How to Return Value in PowerShell
-
Using the
return
Keyword in PowerShell - Return Values in the Pipeline in PowerShell
- Defining Classes in PowerShell 5
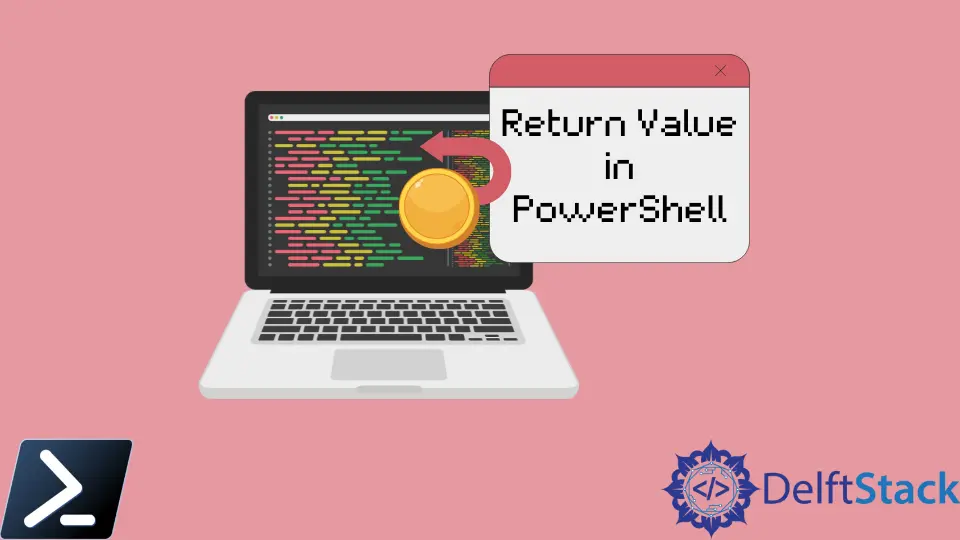
In general, the return
keyword exits a function, script, or script block. So, for example, we can use it to leave a scope at a specific point, return a value, or indicate that the scope’s end has been reached.
However, in Windows PowerShell, using the return
keyword can be a little bit confusing as your script may print an output that you may not have expected.
This article will discuss how the return
keyword works and how to properly use them in Windows PowerShell.
Using the return
Keyword in PowerShell
The script block below is the basic form of the syntax of the return
keyword.
return <expression>
The return
keyword can appear alone, or a value or expression can follow it. The return
keyword alone will return the command line to its previous calling point.
return
return $a
return (1 + $a)
The example below uses the return
keyword to exit a function if a conditional is met at a specific point. In this example, the odd numbers are not multiplied because the return statement goes before that statement can execute.
function MultiplyOnlyEven {
param($num)
if ($num % 2) { return "$num is not even" }
$num * 2
return
}
1..10 | ForEach-Object { MultiplyOnlyEven -Num $_ }
Output:
1 is not even
4
3 is not even
8
5 is not even
12
7 is not even
16
9 is not even
20
Windows PowerShell has confusing return semantics when viewed from a more native programming perspective. There are two main ideas that we need to consider:
- All output is captured and returned.
- The return keyword indicates a logical exit point.
With that being said, the following couple of script blocks will return the value of the $a
variable.
Return keyword with an expression:
$a = "Hello World"
return $a
Return keyword without an expression:
$a = "Hello World"
$a
return
The return
keyword is also not needed in the second script block, as calling the variable in the command-line will explicitly return that said variable.
Return Values in the Pipeline in PowerShell
When you return a value from your script block or function, Windows PowerShell automatically pops the members and pushes them one at a time through the pipeline. The reason behind this use case is due to Windows PowerShell’s one-at-a-time processing.
The following function demonstrates this idea will return an array of numbers.
function Test-Return {
$array = 1, 2, 3
return $array
}
Test-Return | Measure-Object | Select-Object Count
Output:
Count
-----
3
When using the Test-Return
cmdlet, the output from the function below is piped to the Measure-Object
cmdlet. The cmdlet will count the number of objects in the pipeline, and as executed, the returned count is three.
To make a script block or function return only a single object to the pipeline, use one of the following methods:
Utilizing Unary Array Expression in PowerShell
Utilizing a unary expression can send your return value down the pipeline as a single object, as demonstrated by the following example.
function Test-Return {
$array = 1, 2, 3
return (, $array)
}
Test-Return | Measure-Object | Select-Object Count
Output:
Count
-----
1
Use Write-Output
With NoEnumerate
Parameter in PowerShell
We can also use the Write-Output
cmdlet with the -NoEnumerate
parameter. The example below uses the Measure-Object
cmdlet to count the objects sent to the pipeline from the sample function by the return
keyword.
Example Code:
function Test-Return {
$array = 1, 2, 3
return Write-Output -NoEnumerate $array
}
Test-Return | Measure-Object | Select-Object Count
Output:
Count
-----
1
Another method of forcing the pipeline to return only a single object is introduced in PowerShell version 5, which we will discuss in the article’s next section.
Defining Classes in PowerShell 5
With Windows PowerShell version 5.0, we can now create and define our custom classes. Change your function into a class, and the return
keyword will only return the single object immediately preceding it.
Example Code:
class test_class {
[int]return_what() {
Write-Output "Hello, World!"
return 1000
}
}
$tc = New-Object -TypeName test_class
$tc.return_what()
Output:
1000
If the above class is a function, it will return all of the stored values in the pipeline.
Output:
Hello World!
1000
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn