How to Sending a Mouse Click Event in PowerShell
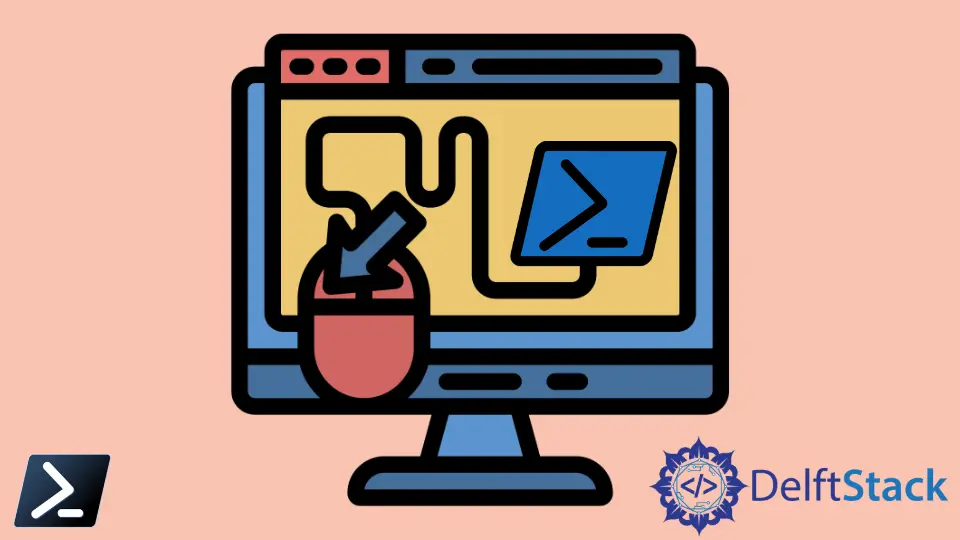
When using Windows PowerShell, there will be unusual requests that we may or may not encounter when developing a script for an organization or client, and one example of this is the recording and sending of keystrokes. It is unsuitable for PowerShell to handle keystrokes as the function is how far the scripting library goes, but it is possible to execute in PowerShell despite its limitations.
Since it is a complex topic, in this article, we will focus more on creating and building the send input structure and calling the send mouse click class in our script.
Use the Mouse Event Function in PowerShell
The mouse event function mouse_event()
is derived from the Win32 API winuser.h
, which calls an API from the Microsoft Developer Network (MSDN) specifically from the winuser.h
API. The API was developed to call an external API that will handle mouse events, including mouse clicks.
Let’s prepare our $cSource
variable to establish our INPUT
structure and import all the libraries we need to send a mouse event.
$cSource = @'
using System;
using System.Drawing;
using System.Runtime.InteropServices;
using System.Windows.Forms;
public class Clicker{
[StructLayout(LayoutKind.Sequential)]
struct INPUT
{
public int 0;
public MOUSEINPUT mi;
}
//continued on the following script block
We have declared our INPUT
structure, which we can use and call a sub-structure called the MOUSEINPUT
structure. Remember that the following snippet is still inside the Clicker
class.
[StructLayout(LayoutKind.Sequential)]
struct MOUSEINPUT
{
public int dx ;
public int dy ;
public int mouseData ;
public int dwFlags;
public int time;
public IntPtr dwExtraInfo;
}
In some cases, there will be advanced mice that have additional buttons, but for now, since we are only focusing on the left mouse click, we can declare the frequently used constants below. Notice that we also have declared a constant for the screen length of your monitor.
const int MOUSEEVENTF_MOVED = 0x0001 ;
const int MOUSEEVENTF_LEFTDOWN = 0x0002 ;
const int MOUSEEVENTF_LEFTUP = 0x0004 ;
const int MOUSEEVENTF_RIGHTDOWN = 0x0008 ;
const int MOUSEEVENTF_RIGHTUP = 0x0010 ;
const int MOUSEEVENTF_MIDDLEDOWN = 0x0020 ;
const int MOUSEEVENTF_MIDDLEUP = 0x0040 ;
const int MOUSEEVENTF_WHEEL = 0x0080 ;
const int MOUSEEVENTF_XDOWN = 0x0100 ;
const int MOUSEEVENTF_XUP = 0x0200 ;
const int MOUSEEVENTF_ABSOLUTE = 0x8000 ;
const int screen_length = 0x10000 ;
Now, we are ready to create a function that will specifically send a left-click event on the screen. The function accepts two arguments that will determine the location on the monitor where the left click event will happen.
[System.Runtime.InteropServices.DllImport("user32.dll")]
extern static uint SendInput(uint nInputs, INPUT[] pInputs, int cbSize);
public static void LeftClickAtPoint(int x, int y)
{
//Move the mouse
INPUT[] input = new INPUT[3];
input[0].mi.dx = x*(65535 / System.Windows.Forms.Screen.PrimaryScreen.Bounds.Width);
input[0].mi.dy = y*(65535 / System.Windows.Forms.Screen.PrimaryScreen.Bounds.Height);
input[0].mi.dwFlags = MOUSEEVENTF_MOVED | MOUSEEVENTF_ABSOLUTE;
//Left mouse button down
input[1].mi.dwFlags = MOUSEEVENTF_LEFTDOWN;
//Left mouse button up
input[2].mi.dwFlags = MOUSEEVENTF_LEFTUP;
SendInput(3, input, Marshal.SizeOf(input[0]));
}
} # Close the Clicker Class
'@ #Close the $cSource variable
To call our left-click function, we must assemble the library under the Add-Type
cmdlet. Once declared, we can now call our custom class [Clicker]::LeftClickAtPoint(x,y)
to send a left-click mouse event on the screen.
Add-Type -TypeDefinition $cSource -ReferencedAssemblies System.Windows.Forms, System.Drawing
[Clicker]::LeftClickAtPoint(300, 300)
Full Script:
$scSource = @'
using System;
using System.Drawing;
using System.Runtime.InteropServices;
using System.Windows.Forms;
public class Clicker
{
[StructLayout(LayoutKind.Sequential)]
struct INPUT
{
public int type; // 0 = INPUT_MOUSE,
// 1 = INPUT_KEYBOARD
// 2 = INPUT_HARDWARE
public MOUSEINPUT mi;
}
[StructLayout(LayoutKind.Sequential)]
struct MOUSEINPUT
{
public int dx ;
public int dy ;
public int mouseData ;
public int dwFlags;
public int time;
public IntPtr dwExtraInfo;
}
const int MOUSEEVENTF_MOVED = 0x0001 ;
const int MOUSEEVENTF_LEFTDOWN = 0x0002 ;
const int MOUSEEVENTF_LEFTUP = 0x0004 ;
const int MOUSEEVENTF_RIGHTDOWN = 0x0008 ;
const int MOUSEEVENTF_RIGHTUP = 0x0010 ;
const int MOUSEEVENTF_MIDDLEDOWN = 0x0020 ;
const int MOUSEEVENTF_MIDDLEUP = 0x0040 ;
const int MOUSEEVENTF_WHEEL = 0x0080 ;
const int MOUSEEVENTF_XDOWN = 0x0100 ;
const int MOUSEEVENTF_XUP = 0x0200 ;
const int MOUSEEVENTF_ABSOLUTE = 0x8000 ;
const int screen_length = 0x10000 ;
[System.Runtime.InteropServices.DllImport("user32.dll")]
extern static uint SendInput(uint nInputs, INPUT[] pInputs, int cbSize);
public static void LeftClickAtPoint(int x, int y)
{
INPUT[] input = new INPUT[3];
input[0].mi.dx = x*(65535/System.Windows.Forms.Screen.PrimaryScreen.Bounds.Width);
input[0].mi.dy = y*(65535/System.Windows.Forms.Screen.PrimaryScreen.Bounds.Height);
input[0].mi.dwFlags = MOUSEEVENTF_MOVED | MOUSEEVENTF_ABSOLUTE;
input[1].mi.dwFlags = MOUSEEVENTF_LEFTDOWN;
input[2].mi.dwFlags = MOUSEEVENTF_LEFTUP;
SendInput(3, input, Marshal.SizeOf(input[0]));
}
}
'@
Add-Type -TypeDefinition $scSource -ReferencedAssemblies System.Windows.Forms, System.Drawing
[Clicker]::LeftClickAtPoint(300, 300)
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn