How to Prompt User Input in PowerShell
-
Use the
Read-Host
to Prompt for User Input in PowerShell -
Use the
Mandatory
Parameters to Prompt for User Input in PowerShell -
Use the
PromptForChoice
to Prompt for User Input in PowerShell - Conclusion
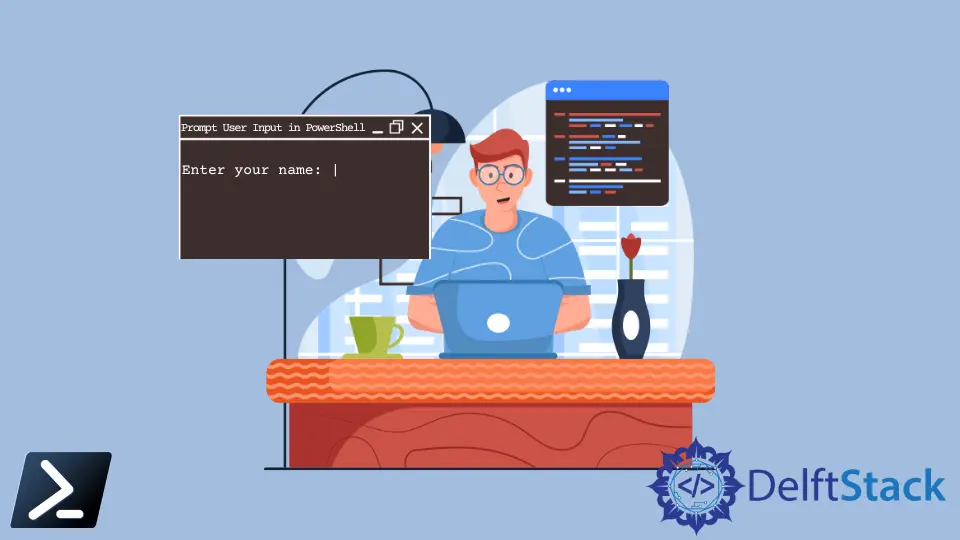
In PowerShell scripting, interacting with users to gather input is a common requirement. Whether it’s for customization, decision-making, or navigation, prompting users for input is essential.
This article explores various methods of prompting user input in PowerShell, including the Read-Host
method, the Mandatory
parameter method, and the PromptForChoice
method. Each method offers unique capabilities and is suited for different scenarios, enhancing the interactivity and usability of PowerShell scripts.
Use the Read-Host
to Prompt for User Input in PowerShell
The Read-Host
cmdlet prompts user input and reads a line of input from the console. The Read-Host
can accept only 1022
characters as input from a user.
The -Prompt
parameter is used to specify a text to provide the information about what to input. It appends a colon :
to the text you enter.
Example:
Read-Host -Prompt "Enter your name"
In this example, when we utilize Read-Host
, it prompts the user with the message "Enter your name"
and then pauses script execution, waiting for input. After the user enters their name and presses Enter, the script proceeds with execution.
However, since we’re not capturing or storing the input in a variable nor performing any subsequent actions based on the input received, this example primarily demonstrates the basic functionality of Read-Host
without further processing of the user input.
Output:
The Read-Host
pauses execution and receives input. When a user enters the value at the prompt, it returns that same value.
It does not accept input from the PowerShell pipeline. Here is another example:
$name = Read-Host "Enter your name"
Write-Output "Hello, $name!"
In this example, we use Read-Host
to prompt the user to enter their name. The string "Enter your name"
serves as the prompt message.
The user’s input is stored in the $name
variable. We then use Write-Output
to display a personalized greeting message, incorporating the value entered by the user.
With the Read-Host
cmdlet, we can save the input as a secure string, making it an invaluable tool for collecting sensitive information like passwords. This cmdlet enables us to prompt users for secure data while ensuring confidentiality and security.
Output:
The parameter -AsSecureString
displays asterisks *
in place of the characters that the user enters as input. With this parameter, the output of Read-Host
is a System.Security.SecureString
object.
Read-Host "Enter password" -AsSecureString
In this example, we use Read-Host
with the -AsSecureString
parameter to prompt the user to enter a password securely. The prompt message "Enter password"
is displayed to the user.
When the user enters the password, it is masked on the screen, ensuring confidentiality. The password is then stored as a secure string in the $password
variable, ready for further processing or validation.
Output:
Use the Mandatory
Parameters to Prompt for User Input in PowerShell
In PowerShell, the Mandatory
parameter method is a powerful way to prompt users for input while ensuring that certain parameters are required for script execution. By marking parameters as Mandatory
, script authors can enforce the provision of essential input values, thereby enhancing script reliability and usability.
Here is an example of the function Name
that asks for the user input when it is run.
Example:
function Name {
param(
[Parameter(Mandatory)]
[string]$name
)
Write-Output "Your name is $name."
}
Name
In this example, we define a PowerShell function called Name
with a single parameter named name
. By specifying [Parameter(Mandatory)]
, we ensure that the user must provide a value for the name
parameter when invoking the function.
The function then outputs a message incorporating the provided name using Write-Output
.
Output:
Use the PromptForChoice
to Prompt for User Input in PowerShell
In PowerShell, the PromptForChoice
method provides a structured way to prompt users with a set of options and receive their choice as input. This method is particularly useful when presenting users with a menu or list of choices, allowing for a more interactive and user-friendly script execution experience.
Example:
$choicePrompt = "Choose an option:"
$choices = [System.Management.Automation.Host.ChoiceDescription[]]@(
"&Option 1",
"&Option 2",
"&Option 3"
)
$defaultChoice = 0
$result = $host.UI.PromptForChoice("Title", $choicePrompt, $choices, $defaultChoice)
Write-Output "You selected $($choices[$result].Label)"
In this example, we define a prompt message and an array of choices and specify the default choice index. We then use the PromptForChoice
method to display the prompt dialog box with the provided choices.
The user’s selection is stored in the $result
variable, which we use to retrieve the chosen option label and display it to the user.
Output:
Conclusion
Prompting user input in PowerShell is crucial for creating interactive and user-friendly scripts. By utilizing methods such as Read-Host
, Mandatory
parameters, and PromptForChoice
, script authors can efficiently collect user input while ensuring reliability and security.
Whether it’s gathering simple text input, enforcing mandatory parameters, or presenting users with a structured set of choices, these methods empower script authors to create robust and versatile automation solutions. By understanding and leveraging these techniques, PowerShell script authors can enhance the usability and effectiveness of their scripts, catering to the diverse needs of users and administrators alike.