How to Send Emails Using PowerShell
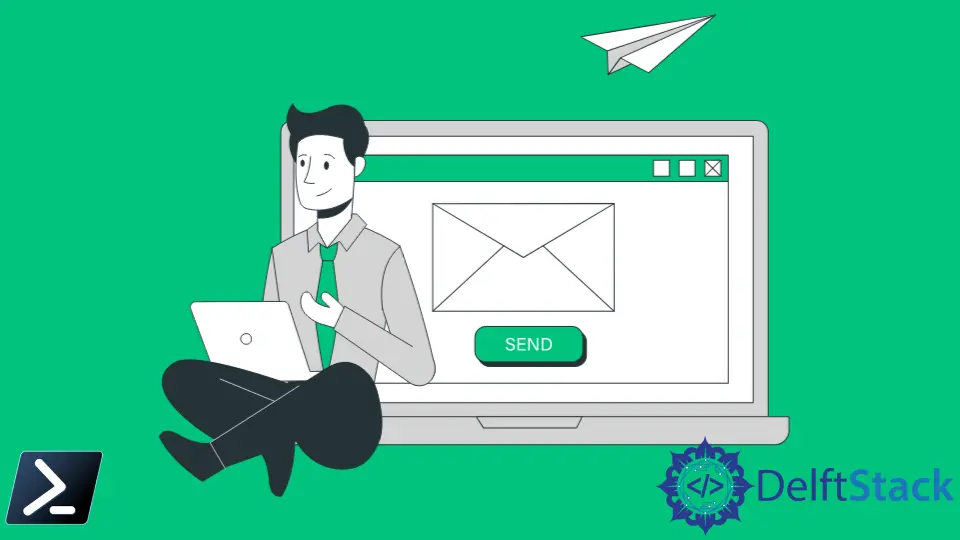
Windows PowerShell is a command-line shell used to solve some administration tasks in Windows and apps running on this OS. A common scripting language allows you to tailor cmdlets to perform specific functions.
Sending emails is one of them. This article will discuss using Windows PowerShell to send emails and other ways to handle this method.
Send Emails Using Send-MailMessage
Cmdlet in PowerShell
Here is an example based on the Send-MailMessage
cmdlet you can use right now to send an email from PowerShell using SMTP protocol.
Send-MailMessage -To "user@contoso.com" -From "user@abccompany.net" -Subject "Hello!" -Body "This is important" -Credential (Get-Credential) -SmtpServer "smtp.gmail.com" -Port 587
All we need is to insert the email address of the sender and the recipient and specify the SMTP server that we are going to use.
The Send-MailMessage
cmdlet also lets you put more details on your email with the command’s many parameters. Here is the list of parameters that we can use using the cmdlet.
Parameter | Description |
---|---|
-To |
Email address of the destination recipient or recipients. |
-Bcc |
Email address that will be Blind Carbon Copied. |
-Cc |
Email address that will be Carbon Copied. |
-From |
The sender or source email address. |
-Subject |
Subject of the email. |
-Body |
The body of the email. |
-BodyAsHTML |
Indicates that the body of the email has HTML tags. |
-Attachments |
Filenames to be attached to the name. |
-Credential |
Credentials used to send the email. |
-SMTPServer |
Name of the SMTP Server. |
-Port |
SMTP Server Port. |
-UseSSL |
Secure Sockets Layer (SSL) protocol will be used when sending the email. |
You can assign the parameter values to a variable first before inserting them into the command line for easier readability.
$From = "user@abccompany.net"
$To = "user@contoso.com"
$Copy = "manager@contoso.com"
$Attachment = "C:\Temp\file.jpg"
$Subject = "Photos of Drogon"
$Body = "<br>Please see attached picture for reference."
$SMTPServer = "smtp.gmail.com"
$SMTPPort = "465"
Send-MailMessage -From $From -To $To -Cc $Copy -Subject $Subject -Body $Body -SmtpServer $SMTPServer -Port $SMTPPort -UseSsl -Credential (Get-Credential) -Attachments $Attachment
By default, the Send-MailMessage
command tries to send an email via the standard SMTP port TCP 25.
However, suppose your SMTP server allows sending email only using an encrypted protocol. In that case, we can specify the port number the most often; it is 465 or 587 and the -UseSSL
switch parameter.
The table below shows the list of SMTP server parameters of the popular public email providers that we can use to send messages from Windows PowerShell (note that we will have to allow sending emails via SMTP in the account interface):
Service | SMTP Server | Port | Connection |
---|---|---|---|
Gmail | smtp.gmail.com |
587 | TLS |
25 | TLS | ||
465 | SSL | ||
Office 365 | smtp.office365.com |
587 | TLS |
25 | TLS | ||
Outlook.com | smtp-mail.outlook.com |
587 | TLS |
25 | TLS | ||
Yahoo Mail | smtp.mail.yahoo.com |
587 | TLS |
25 | TLS | ||
465 | SSL | ||
Windows Live Hotmail | smtp.live.com |
587 | TLS |
25 | TLS | ||
Zoho | smtp.zoho.com |
587 | TLS |
465 | SSL |
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn