How to Rename Files and Folders Using PowerShell
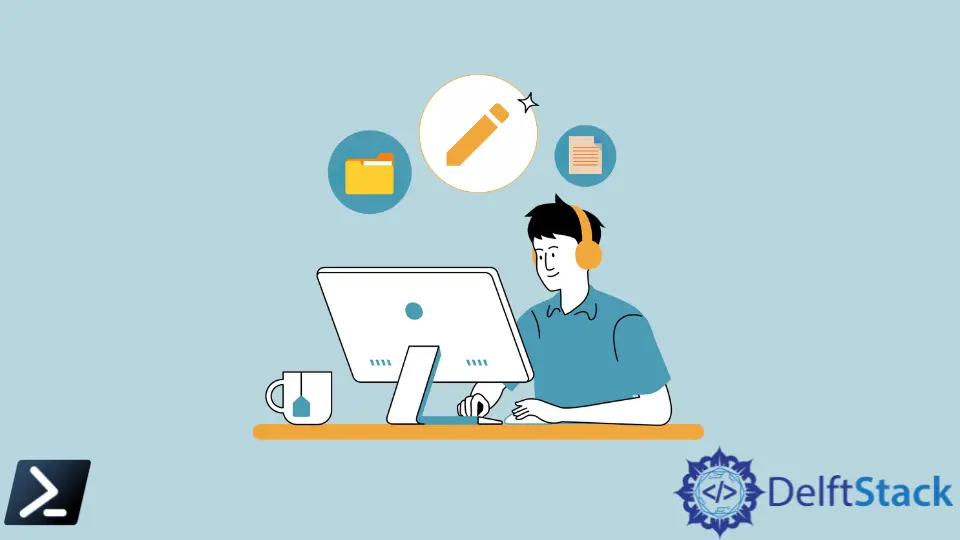
Renaming files and folders is most likely one of the basic functionalities of the Windows operating system. We can rename files and folders through the GUI by right-clicking on a file and selecting "Rename"
.
If we need multiple files to be renamed, it is more efficient to tackle it through automation. This article will discuss filtering files and folders and how to rename the file names and change the file extension using Windows PowerShell.
Filtering Files and Folders in PowerShell
The Windows PowerShell Get-ChildItem
command gets the operating system items in a specified directory or location. In addition, the location specified in the cmdlet can be a registry, file system, or certificate store.
We will focus our examples more on files and folders for this article.
Syntax:
Get-ChildItem -Path C:\PS\Temp
In the above command, the Get-ChildItem
cmdlet gets all items from the supplied value of the -Path
parameter. The Get-ChildItem
cmdlet when executed, display files, directories with their Mode
, LastWriteTime
, Length
(file size), and Name
properties on the PowerShell console.
Output:
Mode LastWriteTime Length Name
---- ------------- ------ ----
d----l 18/01/2022 8:52 pm Microsoft
d----l 20/12/2021 3:36 pm Cisco
-a---l 30/12/2021 3:24 pm (151) backup_phrase.txt
-a---l 17/06/2021 3:23 am (410049) CEF17501168.pdf
-a---l 16/05/2021 3:32 am (677) default.txt
-a---l 21/08/2020 9:06 am (2240) test.csv
-a---l 26/06/2021 8:25 am (63399) banner.jpg
-a---l 09/03/2021 10:48 pm (143) inventory.xlsx
<SNIP>
Using the -Filter
parameter, we can narrow our search results with just a single expression. We can also use the alias gci
as a substitute for the Get-ChildItem
command for a more seamless writing experience.
Example Code:
gci -Filter C:\PS\Temp\* -Filter *.xlsx
Output:
Mode LastWriteTime Length Name
---- ------------- ------ ----
-a---l 09/03/2021 10:48 pm (143) inventory.xlsx
<SNIP>
We can use the -Include
parameters, which accept multiple conditions. Therefore, we can say the -Include
parameter is better than the -Filter
parameter.
However, the -Include
parameter requires the -Path
parameter to be present in the expression.
Example Code:
gci -Path C:\PS\Temp\* -File -Include CEF*.pdf, *.xlsx, *.txt
Output:
Mode LastWriteTime Length Name
---- ------------- ------ ----
-a---l 17/06/2021 3:23 am (410049) CEF17501168.pdf
-a---l 16/05/2021 3:32 am (677) default.txt
-a---l 09/03/2021 10:48 pm (143) inventory.xlsx
<SNIP>
The path should include a wildcard asterisk (*
) at the end when using the’- Include’ switch parameter. This wildcard denotes that we are querying for all the child path items with the specific extension defined in the -Include
parameter.
Renaming Files in PowerShell
The Windows PowerShell rename command, Rename-Item
, works to rename the filenames of operating system items such as files and folders. For example, we will change the TestFile.txt
name to RenamedFile.txt
.
Example Code:
Rename-Item -Path '.\TestFile.txt' -NewName 'RenamedFile.txt'
gci -Filter 'RenamedFile.txt'
Now, if we will initiate a gci
command to filter a specific item, it should give us verification that the file has been renamed.
Output:
Mode LastWriteTime Length Name
---- ------------- ------ ----
-a---- 12/10/2021 11:38 PM 2982 RenamedFile.txt
The Rename-Item
cmdlet doesn’t only rename the filename of a specific file but can also change the format of files. For example, the snippet of code below uses the combination of Get-ChildItem
and Rename-Item
commands by filtering all files with .txt
format and changing their file format to .csv
.
Example Code:
gci *.txt | Rename-Item -NewName { $_.Name -replace '.txt', '.csv' }
gci -Filter *.csv
Output:
Mode LastWriteTime Length Name
---- ------------- ------ ----
-a---- 12/10/2021 11:38 PM 2982 RenamedFile.csv
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn