How to Run CMD Commands in PowerShell
-
Using the PowerShell Invocation Operator
&
-
Running
CMD
Commands Usingcmd.exe
-
Piping to
CMD
Using PowerShell - Conclusion
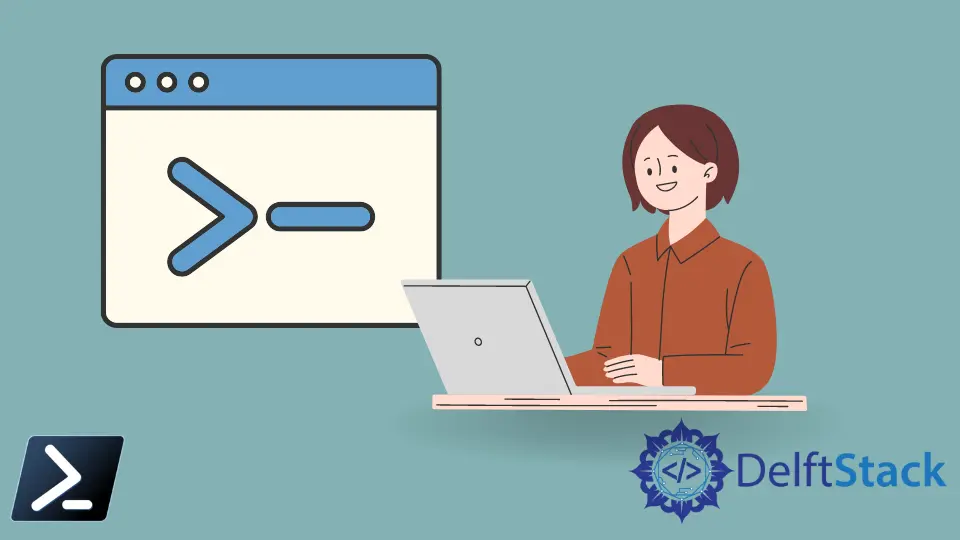
Many legacy Command Prompt (CMD
) commands work in the Windows PowerShell scripting environment. The PowerShell environment carries these commands forward from the most used commands like ping
to the most informational commands like tracert
from the legacy environment using aliases.
However, some running commands in the Command Prompt terminal will not work in Windows PowerShell’s scripting environment. This article will discuss how we can transcribe them correctly to Windows PowerShell.
Using the PowerShell Invocation Operator &
In the versatile world of scripting, PowerShell stands out for its ability to incorporate and execute commands from other scripting environments, notably the Command Prompt (CMD
). One of the most direct methods to achieve this is through the Invocation Operator (&
).
This article delves into the usage of the Invocation Operator for running CMD
commands within PowerShell, an invaluable skill for those looking to bridge the functionalities of PowerShell and traditional CMD
scripts.
The basic syntax for using the Invocation Operator is as follows:
& <Command-String> <Arguments>
&
: This is the Invocation Operator.<Command-String>
: This is the command you want to execute. This can be aCMD
command, a PowerShell cmdlet, or a script block.<Arguments>
: This is optional and represents any arguments or parameters that the command requires.
Example Code:
& cmd.exe /c echo "Hello from CMD"
In this script, we employ the Invocation Operator &
to seamlessly execute a CMD
command within PowerShell. Firstly, we call upon cmd.exe
, the designated executable for the Command Prompt, ensuring that we are working within the correct command-line environment.
We then pass the /c
parameter to cmd.exe
, a crucial instruction that directs the Command Prompt to execute our specified command and promptly terminate thereafter. Our command of choice, echo "Hello from CMD"
, is a straightforward yet effective demonstration, as it simply prints the message Hello from CMD
to the console.
Output:
This output demonstrates that the CMD
command echo
was successfully executed within PowerShell, displaying the intended message.
Running CMD
Commands Using cmd.exe
Another example of running CMD
commands is by using the cmd.exe
. We can add cmd.exe
inside Windows PowerShell like our previous method.
Once added and executed, it will call the command line interface inside the Windows PowerShell command prompt.
The syntax for using cmd.exe
directly in PowerShell is as follows:
cmd.exe /c "<Command-String>"
cmd.exe
: This is the executable for the Command Prompt./c
: This is a parameter that instructscmd.exe
to carry out the command specified by the string and then terminate."<Command-String>"
: This is theCMD
command or series of commands you want to execute.
Example Code:
cmd.exe /c "echo Hello from CMD"
In this script, we seamlessly integrate CMD
functionality into PowerShell by directly invoking cmd.exe
with the /c
parameter, followed by our command of interest, echo Hello from CMD
. By using cmd.exe /c
, we establish a bridge between PowerShell and CMD
, enabling us to tap into the familiar command-line environment and functionalities of CMD
within our PowerShell script.
The command "echo Hello from CMD"
is a straightforward yet illustrative example of this integration, where we utilize a basic CMD
command to print our desired text to the console.
Output:
This output demonstrates the successful execution of a CMD echo
command in PowerShell, displaying the message Hello from CMD
.
Piping to CMD
Using PowerShell
In Windows PowerShell, we can also send over commands to the command prompt terminal by piping in the cmd
cmdlet to the command you wanted to send over.
Piping to CMD
in PowerShell is primarily used for executing CMD
commands or batch scripts within a PowerShell context. This method is particularly useful when you need to run a CMD
command that doesn’t have a direct PowerShell equivalent or when you’re dealing with legacy CMD
scripts.
The basic syntax for piping to CMD
in PowerShell is:
"<Command-String>" | cmd.exe /c -
"<Command-String>"
: This is theCMD
command you wish to execute.|
: This is the PowerShell pipe operator, which passes the output of one command as input to another.cmd.exe /c -
: This calls the Command Prompt to execute the command passed to it via the pipe. The-
signifies thatcmd.exe
should expect the command from the standard input (stdin
).
Example Code:
"echo Hello from CMD" | cmd.exe
In this example, we use the piping technique to send the string "echo Hello from CMD"
into cmd.exe
, demonstrating an elegant blend of PowerShell’s piping capabilities with CMD
’s command execution.
Our journey begins with "echo Hello from CMD"
, a simple yet effective CMD
command encapsulated as a string, designed to display a specific message when executed in the CMD
environment.
We then employ the pipe operator |
, a powerful tool in PowerShell, to seamlessly forward this command string to cmd.exe
. The culmination of this process is observed in cmd.exe /c -
, where the -
symbol plays a crucial role, instructing cmd.exe
to attentively read and execute the command arriving from the pipeline.
Output:
This output is the result of the CMD echo
command, which prints Hello from CMD
to the screen.
Running CMD
Commands in PowerShell Using Invoke-Expression
A versatile and powerful method to execute CMD
commands within PowerShell is through the Invoke-Expression
cmdlet. This approach is particularly useful when you need to dynamically construct and execute a command or a script block.
This article delves into the purpose, application, and usage of the Invoke-Expression
method in PowerShell for running CMD
commands.
The syntax for Invoke-Expression
is:
Invoke-Expression -Command "<Command-String>"
-Command
: This specifies the command to execute. This parameter can be abbreviated as-C
."<Command-String>"
: This is the string that contains the command to be executed.
Example Code:
Invoke-Expression -Command "cmd.exe /c echo Hello from CMD"
In our script, we harness the power of Invoke-Expression
to adeptly execute a CMD
command encapsulated within a string. Our process begins with Invoke-Expression -Command
, a pivotal cmdlet and parameter combination that equips PowerShell with the ability to interpret and execute the provided command string.
We then introduce "cmd.exe /c echo Hello from CMD"
as our chosen command string. This string deftly instructs cmd.exe
to utilize the /c
parameter, a directive that commands CMD
to execute the subsequent command and then conclude its process.
The echo
command, nestled within this string, is the heart of our execution, designed to output Hello from CMD
in CMD
.
Output:
This output is the direct result of the echo
command being processed by CMD
, which is invoked by Invoke-Expression
in PowerShell.
Conclusion
In this comprehensive exploration, we delved into the versatility of running Command Prompt (CMD
) commands within the Windows PowerShell environment, covering four distinct methods: the straightforward Invocation Operator &
, directly using cmd.exe
, the innovative technique of piping commands to CMD
, and the dynamic Invoke-Expression
cmdlet. Each approach offers unique advantages for integrating CMD
’s familiar functionalities into PowerShell’s advanced scripting realm.
For those seeking to further their PowerShell expertise, delving into advanced topics like Creating Custom PowerShell Cmdlets or Advanced PowerShell Scripting Techniques is highly recommended, opening doors to more sophisticated and robust scripting solutions that harmoniously blend the strengths of both PowerShell and CMD
.
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn