How to Round Numbers in PowerShell
- Truncating Numbers in PowerShell
- Rounding Down to Whole Numbers in PowerShell
- Rounding Up to Whole Numbers in PowerShell
- Rounding Half Up (Arithmetic Rounding) in PowerShell
- Rounding in General in PowerShell
- Conclusion
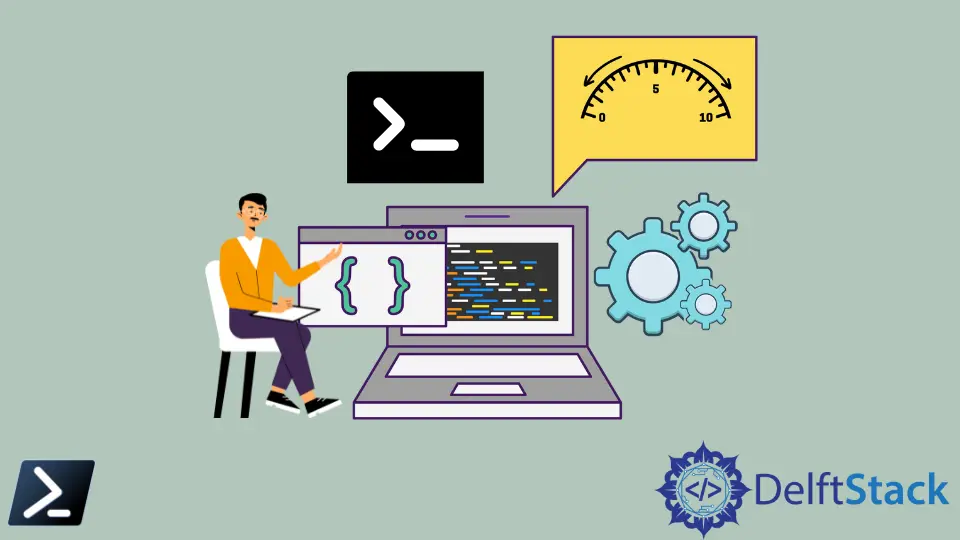
When working with numbers in PowerShell, there are various scenarios where you might need to round them to specific decimal places or convert them to whole numbers.
This article explores how to achieve precise number rounding in PowerShell using functions from the .NET framework’s Math class.
Truncating Numbers in PowerShell
Truncating in PowerShell may not precisely be a function that rounds a number but is still considered to calculate an integral part of the number. In other words, the method drops the decimal part of the integer, rounding it down to the nearest whole number.
To truncate (or crop) a number, we will be using the math class function called [Math]::Truncate
. It accepts an integer with a Double
data type, the decimal numbers.
Syntax:
[Math]::Truncate(variable)
variable
: This is the variable that holds the decimal number you want to truncate. It’s the input to theTruncate
method.
Example Code:
$decimalNum = 63.82
[Math]::Truncate($decimalNum)
In this code, we first assign the decimal number 63.82
to the variable $decimalNum
. Then, we use the [Math]::Truncate
function to truncate this decimal number.
Truncating means removing the decimal part and obtaining the nearest whole number, which in this case is 63
as shown in the output.
Output:
63
Rounding Down to Whole Numbers in PowerShell
The [Math]: Floor
is a PowerShell math class function that crops the integer’s decimal and rounds it down mathematically to a whole number in the backend.
Syntax:
[Math]::Floor(variable)
variable
: This is the variable that holds the numeric value you want to round down. It’s the input to theFloor
method.
Example Code:
$decimalNum = 63.82
[Math]::Floor($decimalNum)
In this code, we first assign the decimal number 63.82
to the variable $decimalNum
. Then, we use the [Math]::Floor
function to round down this decimal number to the nearest whole number, which is 63
.
Output:
63
Rounding Up to Whole Numbers in PowerShell
The [Math]::Ceiling
is a PowerShell math class function that rounds up the decimal number to the nearest whole number.
Syntax:
[Math]::Ceiling(variable)
variable
: This is the variable that holds the decimal number you want to round up. It’s the input to theCeiling
method.
Example Code:
$decimalNum = 63.82
[Math]::Ceiling($decimalNum)
In this code, we first assign the decimal number 63.82
to the variable $decimalNum
. Then, we use the [Math]::Ceiling
function to round up this decimal number to the nearest whole number, which is 64
.
Output:
64
Rounding Half Up (Arithmetic Rounding) in PowerShell
Arithmetic rounding is a common rounding method where numbers ending in 0.5 or higher are rounded up. PowerShell’s [Math]::Round
function can be used for arithmetic rounding without specifying a rounding mode.
Syntax:
[Math]::Round(variable)
variable
: This is the variable that holds the decimal number you want to round. It’s the input to theRound
method.
Example Code:
$decimalNum = 63.5
[Math]::Round($decimalNum)
In this code, we first assign the decimal number 63.5
to the variable $decimalNum
. Then, we use the [Math]::Round
function to round this decimal number to the nearest whole number.
When using this method without specifying a rounding mode, it follows the "Rounding Half Up"
rule, meaning numbers equal to or greater than 0.5
will round up to the nearest integer, which is 64
in this case.
Output:
64
Rounding in General in PowerShell
We can also use the official [Math]::Round
function to have more flexibility than rounding to a whole number.
The [Math]::Round
function accepts two arguments. The first argument is the decimal number that the function will round, and the second argument is the decimal place to which the number will be rounded (0
for the whole number, 1
for one decimal number, 2
for two decimal number, and so on).
Syntax:
[Math]::Round(par1, par2)
par1
: This is the first parameter that you pass to theRound
method. It represents the number that you want to round.par2
: This is the second parameter that you pass to theRound
method. It represents the number of decimal places to which you want to round the number specified inpar1
.
Example Code:
$decimalNum = 63.827439
[Math]::Round($decimalNum, 0)
[Math]::Round($decimalNum, 1)
[Math]::Round($decimalNum, 2)
[Math]::Round($decimalNum, 5)
In this code, we have a decimal number 63.827439
assigned to the variable $decimalNum
. We then use the [Math]::Round
function to perform multiple rounds with different numbers of decimal places.
Every time, the function rounds the decimal integer to the number of decimal places provided and outputs the corresponding value.
Output:
64
63.8
63.83
63.82744
Conclusion
In PowerShell, achieving precise number rounding is essential for accurate calculations. You can easily round numbers up and down or perform other rounding operations using the .NET framework’s Math class.
These functions provide the flexibility needed to tailor your calculations to your specific requirements, ensuring precision in your PowerShell scripts.
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn