How to Apply Function to Every Row in Pandas DataFrame
-
Basic Syntax of Pandas
apply()
Function -
Apply
lambda
Function to Each Row in PandasDataFrame
-
Apply a
NumPy
Function to Each Row of PandasDataFrame
-
Apply a User-Defined Function to Each Row of Pandas
DataFrame
With Arguments -
Apply a User-Defined Function to Each Row of Pandas
DataFrame
Without Arguments
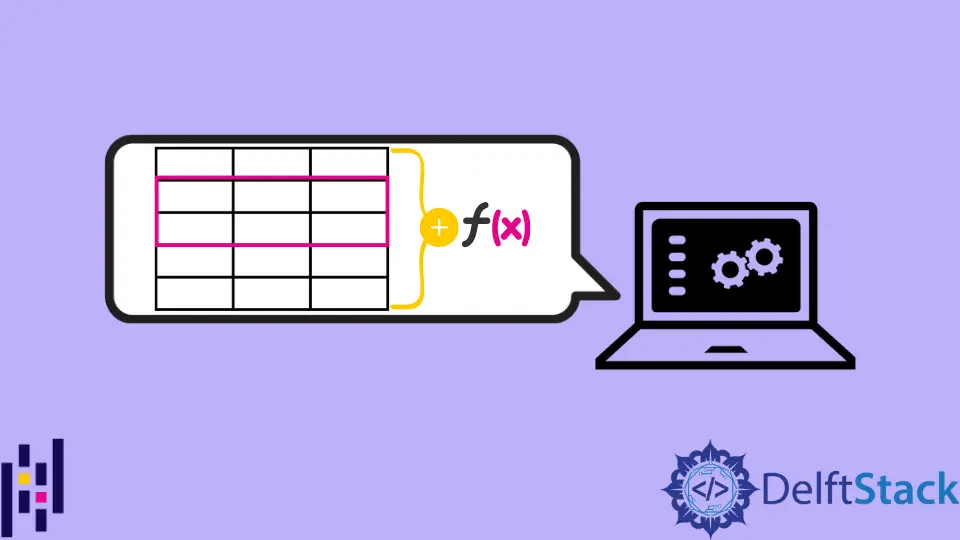
Pandas is a python library, which provides a huge list of classes and functions for performing data analysis and manipulation tasks in an easier way. We manipulate data in the pandas DataFrame
in the form of rows and columns. Therefore, most of the time, we need to apply appropriate functions to each row or a column to obtain the desired results.
This article will explore how to use pandas to apply function to every row in Pandas DataFrame
. Moreover, we will demonstrate how to apply various functions such as the lambda
function, a user-defined function, and NumPy
function to each row in a pandas DataFrame
.
Basic Syntax of Pandas apply()
Function
The following basic syntax is used for applying pandas apply()
function:
DataFrame.apply(function, axis, args=())
See In the above syntax, the function is to be applied to each row. The axis
is the argument along which the function is applied in the DataFrame
. By default, the axis
value is 0
. The value of axis=1
, if function applies to every row. The args
represents the tuple or list of arguments passed to the function.
Using the pandas apply()
function, we can easily apply different functions to every row in the DataFrame
. The following listed ways help us to achieve this goal:
Apply lambda
Function to Each Row in Pandas DataFrame
For applying the lambda
function to every row in the DataFrame
, we used the lambda
function as the first argument in the DataFrame
, and axis=1
as the second argument, in the dataframe.apply()
function.
To see how to apply the lambda
function to every row in the DataFrame
, try the following example:
Example Codes:
import pandas as pd
import numpy as np
from IPython.display import display
# List of Tuples data
data = [
(1, 34, 23),
(11, 31, 11),
(22, 16, 21),
(33, 32, 22),
(44, 33, 27),
(55, 35, 11),
]
# Create a DataFrame object
dataframe = pd.DataFrame(data, columns=list("ABC"))
print("Original Dataframe before applying lambda function: ", sep="\n")
display(dataframe)
# Apply a lambda function to each row by adding 10
new_dataframe = dataframe.apply(lambda x: x + 10, axis=1)
print("Modified New Dataframe by applying lambda function on each row:")
display(new_dataframe)
Output:
Original Dataframe before applying lambda function:
A B C
0 1 34 23
1 11 31 11
2 22 16 21
3 33 32 22
4 44 33 27
5 55 35 11
Modified Dataframe by applying lambda function on each row:
A B C
0 11 44 33
1 21 41 21
2 32 26 31
3 43 42 32
4 54 43 37
5 65 45 21
Apply a NumPy
Function to Each Row of Pandas DataFrame
We can also use the NumPy
function passed as an argument to dataframe.apply()
. In the following example, we apply the NumPy
function to every row and calculate each value’s square root.
Example Codes:
import pandas as pd
import numpy as np
from IPython.display import display
def main():
# List of Tuples
data = [
(2, 3, 4),
(3, 5, 10),
(44, 16, 2),
(55, 32, 12),
(60, 33, 27),
(77, 35, 11),
]
# Create a DataFrame object
dataframe = pd.DataFrame(data, columns=list("ABC"))
print("Original Dataframe", sep="\n")
display(dataframe)
# Apply a numpy function to every row by taking square root of each value
new_dataframe = dataframe.apply(np.sqrt, axis=1)
print("Modified Dataframe by applying numpy function on each row:", sep="\n")
display(new_dataframe)
if __name__ == "__main__":
main()
Output:
Original Dataframe
A B C
0 2 3 4
1 3 5 10
2 44 16 2
3 55 32 12
4 60 33 27
5 77 35 11
Modified Dataframe by applying numpy function on each row:
A B C
0 1.414214 1.732051 2.000000
1 1.732051 2.236068 3.162278
2 6.633250 4.000000 1.414214
3 7.416198 5.656854 3.464102
4 7.745967 5.744563 5.196152
5 8.774964 5.916080 3.316625
Apply a User-Defined Function to Each Row of Pandas DataFrame
With Arguments
We can also pass the user defined function as a parameter in the dataframe.apply
with some argument. In the following example, we passed a user-defined function with the argument args=[2]
. Each row value series is multiplied by 2.
See the following example:
Example Code:
import pandas as pd
import numpy as np
from IPython.display import display
def multiplyData(x, y):
return x * y
def main():
# List of Tuples
data = [
(2, 3, 4),
(3, 5, 10),
(44, 16, 2),
(55, 32, 12),
(60, 33, 27),
(77, 35, 11),
]
# Create a DataFrame object
dataframe = pd.DataFrame(data, columns=list("ABC"))
print("Original Dataframe", sep="\n")
display(dataframe)
# Apply a user defined function with arguments to each row of Pandas dataframe
new_dataframe = dataframe.apply(multiplyData, axis=1, args=[2])
print(
"Modified Dataframe by applying user defined function on each row of pandas dataframe:",
sep="\n",
)
display(new_dataframe)
if __name__ == "__main__":
main()
Output:
Original Dataframe
A B C
0 2 3 4
1 3 5 10
2 44 16 2
3 55 32 12
4 60 33 27
5 77 35 11
Modified Dataframe by applying user defined function on each row of pandas dataframe:
A B C
0 4 6 8
1 6 10 20
2 88 32 4
3 110 64 24
4 120 66 54
5 154 70 22
Apply a User-Defined Function to Each Row of Pandas DataFrame
Without Arguments
We can also apply a user-defined function to every row without any arguments. See the following example:
Example Codes:
import pandas as pd
import numpy as np
from IPython.display import display
def userDefined(x):
return x * 4
def main():
# List of Tuples
data = [
(2, 3, 4),
(3, 5, 10),
(44, 16, 2),
(55, 32, 12),
(60, 33, 27),
(77, 35, 11),
]
# Create a DataFrame object
dataframe = pd.DataFrame(data, columns=list("ABC"))
print("Original Dataframe", sep="\n")
display(dataframe)
# Apply a user defined function without arguments to each row of Pandas dataframe
new_dataframe = dataframe.apply(userDefined, axis=1)
print(
"Modified Dataframe by applying user defined function on each row of pandas dataframe:",
sep="\n",
)
display(new_dataframe)
if __name__ == "__main__":
main()
Output:
Original Dataframe
A B C
0 2 3 4
1 3 5 10
2 44 16 2
3 55 32 12
4 60 33 27
5 77 35 11
Modified Dataframe by applying user defined function on each row of pandas dataframe:
A B C
0 8 12 16
1 12 20 40
2 176 64 8
3 220 128 48
4 240 132 108
5 308 140 44