How to Convert Pandas DataFrame to Series
-
Convert First Column of Pandas
DataFrame
toSeries
-
Convert Specific or Last Column of Pandas
DataFrame
toSeries
-
Convert Multiple Columns of Pandas
DataFrame
toSeries
-
Convert Single Row of Pandas
DataFrame
toSeries
- Conclusion
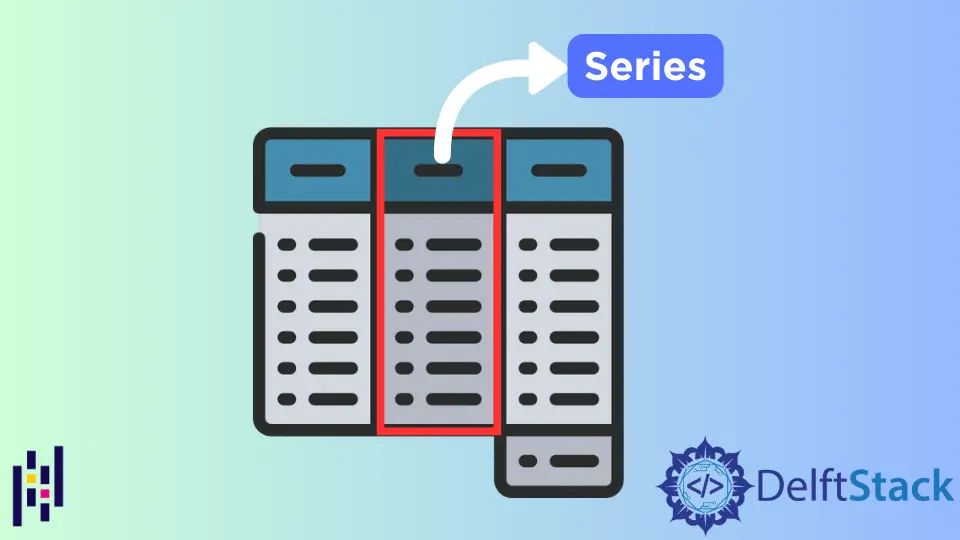
Pandas DataFrame
is a two-dimensional structure in which data is aligned into several columns and rows. In most cases, we need to convert the Pandas dataframe to series.
Series contains data in the form of a list with an index. In other words, a dataframe is a collection of series.
To analyze data, we can convert the columns or rows of the Pandas dataframe to series.
Using the Pandas series
, we can return an object type in a list with a proper index that starts from 0 to n value. We can convert the Pandas dataframes into a series using the squeeze
and index location df.iloc[:,0]
method.
This article will show you how to convert the Pandas DataFrame to Series. We will implement various scenarios like converting columns or rows of the Pandas dataframe to a series.
Convert First Column of Pandas DataFrame
to Series
We can convert a single or first column of the Pandas dataframe to a series. For example, we have taken students’ data in a dictionary and then created a DataFrame
.
Example Code:
import pandas as pd
# Create dictionary
students_record = {
"Student Names": ["Samreena", "Raees", "Asif", "Affan", "Idrees", "Mirha"],
"Physics": [87, 54, 68, 92, 50, 90],
"Chemistry": [78, 58, 85, 82, 76, 71],
"Maths": [90, 88, 50, 89, 77, 80],
}
# Converting dictionary to dataframe
dataframe = pd.DataFrame(data=students_record)
# Display DataFrame
print(dataframe)
Output:
In the following source code, we want to convert the first column of the Pandas dataframe to a series. Using the df.iloc[:,0]
, we can convert the first DataFrame
column to a series.
Example Code:
import pandas as pd
# Create dictionary
students_record = {
"Student Names": ["Samreena", "Raees", "Asif", "Affan", "Idrees", "Mirha"],
"Physics": [87, 54, 68, 92, 50, 90],
"Chemistry": [78, 58, 85, 82, 76, 71],
"Maths": [90, 88, 50, 89, 77, 80],
}
# Converting dictionary to dataframe
dataframe = pd.DataFrame(data=students_record)
# Display DataFrame
dataframe
# Converting first column to Series
series = dataframe.iloc[:, 0]
print("\nFirst column as a Series:\n")
print(series)
# display type
print(type(series))
Output:
In the following example, we have converted the first column Students Names
of Pandas dataframe to series using the dataframe['Student Names'].squeeze()
method. It will also show a similar output to the above.
Example Code:
import pandas as pd
# Create dictionary
students_record = {
"Student Names": ["Samreena", "Raees", "Asif", "Affan", "Idrees", "Mirha"],
"Physics": [87, 54, 68, 92, 50, 90],
"Chemistry": [78, 58, 85, 82, 76, 71],
"Maths": [90, 88, 50, 89, 77, 80],
}
# Converting dictionary to dataframe
dataframe = pd.DataFrame(data=students_record)
# Display DataFrame
dataframe
# Converting first column to Series
series = dataframe["Student Names"].squeeze()
print("\nFirst column as a Series:\n")
print(series)
# display type
print(type(series))
Output:
Convert Specific or Last Column of Pandas DataFrame
to Series
To convert the last or specific column of the Pandas dataframe to series, use the integer-location-based index in the df.iloc[:,0]
.
For example, we want to convert the third or last column of the given data from Pandas dataframe to series.
In this case, the following code example will help us.
import pandas as pd
# Create dictionary
students_record = {
"Student Names": ["Samreena", "Raees", "Asif", "Affan", "Idrees", "Mirha"],
"Physics": [87, 54, 68, 92, 50, 90],
"Chemistry": [78, 58, 85, 82, 76, 71],
"Maths": [90, 88, 50, 89, 77, 80],
}
# Converting dictionary to dataframe
dataframe = pd.DataFrame(data=students_record)
# Display DataFrame
dataframe
# Converting specific column to Series
series = dataframe.iloc[:, 3]
print("\nSpecific column as a Series:\n")
print(series)
# display type
print(type(series))
Output:
We can also convert the specific column of Pandas dataframe to series using squeeze method by passing the column name with DataFrame
like dataframe['Maths'].squeeze()
.
Example Code:
import pandas as pd
# Create dictionary
students_record = {
"Student Names": ["Samreena", "Raees", "Asif", "Affan", "Idrees", "Mirha"],
"Physics": [87, 54, 68, 92, 50, 90],
"Chemistry": [78, 58, 85, 82, 76, 71],
"Maths": [90, 88, 50, 89, 77, 80],
}
# Converting dictionary to dataframe
dataframe = pd.DataFrame(data=students_record)
# Display DataFrame
dataframe
# Converting Specific column to Series
series = dataframe["Maths"].squeeze()
print("\nSpecific column as a Series:\n")
print(series)
# display type
print(type(series))
Output:
Convert Multiple Columns of Pandas DataFrame
to Series
We can convert the multiple columns of the Pandas DataFrame to series.
First, we have created a DataFrame
from the dictionary. Further, the DataFrame
columns are converted to a series
.
Example Code:
import pandas as pd
# Create dictionary
students_record = {
"Student Names": ["Samreena", "Raees", "Asif", "Affan", "Idrees", "Mirha"],
"Physics": [87, 54, 68, 92, 50, 90],
"Chemistry": [78, 58, 85, 82, 76, 71],
"Maths": [90, 88, 50, 89, 77, 80],
}
# Converting dictionary to DataFrame
dataframe = pd.DataFrame(data=students_record)
# Display DataFrame
dataframe
# Converting multiple columns of DataFrame to Series
series1 = dataframe.iloc[:, 1]
series2 = dataframe.iloc[:, 2]
series3 = dataframe.iloc[:, 3]
print("\nConvert Multiple columns of dataframe to Series:\n")
print(series1, "\n")
print(series2, "\n")
print(series3, "\n")
# Display type
print(type(series1))
print(type(series2))
print(type(series3))
Output:
We have converted three Pandas dataframe columns to series in the above output.
Convert Single Row of Pandas DataFrame
to Series
As shown below, we can also convert the single Pandas dataframe row to series using the squeeze()
method.
import pandas as pd
# Create dictionary
students_record = {
"Student Names": ["Samreena", "Raees", "Asif", "Affan", "Idrees", "Mirha"],
"Physics": [87, 54, 68, 92, 50, 90],
"Chemistry": [78, 58, 85, 82, 76, 71],
"Maths": [90, 88, 50, 89, 77, 80],
}
# Converting dictionary to DataFrame
dataframe = pd.DataFrame(data=students_record)
# Display DataFrame
dataframe
# Converting DataFrame row to Series
series = dataframe.iloc[3].reset_index(drop=True).squeeze()
print(series)
print(type(series))
Output:
In the above output, we can see the series with the new index that contains integers.
Conclusion
We have shown in this tutorial how to convert the columns or rows of the Pandas DataFrame
to series
. Using the different scenarios, we demonstrated each case with the help of source code.
We hope you find all the examples useful and increase your understanding of Pandas DataFrame
and series
. You can contact us in case of any feedback.