How to Wait for Each Command to Finish in PowerShell
-
Using the
Out
Commands to Wait for Each Command to Finish in PowerShell -
Using the
Start-Process
Cmdlet to Wait for Each Command to Finish in PowerShell - Using Background Jobs to Wait for Each Command to Finish in PowerShell
-
Using the
Wait-Process
Cmdlet to Wait for Each Command to Finish in PowerShell -
Using the
[Diagnostics.Process]
Class to Wait for Each Command to Finish in PowerShell
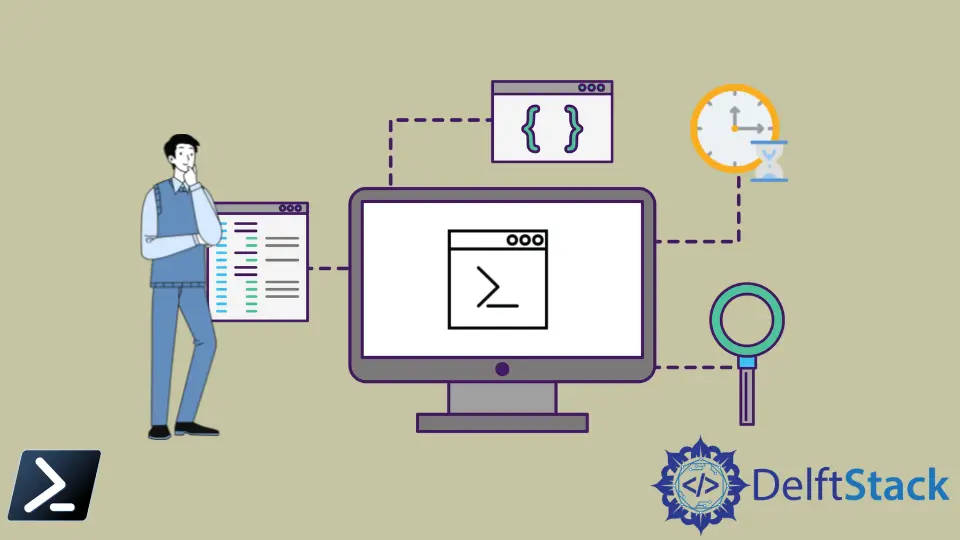
Usually, Windows PowerShell does wait for internal commands before starting the following command.
One exception to this rule is external Windows subsystem-based EXE. In addition, Windows PowerShell does not wait for the commands to finish when running executable programs.
This article will show you ways to force the Windows PowerShell environment to wait for the commands with executable files to finish before moving to the following command.
Using the Out
Commands to Wait for Each Command to Finish in PowerShell
As mentioned, running an executable file will not wait for the command to finish and will directly go to the following command. We may need to avoid this, especially if we create a script that should run applications sequentially.
One nifty trick is to use one of the Out
commands like Out-Null.
When running an application, piping the Out-Null
cmdlet will trick Windows PowerShell into running an underlying cmdlet on top of the running application.
Remember that the cmdlet Out-Null
does not display anything in the console and thus making it seem like a standard running application. The only difference is that it waits for the whole command line to execute.
Example Code:
Notepad.exe | Out-Null
Other examples of Out
commands that we can use are the following:
Out-Default
- Send the output to the default format environment and the default output cmdlet.Out-File
- Sends output to a file.Out-GridView
- Sends output to an interactive table in a separate window.Out-Host
- Sends the output to the command line.Out-Printer
- Sends output to a printer.Out-String
- Sends objects to the host as a series of strings.
Using the Start-Process
Cmdlet to Wait for Each Command to Finish in PowerShell
Another method of making Windows PowerShell wait for the command line to finish is the Start-Process
cmdlet.
For example, we can use the Start-Process
cmdlet’s -Wait
parameter, and the Windows PowerShell will wait for the process to finish before executing the following command line.
Start-Process notepad.exe -NoNewWindow -Wait
-NoNewWindow
parameter in the above example will start the current process without opening a new console window.Using PowerShell community extension versions, a new object is created first. Once the object is made, the WaitForExit()
function is called.
$proc = Start-Process notepad.exe -NoNewWindow -PassThru
$proc.WaitForExit()
Using Background Jobs to Wait for Each Command to Finish in PowerShell
Another method of making Windows PowerShell wait for the command line to finish is using a background job.
A background job is a command or set of commands run in a separate process in the local machine. Example syntax of a background job is shown below.
$job = Start-Job { notepad.exe }
Wait-Job $job
Receive-Job $job
Calling the Wait-Job
cmdlet will make the process wait until the Receive-Job
is called in the command line.
Using the Wait-Process
Cmdlet to Wait for Each Command to Finish in PowerShell
Another method of making Windows PowerShell wait for the command line to finish is the Wait-Process
cmdlet.
Like our first example, we can pipe this into our executable file and wait for the command line to finish. However, using this cmdlet only works on Windows PowerShell versions 5.1 and below and will not work with the latest PowerShell (Core) 7.
Notepad.exe | Wait-Process
Using the [Diagnostics.Process]
Class to Wait for Each Command to Finish in PowerShell
If you have a .NET
library installed in your system, you can use the [Diagnostics.Process]
class to wait for your processes to finish before moving to the following command line.
However, we do not recommend using this as other simpler cmdlets are available inside Windows PowerShell that we can fully utilize. Only use .NET
libraries if PowerShell commands are not available.
[Diagnostics.Process]::Start('notepad.exe').WaitForExit()
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn