How to Run a PowerShell Script From Within the Python Program
-
Python
subprocess.Popen()
Method -
Run a PowerShell Script From Within the Python Program Using the
Popen()
Method
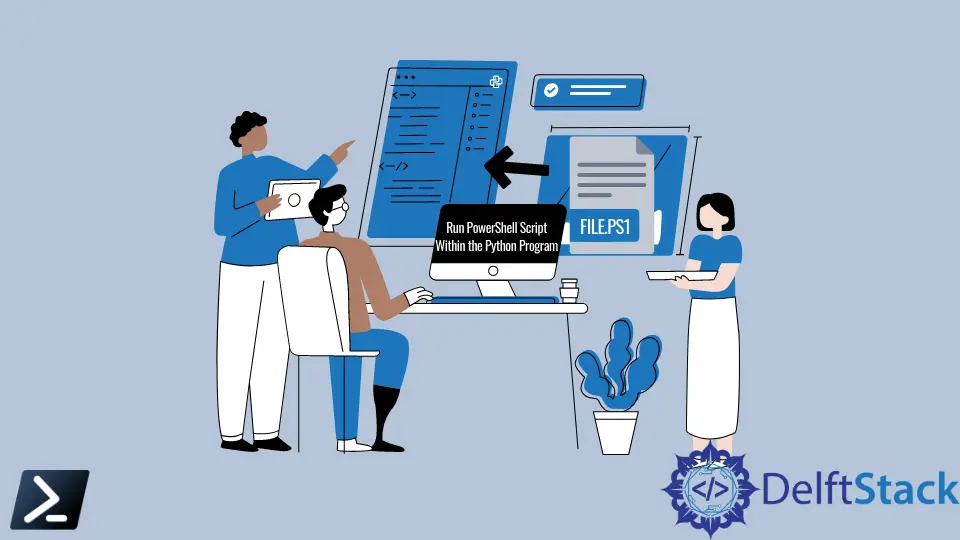
Windows PowerShell consists of tens of in-built cmdlets, which provide a rich set of features. Some of these features are unique and only available via PowerShell; hence, it is very useful if we can use PowerShell scripts within other programming languages like Python.
This article will focus on executing PowerShell logic from Python code.
Python subprocess.Popen()
Method
In Python, the external programs can be executed using the subprocess.Popen()
method. The subprocess
module consists of several more efficient methods than the ones available in the old os
module; hence, it is recommended to use the subprocess
module instead of the os
module.
Since we will run a PowerShell code from the Python program, the most convenient approach is to use the Popen
class in the subprocess
module. It creates a separate child process to execute an external program.
The one thing to keep in mind is that this process is platform-dependent.
Whenever you call subprocess.Popen()
, the Popen
constructor is called. It accepts multiple parameters, as shown in the following.
subprocess.Popen(args, bufsize=0, executable=None, stdin=None, stdout=None, stderr=None, preexec_fn=None, close_fds=False, shell=False, cwd=None, env=None, universal_newlines=False, startupinfo=None, creationflags=0)
The argument args
is used to pass the command that starts the external program. There are two ways that you can pass the command with its parameters.
-
As a Sequence of Arguments
Popen(["path_to_external_program_executable", "command_args1", "command_args2", ...])
-
As a Single Command String
Popen('path_to_external_program_executable -command_param1 arg1 -command_param2 arg2 ...')
The sequence of arguments is recommended with the Popen
constructor.
The next important argument is the stdout
parameter which we will be used in the next section. This specifies the standard output that the process should use.
Run a PowerShell Script From Within the Python Program Using the Popen()
Method
First, create a simple PowerShell script that prints to the console window.
Write-Host 'Hello, World!'
We will be saving it as sayhello.ps1
.
Next, we will be creating a Python script, runpsinshell.py
. Since we will use the subprocess.Popen()
command, we must import the subprocess
module first.
import subprocess