Pandas is by using the ewm()
function. This function allows you to specify the span, which determines the weighting of the observations. The EMA is then calculated based on this span.
Here’s how you can implement it:
import pandas as pd
data = {'Price': [100, 102, 101, 105, 107, 110, 108]}
df = pd.DataFrame(data)
df['EMA'] = df['Price'].ewm(span=3, adjust=False).mean()
print(df)
Output:
Price EMA
0 100 100.0
1 102 100.7
2 101 100.9
3 105 102.3
4 107 104.0
5 110 106.3
6 108 107.1
In this example, we created a DataFrame with stock prices and calculated the EMA with a span of 3. The ewm()
function is called on the ‘Price’ column, and the mean()
method computes the EMA values. The adjust=False
parameter ensures that the EMA is calculated in a straightforward way, without adjusting for the number of periods. As a result, the EMA values reflect the most recent prices more heavily, making them responsive to price changes.
Method 2: Customizing the Span
While the previous method used a fixed span, you might want to customize the span based on your specific analysis needs. The span determines how much weight is given to recent data points versus older ones. A shorter span will react more quickly to price changes, while a longer span will smooth out fluctuations.
Here’s how you can modify the span in your EMA calculation:
import pandas as pd
data = {'Price': [100, 102, 101, 105, 107, 110, 108]}
df = pd.DataFrame(data)
# Custom span
span = 5
df['EMA_Custom'] = df['Price'].ewm(span=span, adjust=False).mean()
print(df)
Output:
Price EMA_Custom
0 100 100.0
1 102 100.4
2 101 100.6
3 105 101.6
4 107 102.8
5 110 104.4
6 108 105.2
In this code snippet, we set a custom span of 5. The ewm()
function works the same way, but now the EMA values will incorporate a larger number of previous observations, resulting in a smoother line. This can be particularly useful when analyzing data that has a lot of noise or volatility. By adjusting the span, you can tailor the EMA calculation to fit your analytical requirements, helping you make more informed decisions.
Method 3: Visualizing the EMA
Visual representation can be a powerful tool in data analysis. Once you have calculated the EMA, plotting it alongside the original data can provide valuable insights into trends and price movements. For this, you can use the matplotlib
library to create a visual representation.
Here’s how you can visualize the EMA:
import pandas as pd
import matplotlib.pyplot as plt
data = {'Price': [100, 102, 101, 105, 107, 110, 108]}
df = pd.DataFrame(data)
df['EMA'] = df['Price'].ewm(span=3, adjust=False).mean()
plt.figure(figsize=(10, 5))
plt.plot(df['Price'], label='Price', marker='o')
plt.plot(df['EMA'], label='EMA', marker='x')
plt.title('Price and Exponential Moving Average')
plt.xlabel('Time')
plt.ylabel('Price')
plt.legend()
plt.show()
In this example, we first calculate the EMA as before. Then, we use matplotlib
to create a line plot that shows both the original price data and the EMA. The marker
parameter adds distinct markers to the data points, making it easier to visualize changes over time. This visualization can help you quickly identify trends, making it easier to spot potential buy or sell signals based on EMA behavior.
Conclusion
Calculating the Exponential Moving Average in Pandas is a straightforward process that can significantly enhance your data analysis capabilities. By using the ewm()
function, customizing the span, and visualizing the results, you can gain deeper insights into time series data. Whether you’re tracking stock prices or analyzing any other dataset, mastering EMA calculations will serve you well in your analytical journey. So, go ahead and apply these techniques in your projects, and watch your data analysis skills soar!
FAQ
-
What is the Exponential Moving Average?
The Exponential Moving Average (EMA) is a type of moving average that gives more weight to recent data points, making it more responsive to new information. -
How does the span affect the EMA?
The span determines how many previous observations are considered in the EMA calculation. A shorter span reacts more quickly to price changes, while a longer span smooths out fluctuations. -
Can I calculate EMA for multiple columns in a DataFrame?
Yes, you can calculate EMA for multiple columns by applying theewm()
function to each column separately. -
What library do I need to calculate EMA in Python?
You need the Pandas library to calculate EMA in Python. Additionally, for visualization, you may want to use Matplotlib. -
Is EMA better than Simple Moving Average (SMA)?
It depends on your analysis needs. EMA reacts more quickly to price changes, while SMA provides a smoother average over a set period.