How to Suppress PowerShell Errors
- Introduction to Error Action in PowerShell
-
Use the
-ErrorAction
Parameter in PowerShell - Setting Error Action Preferences in PowerShell
-
Use the
Try-Catch
Statement Parameter in PowerShell - Conclusion
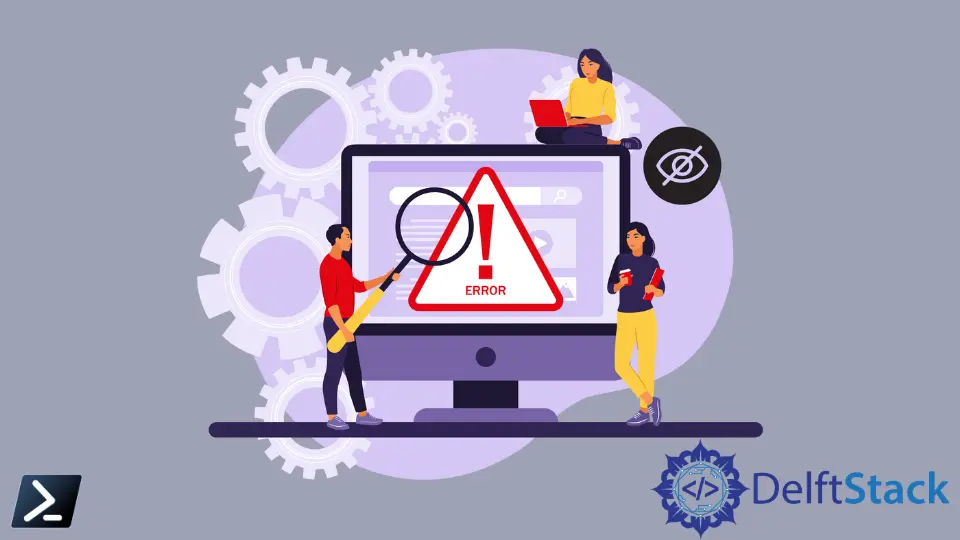
This article delves into the intricate world of PowerShell error handling, exploring various methods such as the -ErrorAction
parameter, setting error action preferences, and employing the Try-Catch
statement. Each of these methods serves a unique purpose, from silently continuing script execution despite errors to implementing sophisticated error handling mechanisms.
This detailed exploration is designed to equip both novice and seasoned scripters with the knowledge to make informed decisions about error handling in their PowerShell scripts, ensuring they can effectively manage unexpected situations and maintain script integrity.
Introduction to Error Action in PowerShell
Even though it is effortless to suppress Windows PowerShell errors, doing so isn’t always the best option (although it can be). If we carelessly tell PowerShell to hide errors, it can cause our script to behave unpredictably.
Suppressing error messages also makes troubleshooting and information gathering a lot more complicated. So tread lightly and be careful about using the following snippets that you will see in this article.
Use the -ErrorAction
Parameter in PowerShell
The -ErrorAction
parameter in PowerShell is designed to dictate how a cmdlet responds to runtime errors. By default, PowerShell will display errors to the console and continue execution.
However, in certain scenarios, you might want to alter this behavior - for instance, to suppress error messages or to halt execution when an error occurs. This is where -ErrorAction
becomes crucial.
Command:
Get-Service 'svc_not_existing' -ErrorAction SilentlyContinue
Write-Host "This line will execute regardless of the above command's result."
In our script, we attempt to retrieve a service named 'svc_not_existing'
, a service that presumably doesn’t exist. By applying -ErrorAction SilentlyContinue
, we instruct PowerShell to carry on without interruption, avoiding the display of an error message should it fail to locate the service.
Subsequently, our script transitions seamlessly to the Write-Host
command. This command outputs a specific message to the console.
Output:
Setting Error Action Preferences in PowerShell
If we need a script to behave in a certain way (such as suppressing errors), we might consider setting up some preference variables. Preference variables act as configuration settings for PowerShell.
We might use a preference variable to control the number of history items that PowerShell retains or force PowerShell to ask the user before performing specific actions.
For example, here is how you can use a preference variable to set the -ErrorAction
parameter to SilentlyContinue
for the entire session.
Command:
$ErrorActionPreference = 'SilentlyContinue'
Get-Service 'nonexistent_service'
Write-Host "This message is displayed even if the previous command fails."
In our script, we begin by setting $ErrorActionPreference
to 'SilentlyContinue'
. This action ensures that the setting is applied to all subsequent commands within our script.
As a result, any errors that would typically emerge are effectively suppressed.
Next, we employ the Get-Service
cmdlet to try and retrieve a non-existent service. Under normal circumstances, this would trigger an error. However, thanks to our prior setting of $ErrorActionPreference
, any error that would have been generated remains unseen and unobtrusive.
Following this, our script gracefully moves on to the Write-Host
command. This command is responsible for displaying a message on the console.
Output:
There are many other error actions that we can specify for the ErrorAction
switch parameter.
Continue
: PowerShell will display the error message, but the script will continue to run.Ignore
: PowerShell does not produce any error message, writes any error output on the host, and continues execution.Stop
: PowerShell will display the error message and stop running the script.Inquire
: PowerShell displays the error message but will ask for confirmation first if the user wants to continue.SilentlyContinue
: PowerShell silently continues with code execution if the code does not work or has non-terminating errors.Suspend
: PowerShell suspends the workflow of the script.
Use the Try-Catch
Statement Parameter in PowerShell
The Try-Catch
statement is a powerful tool in PowerShell for managing and responding to errors in a controlled and sophisticated manner. It allows scripters to try
a block of code and catch
any exceptions (errors) that occur during its execution.
This approach is beneficial when you want to handle errors gracefully, perform specific actions when an error occurs, or prevent errors from abruptly ending your script.
Command:
try {
Get-Service 'nonexistent_service'
}
catch {
Write-Host "An error occurred: $_"
}
In this script, we first encapsulate a potentially error-prone command, Get-Service 'nonexistent_service'
, within a try
block. We’re attempting to retrieve a service that likely doesn’t exist, which would normally result in an error.
However, rather than allowing this error to terminate the script or display an unhandled exception, we catch it. In the catch
block, we then execute a Write-Host
command to display a custom error message, including the error details ($_
represents the current error object).
Output:
Conclusion
As we conclude our journey through the different facets of error handling in PowerShell, it’s clear that the power of this scripting language lies in its flexibility and depth. From the simplicity of the -ErrorAction
parameter to the nuanced control offered by Try-Catch
statements, PowerShell provides a rich toolkit for managing errors in a variety of scenarios.
Understanding these tools and knowing when to apply them is essential for writing robust, resilient scripts. We’ve explored how suppressing errors can be both a boon and a risk, depending on the context, and looked at how to capture errors for later analysis.
By now, you should have a comprehensive understanding of these methods and be well-equipped to apply them in your scripting endeavors.
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn