DatetimeIndex.date in Pandas
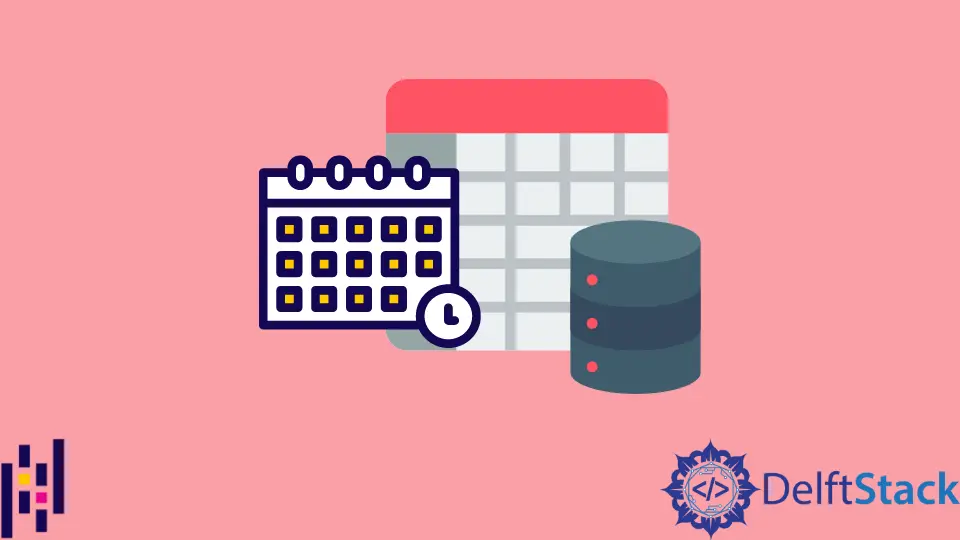
Pandas is a data manipulation library in Python. It is used to analyze data and see data trends.
It is the most widely used library in machine learning and data science. The Pandas library works on 2 main data structures, i.e., Series and DataFrame.
A variable of type Series is simply nothing but a data column. A variable of type DataFrame is a multidimensional table of rows and columns.
Use DatetimeIndex.date
in Pandas
DatetimeIndex
is a multidimensional array of type datetime64
, which can only be accessed but is not editable. Let’s see an example of how to access DatetimeIndex
data:
# Accessing Date from datetimeIndex
import pandas as pd
date = pd.date_range("2022-01-01", periods=5, freq="D")
series = pd.Series(range(10, len(date) + 10), index=date)
print(series)
Output:
2022-01-01 10
2022-01-02 11
2022-01-03 12
2022-01-04 13
2022-01-05 14
Freq: D, dtype: int64
The above code accesses dates ranging from 2022-05-20
to 2022-05-29
along with printing the dates using a Series
object.
To add a timestamp with the date, you can do this.
import pandas as pd
date = pd.DatetimeIndex(start="2022-05-20 2:00:00", periods=5, freq="D")
series = pd.Series(range(10, len(date) + 10), index=date)
print(series)
Output:
2022-05-20 02:00:00 10
2022-05-21 02:00:00 11
2022-05-22 02:00:00 12
2022-05-23 02:00:00 13
2022-05-24 02:00:00 14
Freq: D, dtype: int64
This example code prints a timestamp along with each date.
Now let’s find out the names of months along a date range using DatetimeIndex
:
import pandas as pd
date = pd.DatetimeIndex(start="2022-05-20", end="2023-05-20", periods=5)
series = pd.Series(date.month_name(), index=date)
print(series)
Output:
2022-05-20 00:00:00 May
2022-08-19 06:00:00 August
2022-11-18 12:00:00 November
2023-02-17 18:00:00 February
2023-05-20 00:00:00 May
dtype: object
The above code prints the names of months in the range of dates from 2022-05-20
to 2023-05-20
.
Attributes of the DatetimeIndex
Function
start
: This defines the starting range for the date.end
: This defines the ending range for the date.periods
: This defines the intervals for the date. In the above example,periods=5
means every new date is 5 days later than the previous date.freq
: This tells the frequency inDatetimeIndex
.
Example Code:
import pandas as pd
date = pd.DatetimeIndex(start="2022-07-14", periods=10, freq="BQ")
print(date)
Output:
DatetimeIndex(['2022-09-30', '2022-12-30', '2023-03-31', '2023-06-30',
'2023-09-29', '2023-12-29', '2024-03-29', '2024-06-28',
'2024-09-30', '2024-12-31'],
dtype='datetime64[ns]', freq='BQ-DEC')
print(date.freq)
Output:
<BusinessQuarterEnd: startingMonth=12>
Conclusion
Pandas is a huge and widely used library in Python and contains a lot of functions to help manage and analyze data. DatetimeIndex
is one of the important functions used to access date and time data according to the week, month, and year from a multidimensional array.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn