How to Find the Product of Columns in a Pandas DataFrame
-
Method 1: Using the
prod()
Function -
Method 2: Using the
apply()
Method - Method 3: Using NumPy for Performance
- Conclusion
- FAQ
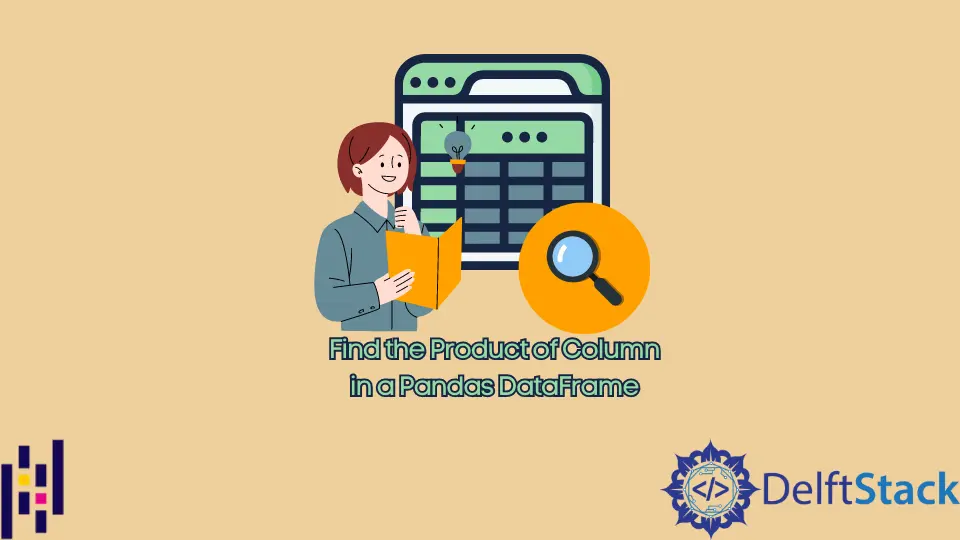
Finding the product of columns in a Pandas DataFrame is a common task for data manipulation and analysis in Python. Whether you’re working with financial data, scientific measurements, or any dataset requiring mathematical computations, knowing how to efficiently compute the product of multiple columns can save you time and enhance your data analysis capabilities.
In this tutorial, we will explore various methods to achieve this using Pandas, a powerful library for data manipulation in Python. By the end of this article, you’ll be equipped with the knowledge to calculate column products and apply these techniques to your own datasets. Let’s dive in!
Method 1: Using the prod()
Function
One of the simplest ways to find the product of columns in a Pandas DataFrame is by using the built-in prod()
function. This method is straightforward and efficient, especially when you want to compute the product across a specific axis.
import pandas as pd
data = {
'A': [1, 2, 3],
'B': [4, 5, 6],
'C': [7, 8, 9]
}
df = pd.DataFrame(data)
product_columns = df.prod(axis=1)
print(product_columns)
Output:
0 28
1 80
2 162
dtype: int64
In this code, we first import the Pandas library and create a DataFrame with three columns: A, B, and C. The prod()
function is then called on the DataFrame, specifying axis=1
to compute the product across rows. The output shows the product of the values in each row, which can be particularly useful for aggregating data points or performing further calculations. This method is not only easy to implement but also leverages Pandas’ optimized performance for handling large datasets.
Method 2: Using the apply()
Method
Another effective way to compute the product of columns is by using the apply()
method. This method allows for more customized operations, making it versatile for various scenarios.
import pandas as pd
data = {
'A': [1, 2, 3],
'B': [4, 5, 6],
'C': [7, 8, 9]
}
df = pd.DataFrame(data)
product_columns = df.apply(lambda x: x.prod(), axis=1)
print(product_columns)
Output:
0 28
1 80
2 162
dtype: int64
In this example, we again create a DataFrame similar to the previous method. However, this time we use the apply()
function along with a lambda function that computes the product of each row. Specifying axis=1
ensures that the function operates on rows rather than columns. The output remains the same as before, illustrating that both methods yield equivalent results. The apply()
method is particularly useful when you need to perform more complex calculations or when you want to combine multiple operations in a single pass.
Method 3: Using NumPy for Performance
For those who prioritize performance, especially with larger datasets, using NumPy can be a great alternative. Since Pandas is built on top of NumPy, leveraging NumPy functions can significantly enhance execution speed.
import pandas as pd
import numpy as np
data = {
'A': [1, 2, 3],
'B': [4, 5, 6],
'C': [7, 8, 9]
}
df = pd.DataFrame(data)
product_columns = np.prod(df.values, axis=1)
print(product_columns)
Output:
[ 28 80 162]
In this code snippet, we import both Pandas and NumPy. After creating the DataFrame, we convert it to a NumPy array using df.values
. The np.prod()
function is then called to compute the product across the specified axis. The output is a NumPy array containing the product of each row. This method is particularly advantageous when dealing with large datasets, as NumPy is optimized for numerical operations, leading to faster computations compared to pure Pandas methods.
Conclusion
In this article, we explored three effective methods for finding the product of columns in a Pandas DataFrame: using the prod()
function, the apply()
method, and NumPy. Each method has its own advantages, depending on your specific needs and the size of your dataset. Whether you’re performing simple calculations or working with complex datasets, these techniques will enhance your data manipulation skills in Python. By mastering these methods, you will be better equipped to handle various data analysis tasks with ease.
FAQ
-
How can I calculate the product of specific columns only?
You can select the specific columns you want to multiply and then apply theprod()
function on that subset of the DataFrame. -
Is there a way to calculate the cumulative product of a column?
Yes, you can use thecumprod()
function in Pandas to calculate the cumulative product of a column. -
Can I find the product of columns conditionally?
Yes, you can apply conditional filtering before using the product functions to compute products based on specific criteria. -
What happens if there are NaN values in my DataFrame?
By default, theprod()
function ignores NaN values, but you can use themin_count
parameter to control this behavior. -
How can I visualize the product of columns in a DataFrame?
You can use libraries like Matplotlib or Seaborn to create visualizations of the product results after calculating them.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn