How to Set Default Value With Read-Host in PowerShell
-
Overview of the
Read-Host
Command in PowerShell -
Use a Conditional Statement to Set Default Value With the
Read-Host
Command in PowerShell -
Use a Function to Set Default Value With the
Read-Host
Command in PowerShell -
Use a Do-While Loop to Set Default Value With the
Read-Host
Command in PowerShell - Conclusion
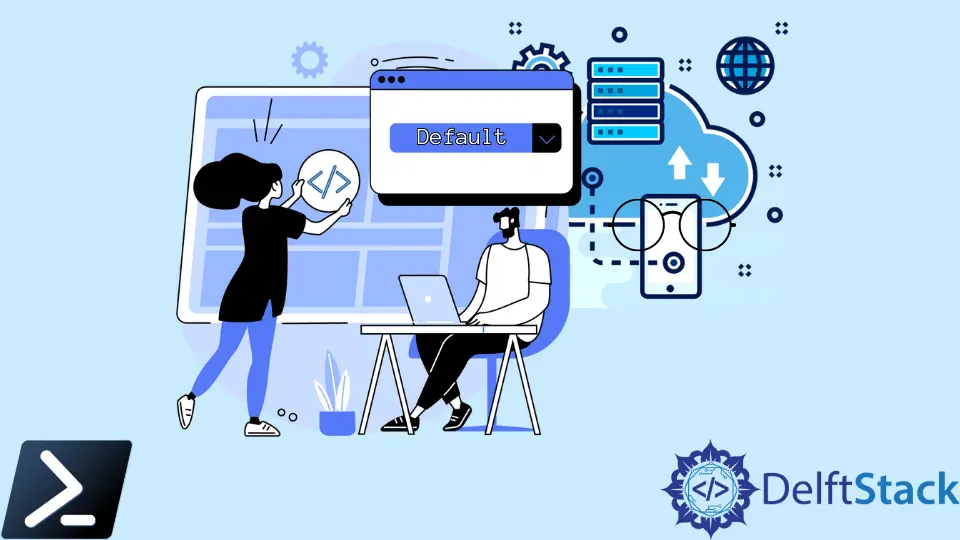
PowerShell is a powerful scripting language that provides various ways to interact with users. One common method is using Read-Host
to prompt users for input.
However, there are scenarios where providing a default value is beneficial. In this guide, we’ll explore different methods to incorporate default values with Read-Host
in PowerShell.
Overview of the Read-Host
Command in PowerShell
Read-Host
is a fundamental cmdlet in PowerShell that allows scripts to interact with users by prompting them for input. It is a versatile tool for gathering information during script execution. This cmdlet is particularly useful in scenarios where dynamic or user-specific information is needed.
Syntax:
Read-Host [-Prompt] <String> [-AsSecureString] [-Credential <PSCredential>] [-ErrorAction <ActionPreference>] [-Timeout <Int32>] [-OutVariable <String>] [-OutBuffer <Int32>] [-InputObject <PSObject>] [<CommonParameters>]
Here, the key parameters include:
-Prompt
: Specifies the message that will be displayed to the user.-AsSecureString
: Requests input as a secure string (password).-Credential
: Prompts for a username and password. Returns aPSCredential
object.-ErrorAction
: Defines the action to be taken if an error occurs.-Timeout
: Sets a time limit for how long the cmdlet will wait for input.-OutVariable
: Stores the result in a variable.-OutBuffer
: Determines the number of objects to buffer before outputting.-InputObject
: Allows input from the pipeline.
Basic Usage of the Read-Host
Command
$userInput = Read-Host "Please enter your name"
In this example, the user will be prompted with the message "Please enter your name"
. The value they enter will be stored in the variable $userInput
.
Secure String Input
$securePassword = Read-Host "Please enter your password" -AsSecureString
This command prompts the user for a password, and the input is stored as a secure string in the variable $securePassword
.
Timeout Functionality
$userInput = Read-Host "Please enter your choice" -Timeout 10
This command prompts the user for input and will wait for a maximum of 10
seconds. If no input is provided within that time, the variable $userInput
will be empty.
You can incorporate default values using conditional statements, functions, loops, or try-catch blocks in combination with Read-Host
.
Use a Conditional Statement to Set Default Value With the Read-Host
Command in PowerShell
Create a variable called defaultName
and give it the string value John Doe
. This value will be used in place of the user’s input if they choose not to provide one.
$defaultName = "John Doe"
Define a new variable, userName
, that will produce a message asking the user for input and will utilize the Read-Host
command to do this action.
$userName = Read-Host ("Please enter your name (default is {0})" -f $defaultName)
The code below implements a condition that checks to see whether the user has provided any input. It checks if the variable $userName
is null or an empty string.
If the user provides some input (not an empty string), it updates the value of $defaultName
to the user’s input.
if (-not $userName) { $userName = $defaultName }
Full code:
$defaultName = "John Doe"
$userName = Read-Host ("Please enter your name (default is {0})" -f $defaultName)
if (-not $userName) { $userName = $defaultName }
Write-Host "Hello, $($userName)!"
Output:
In the next approach, we relocate the process of receiving user input inside the condition that checks whether the user has entered any value or not. This allows us to better track whether or not the user has provided any data.
Without any action from the user, we use the default value, John Doe
. If the user offers input, however, we use the value saved in the userName
variable.
Code:
$defaultName = "John Doe"
if (($userName = Read-Host "Press enter to accept default value $defaultName") -eq '') { $defaultName } else { $userName }
Output:
The last approach verifies that the input is given from the user, and if it is, it then assigns the value of the user input straight to the variable named defaultName.
Code:
$defaultName = "John Doe"
if ($userName = Read-Host "Value [$defaultName]") { $defaultName = $userName }
Output:
This way, it does not have to use the else
condition.
Use a Function to Set Default Value With the Read-Host
Command in PowerShell
A function encapsulates a set of instructions that can be reused across scripts. We’ll design a function called Read-HostWithDefault
that incorporates default values.
function Read-HostWithDefault {
param (
[string]$prompt,
[string]$default
)
$userInput = Read-Host "$prompt (default is $default)"
$userInput = if ($userInput -ne "") { $userInput } else { $default }
return $userInput
}
Explanation:
function Read-HostWithDefault { ... }
: This defines the function namedRead-HostWithDefault
.param ( [string]$prompt, [string]$default )
: This specifies the parameters the function expects. In this case, it’s a prompt message and a default value.$userInput = Read-Host "$prompt (default is $default)"
: This line prompts the user for input, displaying both the prompt and default value. The input is stored in the variable$userInput
.$userInput = if ($userInput -ne "") { $userInput } else { $default }
: This line checks if$userInput
is not an empty string ($userInput -ne ""
). If this condition is true (meaning the user provided input),$userInput
retains its value. If false (meaning the user did not provide input),$default
is assigned to$userInput
.return $userInput
: Finally, the function returns the resulting value.
Now, let’s see how to use this function in a practical scenario:
$defaultName = "John Doe"
$name = Read-HostWithDefault -prompt "Enter your name" -default $defaultName
Write-Host "Hello, $name!"
Explanation:
$defaultName = "John Doe"
: Here, we set a default value for the user’s name.$name = Read-HostWithDefault -prompt "Enter your name" -default $defaultName
: This line prompts the user for their name, displaying the provided prompt message and default value. The functionRead-HostWithDefault
is called with the appropriate parameters.Write-Host "Hello, $name!"
: This generates a greeting that is either provided by the user or a default value.
Output:
Use a Do-While Loop to Set Default Value With the Read-Host
Command in PowerShell
The do-while
loop is a control structure in PowerShell that repeatedly executes a block of code as long as a specified condition is true. This is particularly useful when you want to ensure that a certain action is performed at least once.
The basic syntax of a do-while
loop is:
do {
# Code to be executed
} while (condition)
The block of code within the do
statement will continue to execute as long as the condition specified after the while
keyword is true.
Let’s consider an example where we want to prompt the user for their name but provide a default value of "John Doe"
if they don’t enter anything:
$defaultName = "John Doe"
do {
$userName = Read-Host "Please enter your name (default is $defaultName)"
$userName = if ($userName) { $userName } else { $defaultName }
} while (-not $userName)
Write-Host "Hello, $userName!"
Explanation:
$defaultName = "John Doe"
: This line sets the default value we want to use if the user doesn’t provide any input.do { ... } while (-not $userName)
: This initiates ado-while
loop. The block of code within thedo
statement will be executed at least once. The condition(-not $userName)
ensures the loop continues as long as$userName
is not provided.$userName = Read-Host "Please enter your name (default is $defaultName)"
: This prompts the user for their name, displaying the default value in the message.$userName = if ($userName) { $userName } else { $defaultName }
: This line uses anif-else
statement to determine the value of$userName
. If$userName
has a value (i.e., it’s not empty or null), it keeps that value. Otherwise, it assigns the default name$defaultName
.-not $userName
: This condition checks if$userName
is empty or not provided. If it’s empty, the loop will continue, prompting the user again.- Once the user provides input, the condition
-not $userName
becomes false, and the loop terminates.
Output:
Conclusion
In this comprehensive guide, we’ve explored various methods for setting default values with the Read-Host
command in PowerShell. Each approach offers distinct advantages depending on the specific requirements of your script.
- Conditional Statements: This method allows for a straightforward check for user input and provides flexibility in handling different scenarios.
- Functions: By encapsulating the logic in a function, you can create reusable code that incorporates default values seamlessly.
- Do-While Loops: This approach ensures that a certain action is performed at least once, making it suitable for scenarios where user input is crucial.
By understanding and applying these techniques, you can enhance the user experience and resilience of your PowerShell scripts. Remember to choose the method that aligns best with your specific script requirements.
I am Waqar having 5+ years of software engineering experience. I have been in the industry as a javascript web and mobile developer for 3 years working with multiple frameworks such as nodejs, react js, react native, Ionic, and angular js. After which I Switched to flutter mobile development. I have 2 years of experience building android and ios apps with flutter. For the backend, I have experience with rest APIs, Aws, and firebase. I have also written articles related to problem-solving and best practices in C, C++, Javascript, C#, and power shell.
LinkedIn