How to Use Credential Manager Module in PowerShell
- Use the Credential Manager Module in PowerShell
- Create, Retrieve, and Remove Stored Credentials in PowerShell
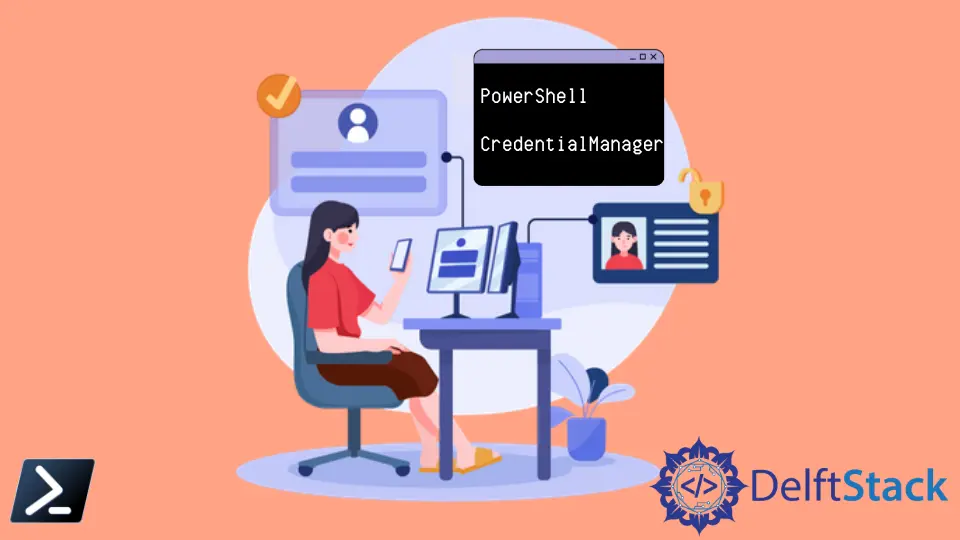
PowerShell is the best tool for regulating Credential Manager at scale. Unfortunately, the latest Windows operating system build does not include PowerShell cmdlets.
Thankfully, we have a PowerShell module for Credential Manager and made it available through the PowerShell gallery.
This article will discuss installing the Credential Manager module in PowerShell and utilizing its commands to manage our stored passwords.
Use the Credential Manager Module in PowerShell
To utilize this module, open an elevated Windows PowerShell window and then enter the following command:
Install-Module -Name CredentialManager
The command above will install the Credential Manager module without us having to download anything manually.
Unfortunately, there aren’t many guides or documentation for the Credential Manager module. However, the module is relatively easy to use. The Credential Manager module is comprised of three commands:
Get-StoredCredential
New-StoredCredential
Remove-StoredCredential
As with any other PowerShell cmdlet, we can display the syntax for any of these cmdlets by using PowerShell’s Get-Help
cmdlet. We have to type Get-Help
, followed by the cmdlet’s name that we need help with.
For example, if we wanted to see the syntax for the Get-StoredCredential
cmdlet, we would type:
Get-Help Get-StoredCredential
Create, Retrieve, and Remove Stored Credentials in PowerShell
Let’s look at how to create, retrieve, and then remove a stored credential.
Create Credentials
There are several ways of storing a credential. One method involves keying in a password in clear text and then writing that password directly to the credential manager.
First, let’s look at the clear text method.
Suppose that we wanted to store a password for a server named Contoso for a moment. Let’s also assume that our password is “password” and our username is “User1”.
Then, the command that we would use to enter that information into the Credential Manager is:
Example code:
New-StoredCredential -Target Contoso -Username User1 -Password Password
Output:
Flags : 0
Type : Generic
TargetName : Contoso
Comment : Updated by: User on: 3/30/2022
LastWritten : 3/30/2022 12:18:55 AM
PaswordSize : 16
Password : Password
Persist : Session
AttributeCount : 0
Attributes : 0
TargetAlias :
UserName : User1
If, for example, we wanted to ask “User2” for their password for the Contoso server, the command would look more like this:
Get-Credential -UserName User2 -Message "Enter your password:" | New-StoredCredential -Target Contoso
When we run this snippet of code above, the user sees a password prompt. This prompt is similar to when running the ‘Get-Credential’ command.
Once the user enters the password, the script will display the output identical to our previous example. However, the password prompt and the result are not on the screen simultaneously in real life.
Retrieve Stored Credentials
So now that we have shown you how to enter credentials into Credential Manager, let’s look at how to retrieve credentials. For example, if we wanted to retrieve User2’s credentials for Contoso, we could do so by entering this command:
Example code:
Get-StoredCredential -Target Contoso
Output:
Username Password
-------- --------
User1 System.Security.SecureString
The password is displayed as a secure string object, as shown in the output above. The password isn’t exposed on the screen.
In addition, the password is in a format that a PowerShell script can natively use since it has been transformed into a PowerShell object. We could map the cmdlet to a variable and store it there:
$Cred = Get-StoredCredential -Target Contoso
Remove Stored Credentials
We no longer wish to cache credentials for the Contoso server. Instead, we can purge the credentials from the Credential Manager. Enter the Remove-StoredCredential
cmdlet followed by the Target switch and the server’s name.
We can also use the Type switch to specify the credential type (Type Generic
).
Just a quick note, be careful in running the remove command. Ensure the Target switch value is written correctly, or accidental removal of passwords already stored in the Credential Manager may occur.
Here is a command that we could use to remove the cached credential for the Contoso server:
Remove-StoredCredential -Target Contoso
When we use this command, PowerShell generates no visible output. We can verify the operation’s success by leveraging the Get-StoredCredential
cmdlet.
We can type Get-StoredCredential
, followed by the Target switch and the target’s name. Below is the command.
Get-StoredCredential -Target Contoso
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn