How to Use Global Variables in PowerShell
- Introduction to PowerShell Global Variables
- Benefits of PowerShell Global Variables
- Declare a PowerShell Global Variable
- Pass the Global Variable as a Parameter in PowerShell
-
Use
Set-Variable
to Set Values to a Global Variables in PowerShell
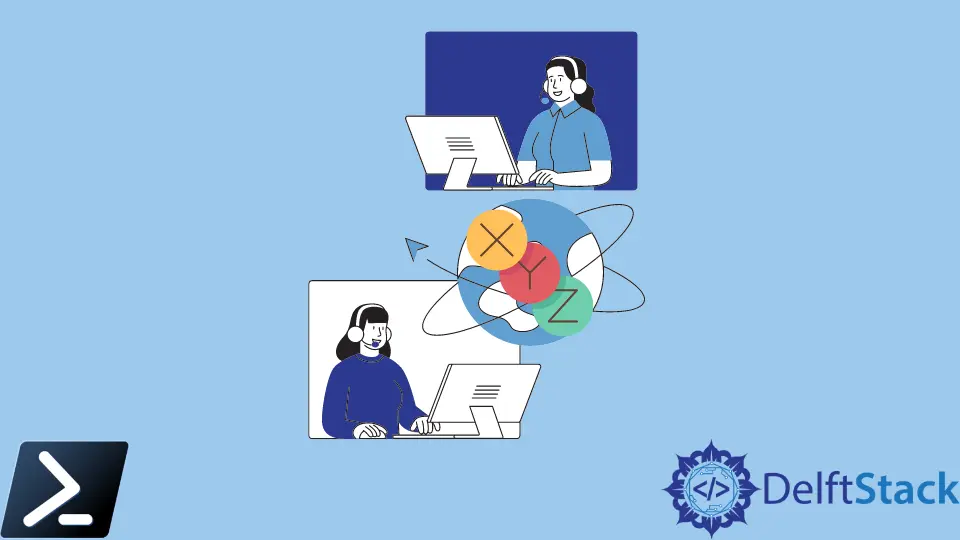
Variables are objects that can store specific values for later processing or display their importance to the user. There are many types of variables in Windows PowerShell.
We will discuss the Windows PowerShell global variables and implement them in PowerShell.
Introduction to PowerShell Global Variables
Every variable has a specific scope. When we define a custom variable inside a function, it can be accessed only within that function and not outside.
Example Code:
function sampleFunction {
$firstvar = 35;
Write-Host $firstvar;
}
Write-Output $firstvar
The script’s output above will be null
because that’s the default value of any variable in PowerShell. In that case, we should use a global variable as it is accessible to scripts, functions, and commands across the session to access a variable throughout your program and other methods.
Benefits of PowerShell Global Variables
- Global variables stores all values such as strings, integers, arrays, objects, and hash tables.
- Global variables can contain a collection of different objects.
- A specific amount of memory is allocated to every global variable.
- Every global variable must be preceded by the dollar sign (
$
) sign. - It is a best practice in programming to declare a global variable right at the beginning of a program.
- If we don’t assign a value to your variable, it will be valued as null by default.
- The name of a global variable can contain a combination of numbers, alphabets, and an underscore (
_
) sign. If we want to use other special characters, enclose the full name of the variable with braces.
Declare a PowerShell Global Variable
To declare a PowerShell global variable, use the below syntax.
Example Code:
$global: myVariable = "apple"
If we choose not to give any value, you can explicitly assign a $null
value.
Example Code:
$global: myVariable2 = $null
We have to mention the $global
to let PowerShell know that this variable should be available throughout the program.
The following code shows that the local and global variables share the same name. So, the local variable will take precedence inside the function, but the local variable had no value outside the function, so the script used the global one.
Example Code:
$global:num1 = 55;
function calculateAge {
$num1 = 45;
Write-Host $num1 "inside the function";
}
Write-Host $num1 "outside the function";
calculateAge;
Output:
45 inside the function
55 outside the function
Pass the Global Variable as a Parameter in PowerShell
We can also pass a global variable as a parameter to a function and set its value inside the function. But of course, you’ll have to give the global variable as a REF
object.
Example Code:
$global:finalVariable = $null;
function addValue ($num1, $num2, [REF]$ans) {
$ans.Value = $num1 + $num2;
}
addValue 2 3 ([REF]$global:finalVariable);
Write-Host "The final value is:" $global:finalVariable;
Output:
The final value is: 5
Use Set-Variable
to Set Values to a Global Variables in PowerShell
The simplest way to set the values to a Windows PowerShell global variable is to use the Set-Variable
cmdlets.
Example Code:
Set-Variable -Name "finalVariable" -Value "6" -Scope Global
Get-Variable -Name "finalVariable"
Output:
Name Value
---- -----
finalVariable 6
The script will display the value of 6
. Note that we have to mention the variable’s scope as Global
.
The Set-Variable
command also comes with an optional parameter that we can use to determine the nature of the global variable.
Example Code:
Set-Variable -Name "finalVariable" -Value "6" -Scope Global -Option Read-Only
The above code will generate a global variable and set a value to it, but it will be a Read-Only
global variable.
Example Code:
Set-Variable -Name "finalVariable" -Value "6" -Scope Global -Option Constant
Now that we know about global variables, a word of caution before you start using them in your program. A PowerShell global variable is not preferred to generate variables or store values because we have no access control.
In other words, it is difficult to keep track of the value of a global variable as we can change it anywhere in a program and testing them is very difficult as they are tightly coupled with the code.
These are why experienced programmers often don’t use global variables, as they can lead to some difficult and hard-to-find bugs in extensive programs.
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn