How to Create an Empty Pandas DataFrame and Fill It With Data
- Create an Empty Pandas DataFrame Without Using Column Names
- Create an Empty Pandas DataFrame With Column Names
- Create an Empty Pandas DataFrame With Column and Row Indices
- Fill Data in an Empty Pandas DataFrame by Appending Columns
- Fill Data in an Empty Pandas DataFrame by Appending Rows
-
Fill Data in an Empty Pandas DataFrame Using
for
Loop
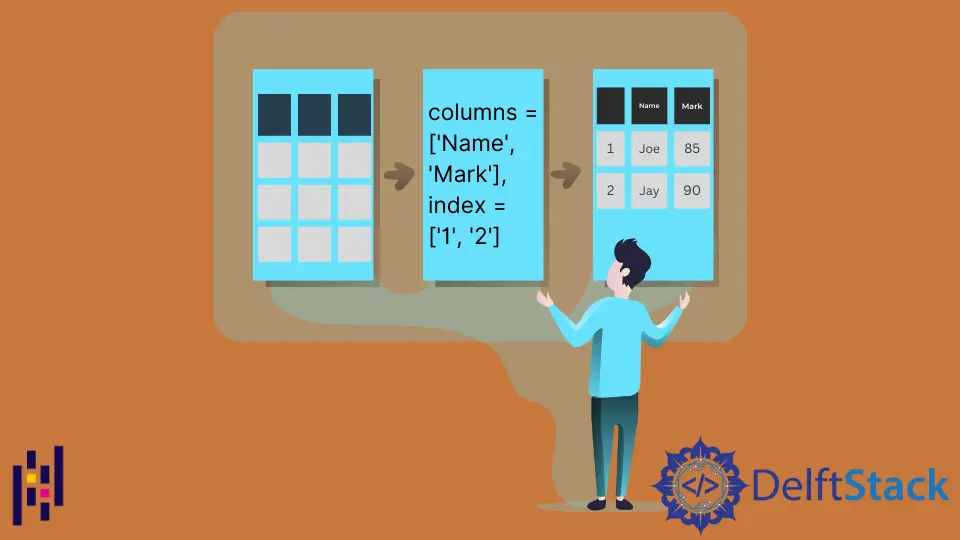
This tutorial discusses creating an empty Pandas DataFrame and filling it with data either by appending rows or columns.
Create an Empty Pandas DataFrame Without Using Column Names
We can create an empty Pandas Dataframe without defining column names and indices as an argument. In the following example, we created an empty Pandas DataFrame by calling the DataFrame
class constructor without passing any argument.
import pandas as pd
# create an Empty pandas DataFrame
dataframe = pd.DataFrame()
print(dataframe)
Output:
Empty DataFrame
Columns: []
Index: []
Create an Empty Pandas DataFrame With Column Names
An alternative method is also available to create an empty Pandas DataFrame. We can create an empty DataFrame by passing column names as arguments.
import pandas as pd
# create an Empty pandas DataFrame with column names
df = pd.DataFrame(columns=["Student Name", "Subjects", "Marks"])
print(df)
Output:
Empty DataFrame
Columns: [Student Names, Subjects, Marks]
Index: []
Create an Empty Pandas DataFrame With Column and Row Indices
If we don’t have data to fill the DataFrame, we can create an empty DataFrame with column names and row indices. Later, we can fill data inside this empty DataFrame.
import pandas as pd
# create an Empty pandas DataFrame with column names indices
df = pd.DataFrame(
columns=["Student Name", "Subjects", "Marks"], index=["a1", "a2", "a3"]
)
print(df)
Output:
Student Names Subjects Marks
a1 NaN NaN NaN
a2 NaN NaN NaN
a3 NaN NaN NaN
Fill Data in an Empty Pandas DataFrame by Appending Columns
After creating an empty DataFrame without columns and indices, we can fill the empty DataFrame by appending columns one by one.
We use the append()
method in the following code.
import pandas as pd
# create an Empty pandas DataFrame
df = pd.DataFrame()
print(df)
# append data in columns to an empty pandas DataFrame
df["Student Name"] = ["Samreena", "Asif", "Mirha", "Affan"]
df["Subjects"] = ["Computer Science", "Physics", "Maths", "Chemistry"]
df["Marks"] = [90, 75, 100, 78]
df
Output:
Fill Data in an Empty Pandas DataFrame by Appending Rows
First, create an empty DataFrame with column names and then append rows one by one.
The append()
method can also append rows.
import pandas as pd
# create an Empty pandas DataFrame with column names
df = pd.DataFrame(columns=["Student Name", "Subjects", "Marks"])
print(df)
df = df.append(
{"Student Name": "Samreena", "Subjects": "Computer Science", "Marks": 100},
ignore_index=True,
)
df = df.append(
{"Student Name": "Asif", "Subjects": "Maths", "Marks": 80}, ignore_index=True
)
df = df.append(
{"Student Name": "Mirha", "Subjects": "Physics", "Marks": 90}, ignore_index=True
)
df
Output:
When creating an empty DataFrame with column names and row indices, we can fill data in rows using the loc()
method.
import pandas as pd
# create an Empty pandas DataFrame with column names indices
df = pd.DataFrame(
columns=["Student Name", "Subjects", "Marks"], index=["a1", "a2", "a3"]
)
print(df)
df.loc["a1"] = ["Samreena", "Computer Science", 100]
df.loc["a2"] = ["Asif", "Maths", 90]
df.loc["a3"] = ["Mirha", "Chemistry", 60]
df
Output:
Fill Data in an Empty Pandas DataFrame Using for
Loop
When we have many files or data, it is difficult to fill data into the Pandas DataFrame one by one using the append()
method. In this case, we can use the for
loop to append the data iteratively.
In the following example, we have initialized data in a list and then used the append()
method inside the for
loop.
import pandas as pd
df = pd.DataFrame()
list = [
["Samreena", "Computer Science", 100],
["Asif", "Maths", 90],
["Mirha", "Chemistry", 60],
]
for student in list:
temp_df = pd.DataFrame([student], columns=["Student Name", "Subjects", "Marks"])
df = df.append(temp_df, ignore_index=True)
print(df)
Output: