How to Send Email Message to Multiple Recipients in PowerShell
- Using PowerShell’s Send-MailMessage Cmdlet
- Sending Emails with Attachments
- Scheduling Emails to Multiple Recipients
- Conclusion
- FAQ
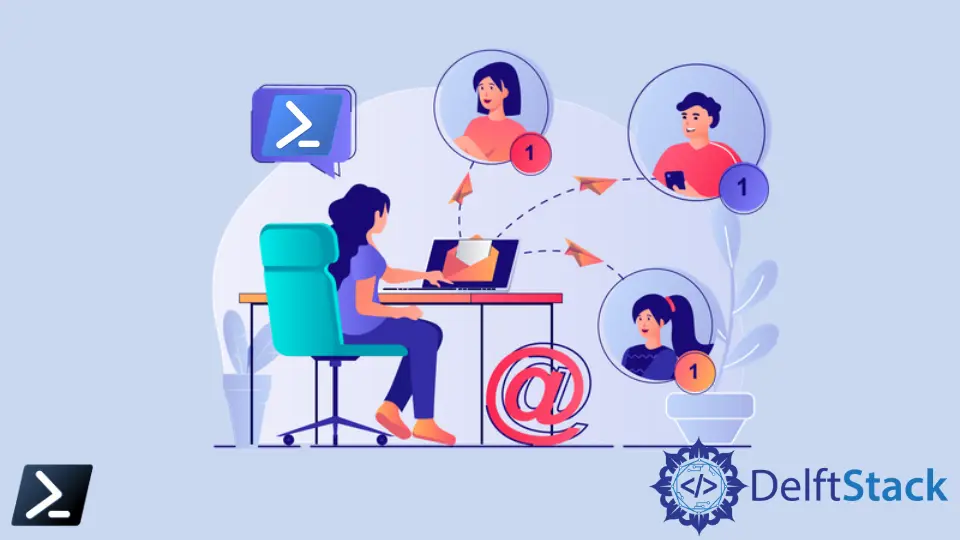
Sending emails to multiple recipients can be a daunting task, especially if you need to do it frequently. Fortunately, PowerShell offers a straightforward way to streamline this process. Whether you’re notifying your team about an update or sending out a newsletter, knowing how to send email messages to multiple recipients using PowerShell can save you time and effort.
In this article, we will explore the commands and methods you can use to send emails to multiple recipients efficiently. We will break down the process into manageable steps, providing clear examples and explanations along the way. Let’s dive in!
Using PowerShell’s Send-MailMessage Cmdlet
One of the simplest methods to send an email to multiple recipients in PowerShell is by using the Send-MailMessage
cmdlet. This cmdlet allows you to specify multiple email addresses in the -To
parameter, making it easy to reach everyone at once. Below is a basic example of how to implement this:
$EmailFrom = "youremail@example.com"
$EmailTo = "recipient1@example.com, recipient2@example.com"
$Subject = "Important Update"
$Body = "Hello Team, this is an important update regarding our project."
$SMTPServer = "smtp.example.com"
Send-MailMessage -From $EmailFrom -To $EmailTo -Subject $Subject -Body $Body -SmtpServer $SMTPServer
Output:
Email sent successfully to recipient1@example.com and recipient2@example.com.
In this example, we first define the sender’s email address, the recipients’ email addresses (separated by commas), the email subject, and the body content. The -SmtpServer
parameter specifies the SMTP server used to send the email. This straightforward approach is effective for sending simple messages to multiple recipients.
When using Send-MailMessage
, ensure that your SMTP server settings are correct. Additionally, consider using secure connections (like TLS) if your SMTP server supports it. This method is ideal for quick notifications or updates without the need for complex configurations.
Sending Emails with Attachments
If you need to send emails with attachments to multiple recipients, PowerShell can handle that too. You can add the -Attachments
parameter to include files in your email. Here’s how to do it:
$EmailFrom = "youremail@example.com"
$EmailTo = "recipient1@example.com, recipient2@example.com"
$Subject = "Project Documents"
$Body = "Attached are the project documents for your review."
$SMTPServer = "smtp.example.com"
$Attachment = "C:\path\to\your\document.pdf"
Send-MailMessage -From $EmailFrom -To $EmailTo -Subject $Subject -Body $Body -SmtpServer $SMTPServer -Attachments $Attachment
Output:
Email sent successfully with attachment to recipient1@example.com and recipient2@example.com.
In this example, we introduced an attachment by specifying its path in the $Attachment
variable. The Send-MailMessage
cmdlet will then include this file in the email sent to all specified recipients. This method is particularly useful for sharing important documents or reports without needing to send separate emails.
When sending attachments, ensure that the file paths are correct and that the recipients have the necessary permissions to access the files. Additionally, be mindful of attachment sizes, as large files may be rejected by some email servers.
Scheduling Emails to Multiple Recipients
PowerShell also allows you to schedule emails to be sent at a later time. This can be particularly handy for reminders or notifications that need to be sent at specific intervals. To achieve this, you can combine the Send-MailMessage
cmdlet with the Start-Sleep
cmdlet to delay execution. Here’s an example:
$EmailFrom = "youremail@example.com"
$EmailTo = "recipient1@example.com, recipient2@example.com"
$Subject = "Scheduled Reminder"
$Body = "This is a reminder for our upcoming meeting."
$SMTPServer = "smtp.example.com"
Start-Sleep -Seconds 60
Send-MailMessage -From $EmailFrom -To $EmailTo -Subject $Subject -Body $Body -SmtpServer $SMTPServer
Output:
Email sent successfully after a delay of 60 seconds to recipient1@example.com and recipient2@example.com.
In this example, we used Start-Sleep
to pause the script for 60 seconds before sending the email. You can adjust the duration as needed. This method is particularly useful when you want to send reminders or alerts at specific times without manually triggering the email.
For more complex scheduling, consider using Windows Task Scheduler to run your PowerShell script at predetermined times. This allows for greater flexibility and automation, making it easier to manage recurring notifications.
Conclusion
Using PowerShell to send email messages to multiple recipients is a powerful way to enhance communication in any organization. With the Send-MailMessage
cmdlet, you can easily send simple messages, include attachments, and even schedule emails for future delivery. By mastering these techniques, you can save time and ensure that your messages reach the right people promptly. Whether you’re sending updates, reminders, or important documents, PowerShell provides a reliable solution for your email needs.
FAQ
-
How do I send an email to multiple recipients using PowerShell?
You can use theSend-MailMessage
cmdlet and specify multiple recipients in the-To
parameter, separating them with commas. -
Can I send attachments with my email in PowerShell?
Yes, you can use the-Attachments
parameter in theSend-MailMessage
cmdlet to include files in your email. -
Is it possible to schedule emails in PowerShell?
Yes, you can use theStart-Sleep
cmdlet to delay sending an email or use Windows Task Scheduler to run your PowerShell script at a specific time. -
What SMTP server should I use for sending emails?
You should use a valid SMTP server that you have access to, such as those provided by your email service provider. -
Are there any security considerations when sending emails with PowerShell?
Yes, ensure that you use secure connections (like TLS) when sending emails and verify that your SMTP server settings are configured correctly.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.