Logical Operators in PowerShell
- PowerShell Logical Operators
-
the
-and
Operator in PowerShell -
the
-or
Operator in PowerShell -
the
-xor
Operator in PowerShell -
the
-not
Operator in PowerShell
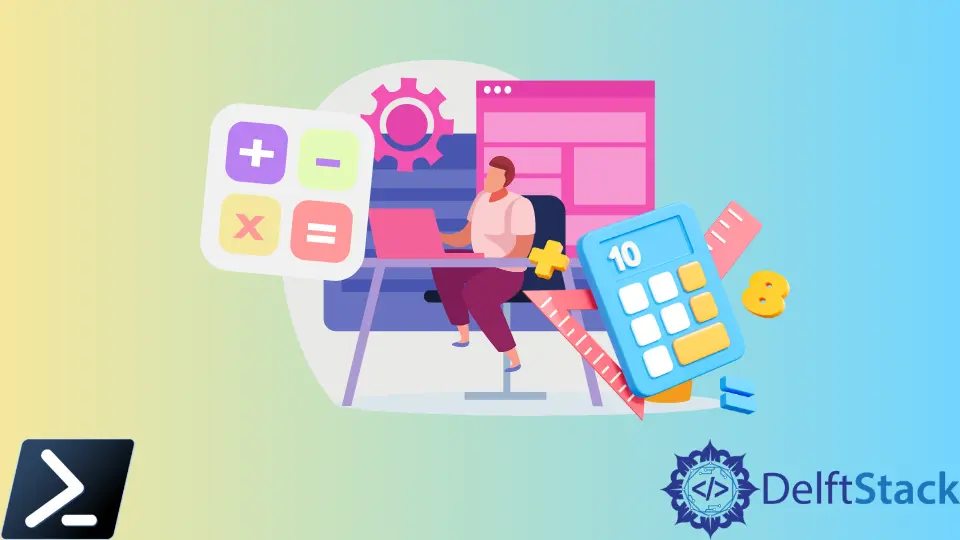
Logical operators can convert various conditions into a single condition.
This article will discuss real-world examples and apply logical operators in script with PowerShell.
PowerShell Logical Operators
Logical operators are and
, or
, xor
, and not
or !
.
the -and
Operator in PowerShell
The output is true
if $a
and $b
are true
; otherwise, false
.
Truth Table:
A | B | Output |
---|---|---|
0 | 0 | 0 |
1 | 0 | 0 |
0 | 1 | 0 |
1 | 1 | 1 |
$a = 0
$b = 0
$a -and $b # false (if both variables are false)
$a = 1
$b = 0
$a -and $b # false (if any of the variables are false)
$a = 1
$b = 1
$a -and $b # true (if both variables are true)
The -and
operator returns true
only when both are true. In general, the -and
operators are used where we want all conditions to be checked and fulfilled.
Here is an example of both conditions that need to be fulfilled.
$attendance = 102
$paid = "Y"
if ($attendance -gt 100 -and $paid -eq "Y") {
Write-Output "Allow for examination."
}
Output:
Allow for examination.
the -or
Operator in PowerShell
The output is false
if $a
and $b
is false
, compared to the -and
operator.
The -or
operator only needs one variable to be true
to output true
.
Truth Table:
A | B | Output |
---|---|---|
0 | 0 | 0 |
1 | 0 | 1 |
0 | 1 | 1 |
1 | 1 | 1 |
$a = 0
$b = 0
$a -or $b # false (if both conditions are false)
$a = 1
$b = 0
$a -or $b # true (if any of the variables are true)
$a = 1
$b = 1
$a -or $b # true (if both of the variables are true)
The -or
operator returns false
only when both conditions are false
. In general, the -or
operators are used when considering any conditions as true
.
$attendance = 99
$marks = 201
if ($attendance -gt 100 -or $marks -gt 200) {
Write-Output "Give five extra marks."
}
Output:
Give five extra marks.
the -xor
Operator in PowerShell
The exclusive or
or -xor
results from true
if only one of $a
or $b
is true
. If both conditions are true
, -xor
yields a result of false
.
Truth Table:
A | B | Output |
---|---|---|
0 | 0 | 0 |
1 | 0 | 1 |
0 | 1 | 1 |
1 | 1 | 0 |
('a' -eq 'A') -xor ('a' -eq 'z') # true as one of them is true
('a' -eq 'A') -xor ('Z' -eq 'z') # false as both of them is true
('a' -eq 's') -xor ('Z' -eq 'p') # false as both of them are false
the -not
Operator in PowerShell
The -not
operator returns the opposite of the expression output. If the output of the expression is true
, the operator will return it as false
, and vice-versa.
-not ('a' -eq 'a') # false as the output of expression is true
-not ('v' -eq 'a') # true as output expression is false
-not ('v' -eq 'V') # false as output expression is true
-not ('V' -eq 'V1') # true as output expression is false
The exclamation point !
is the same as the -not
operator.
!('a' -eq 'a') # false as the output of expression is true
!('v' -eq 'a') # true as output expression is false
!('v' -eq 'V') # false as output expression is true
!('V' -eq 'V1') # true as output expression is false
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn