How to Implement Pandas Conditional Formatting
- Pandas Conditional Formatting
- Use Pandas Conditional Formatting
-
Conditional Formatting Using the
style
Attribute - Conclusion
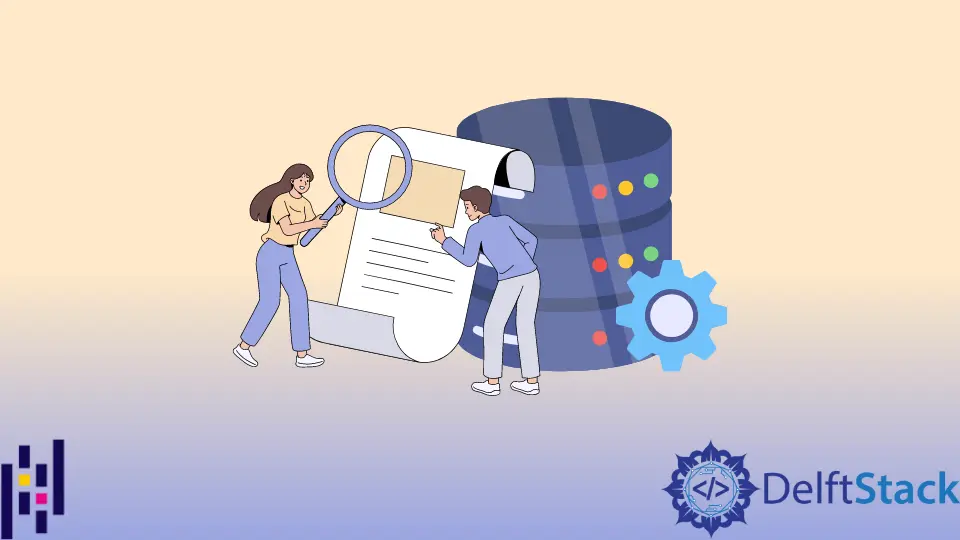
You might want to highlight all cells in a column that contain a specific value or all cells in a row that exceed a certain threshold. Conditional formatting can also quickly identify errors or outliers in your data.
In this article, we’ll discuss Pandas’ conditional formatting.
Pandas Conditional Formatting
Pandas conditional formatting is a powerful tool that allows you to format your dataframe
columns based on conditions. For example, you could utilize conditional formatting to highlight all cells in a column greater than a certain value, or you could use it to format cells based on whether they contain a certain text string.
There are a few different ways to format your dataframe
columns using pandas conditional formatting. One way is to use the style
function; this function takes a dictionary of conditions and formats and applies those formats to the dataframe
.
Another way to use pandas conditional formatting is the apply
function; this function allows you to apply a function to all cells in a column based on a condition.
Use Pandas Conditional Formatting
We can use the round()
function to conditionally format cells containing numeric values.
df.round(2)
This will round all numeric values in the data frame to two decimal places. We can also use the format()
function to conditionally format cells containing text values.
df.format("%s")
This will format all text values in the data frame as strings. We can also utilize the apply()
function to conditionally format cells based on custom criteria.
df.apply(lambda x: x.str.upper() if x.name == "column1" else x)
This will format all values as uppercase text in the column1
column.
Conditional Formatting Using the style
Attribute
There are a few different ways to format pandas cells based on conditions. The most common way is using the style
attribute.
You can accomplish this by setting the cell’s style
attribute to a dictionary of CSS
properties. The dictionary’s keys should be the names of the CSS
properties, and the values should be the values you want to set those properties to.
import pandas as pd
df = pd.DataFrame([[2, 3, 1], [3, 2, 2], [2, 4, 4]], columns=list("ABC"))
df.style.apply(
lambda x: ["background: red" if v > x.iloc[0] else "" for v in x], axis=1
)
Output:
Conditional Formatting Using the applymap()
Method
You can also utilize the applymap()
method to format all the cells in a data frame. This method takes a function as an argument and applies that function to every cell in the data frame.
import numpy as np
import pandas as pd
df = pd.DataFrame(np.random.rand(4, 3))
df.style.applymap(lambda x: "background-color : yellow" if x > df.iloc[0, 0] else "")
Output:
Whichever way you format your cells, make sure you do it in a way that makes your data easy to read and understand.
Conclusion
In conclusion, it is possible to format a Python pandas cell based on its value conditionally. You can use the built-in style
function, which takes a dictionary of CSS
style properties and values.
The most important property to set is the background-color
property, which can be set to a hex code or a named color. Other properties that can be set include color
(for the text color), font-weight
(for the boldness of the text), and text-align
(for the horizontal alignment of the text).
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn