How to Output Informational Messages in PowerShell
- Introduction to Exporting Output in PowerShell
-
the
Write-Output
Cmdlet in PowerShell -
the
Write-Host
andWrite-Information
Cmdlet in PowerShell -
the
Write-Warning
Cmdlet in PowerShell -
the
Write-Debug
Cmdlet in PowerShell -
the
Write-Error
Cmdlet in PowerShell -
the
Write-Verbose
Cmdlet in PowerShell
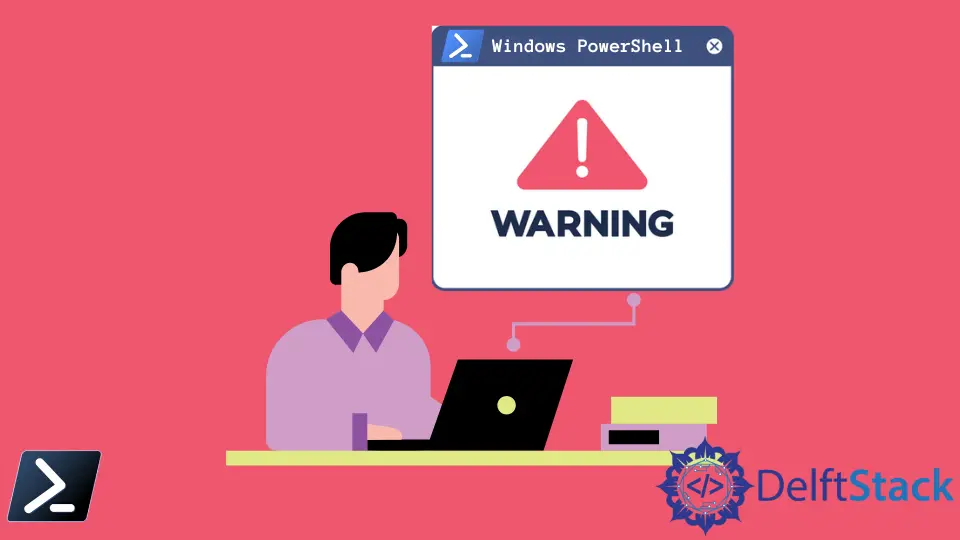
Writing informational messages to the console is an essential process in any language as it correctly gives feedback to the user. However, there are multiple ways of printing messages in Windows PowerShell.
This article will differentiate the multiple write commands such as writing informational, warning, and error messages and providing situations on when and where we can use them.
Introduction to Exporting Output in PowerShell
Before we start discussing the different write commands, it is worth noting that PowerShell can output a message by enclosing a single line with single (''
) or double quotation marks (""
).
Example Code:
"Hello World!"
'Hello World'
Output:
Hello World!
Hello World
This syntax is possible in PowerShell due to the string’s literal expression and the hidden string pipeline. The several snippets of code above are equivalent to the below example code.
Example Code:
"Hello World!" | Out-Host
The Out-Host
command sends the objects preceded for display on the console.
the Write-Output
Cmdlet in PowerShell
The first printing method is the Write-Output
command. This cmdlet is the most basic syntax of all printing methods inside the PowerShell scripting environment.
We can equate it to many different language’s basic printing methods such as print
and stdout
.
Example Code:
Write-Output "Hello World!"
Output:
Hello World!
the Write-Host
and Write-Information
Cmdlet in PowerShell
The Write-Host
command is another printing method similar to the previous way, Write-Output
.
The only difference of this command before it got updated in PowerShell 5.0 is that the method can only output messages with different colors using the parameters -BackgroundColor
and -ForegroundColor
.
As mentioned, starting in Windows PowerShell 5.0, Write-Host
is now a wrapper for Write-Information
. This allows us to use Write-Host
to emit output to the information stream.
This cmdlet enables the suppression or capture of data written using the Write-Host
command while preserving backward compatibility.
The $InformationPreference
preference variable and -InformationAction
standard parameter do not affect Write-Host
messages. The exception to this rule is
-InformationAction Ignore
, which effectively suppresses Write-Host
output.
Writing to the information stream using Write-Host
or Write-information
won’t create problems with your output string.
We can also manipulate the visibility of the information stream if we use the -InformationAction
parameter, provided we also bind the given parameter value to the Write-Host
statements in the function.
For instance, the example below will disable the information stream by default unless requested otherwise through the -InformationAction
parameter.
Write-Host 'This is a test message' -InformationAction $InformationPreference -ForegroundColor Green
the Write-Warning
Cmdlet in PowerShell
The Write-Warning
command writes a warning message to the Windows PowerShell host. The response to the warning event depends on the value of the user’s $WarningPreference
variable and the -WarningAction
standard parameter.
The valid values of the $WarningPreference
variable are as follows.
Stop
- Displays the warning and error messages and then stops executing.Inquire
- Displays the warning message and prompts the user for permission to continue in the form of a confirmation message.Continue
- The default value of the variable. Displays the warning message and then executes it.SilentlyContinue
- Doesn’t display the warning message. Continues executing.
Example Code:
Write-Warning "This is only a test warning notification." -WarningAction Continue
the Write-Debug
Cmdlet in PowerShell
The Write-Debug
command is also another method for printing in PowerShell. However, this is usually used more in development for printing debug messages in the scripting environment.
The debug messages are not displayed by default but can be changed using the $debugPreference
variable.
Example Code:
Write-Debug "Error on line 1. Please investigate."
$debugPreference = "Continue"
Write-Debug "Error on line 5. Please investigate."
Output:
DEBUG: Error on line 5. Please investigate.
the Write-Error
Cmdlet in PowerShell
The Write-Error
command outputs a non-terminating error. By default, the errors are sent in the error stream to the PowerShell host program to be exported along with output.
To output a non-terminating error, enter an error message string, an ErrorRecord
object, or an Exception
object. Then, use the different parameters of Write-Error
to populate the error record that we will export.
Non-terminating errors will write an error to the error stream, but they will not stop the command processing. For example, if a non-terminating error is thrown on one item in a set of input items, the cmdlet continues to process the other items.
Example Code:
Write-Error "Invalid object" -ErrorId B1 -TargetObject $_
Output:
Write-Error "Invalid object" -ErrorId B1 -TargetObject $_ : Invalid object
+ CategoryInfo : NotSpecified: (:) [Write-Error], WriteErrorException
+ FullyQualifiedErrorId : B1
the Write-Verbose
Cmdlet in PowerShell
The Write-Verbose
command writes text to the verbose message stream in PowerShell.
Like the Write-Debug
command, the verbose message is not displayed by default but can be displayed using the variable $VerbosePreference
or by adding the switch parameter -Verbose
.
Example Code:
Write-Verbose -Message "This will not be displayed."
Write-Verbose -Message "This will be displayed" -Verbose
Output:
VERBOSE: This will be displayed
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn