Flask jsonify
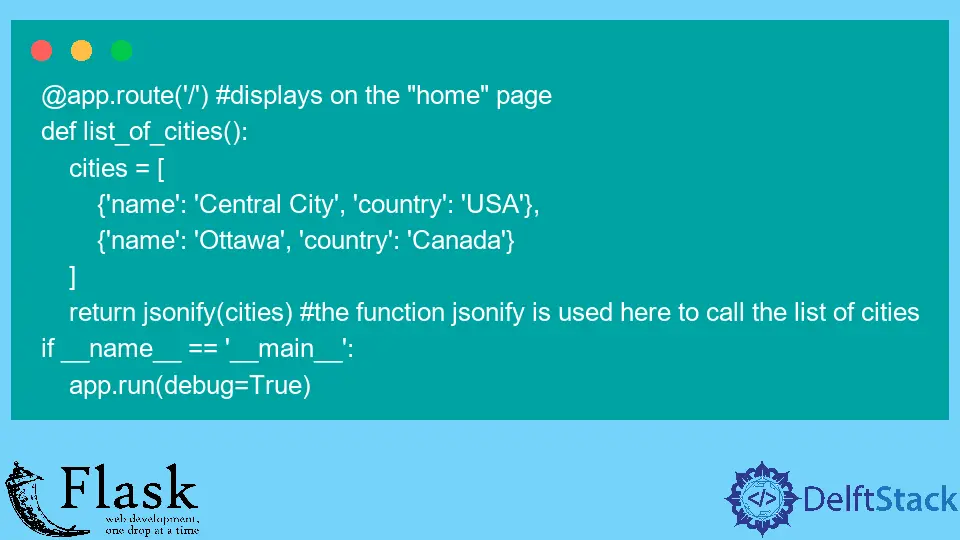
This guide will see what jsonify
is and dumps
it in the flask
. What is the use of flask.jsonify
and json.dump
, and what is the difference between them?
So without any further wait, let us dive in.
Use of flask.jsonify()
and json.dumps()
There seems to be an increasing number of people getting confused between flask.jsonify()
and json.dumps()
since there are only slight differences between these two functions.
The difference between the two in terms of how they work is not as difficult as it may seem initially.
To make it simple for us to understand these differences, let us answer the main question: when should we use json.dumps()
and flask.jsonify()
? But first, let’s see what is json.dumps()
.
the json.dumps()
Method
First, while reading or writing the JSON files, we have to use the json
package. This package includes a method named json.dumps()
to convert the data written in Python to a JSON file.
This method will convert every data written in Python and return it in strings, which will now be read as a JSON file.
An example of the json.dumps()
method is used to name the list of cities.
@app.route("/") # display on "home" page
def list_of_cities_dumps():
cities = [
{"name": "Central City", "country": "USA"},
{"name": "Ottawa", "country": "Canada"},
]
return json.dumps(
cities
) # json.dump() method is being used to call the list of cities
if __name__ == "__main__":
app.run(debug=True)
Output:
[{'name': 'Central City', 'country': 'USA'}, {'name': 'Ottawa', 'country': 'Canada'}]
As shown in the above example, the output of the list of cities is shown. Even though it is readable, imagine if the user had data on students of a school.
It would be hard to distinguish between the students if this method were to be used as there is no clear data formation.
So to overcome this flaw, the flask.jsonify()
function was introduced.
the flask.jsonify()
Function
In the flask.json
module, jsonify
is a function in Flask. To send data as an HTTP response when using the Flask framework, you will need to use the flask.jsonify()
function.
We would need to import flask
to use this function.
This function will then convert the data into a JSON file and then return a response object, which is used for the server to respond to the client request.
The converted JSON file will be added to the newly created response object. Setting the content-type header field to application/JSON
will also add the appropriate mimetype, commonly known as a media type.
When you send a file along with a media type or mimetype, it indicates the type of file that it is along with the message.
With the help of the same example above, let us further understand the working of flask.jsonify()
.
@app.route("/") # displays on the "home" page
def list_of_cities():
cities = [
{"name": "Central City", "country": "USA"},
{"name": "Ottawa", "country": "Canada"},
]
return jsonify(
cities
) # the function jsonify is used here to call the list of cities
if __name__ == "__main__":
app.run(debug=True)
Output:
[
{
"country": "USA",
"name": "Central City"
},
{
"country": "Canada",
"name": "Ottawa"
}
]
As shown in the output, the output of the list of cities is organized and easy to understand compared to when we used the method json.dumps()
.
The below code is the combination of both examples.
from flask import Flask, jsonify
import json
app = Flask(__name__)
@app.route("/")
def list_of_cities():
cities = [
{"name": "Central City", "country": "USA"},
{"name": "Ottawa", "country": "Canada"},
]
return jsonify(cities)
@app.route("/1")
def list_of_cities_dumps():
cities = [
{"name": "Central City", "country": "USA"},
{"name": "Ottawa", "country": "Canada"},
]
return json.dumps(cities)
if __name__ == "__main__":
app.run(debug=True)
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn