How to Create and Throw a New Exception in PowerShell
- Create a Custom Exception Class
-
Use the
throw
Statement to Throw a Custom Exception - Handle Custom Exceptions
- Conclusion
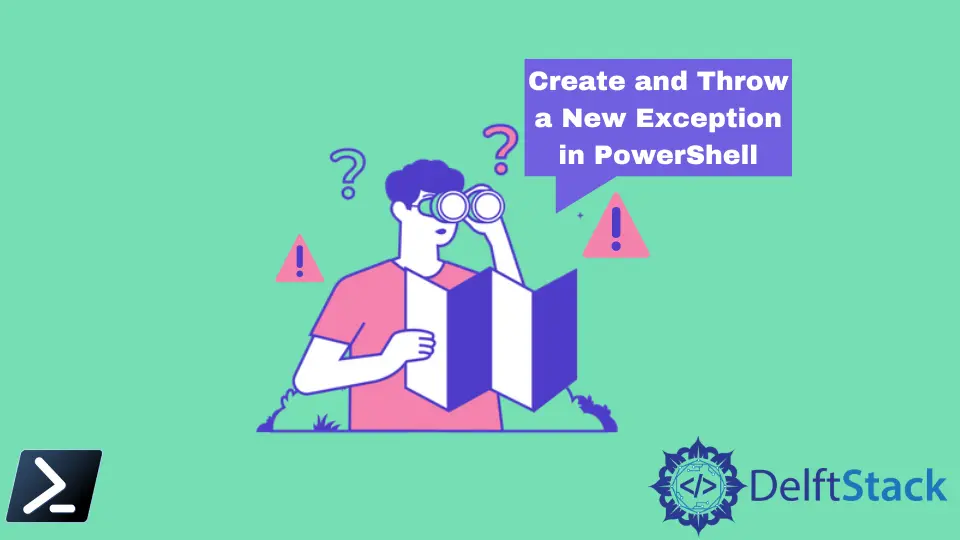
Exception handling is a critical aspect of writing robust and reliable PowerShell scripts. By creating and throwing exceptions, you can effectively communicate errors and issues that may occur during script execution.
While PowerShell offers several built-in exception types, there are scenarios where you need to create and throw your custom exception to tailor error handling to the specific needs of your script.
In this article, we will explore the process of creating and throwing custom exceptions in PowerShell, providing a detailed example and output.
Create a Custom Exception Class
Before diving into custom exceptions, let’s briefly review the concept of exceptions in programming.
An exception is an unexpected event or error that disrupts the normal flow of a program. When an exception occurs, control is transferred to a designated exception-handling code block, allowing for graceful error management.
PowerShell provides a variety of built-in exception types, such as System.Management.Automation.CmdletInvocationException
, System.IO.IOException
, and System.Exception
, to handle common errors. However, creating custom exceptions is beneficial when you encounter specific error cases or want to provide clear and informative error messages to users.
To create a custom exception in PowerShell, you start by defining a new exception class that inherits from the [System.Exception]
base class. You can then add properties and methods to customize the behavior of your exception.
Let’s illustrate this with a practical example:
class MyCustomException : [System.Exception] {
MyCustomException() {
}
MyCustomException([string] $message) {
$this.Message = $message
}
[string] GetMessage() {
return "Custom Exception: $($this.Message)"
}
}
In this example, we create a custom exception class named MyCustomException
. The MyCustomException
class inherits from the [System.Exception]
base class.
Then, two constructors are defined: one default constructor with no arguments and another constructor that accepts a custom error message.
Lastly, we include a GetMessage
method to return a formatted error message. This method can be useful for providing specific details when handling the exception.
Use the throw
Statement to Throw a Custom Exception
Once you have defined your custom exception class, you can throw it within your script using the throw
statement. To do this, create an instance of your custom exception and pass the relevant error message.
Here’s an example that demonstrates how to throw a custom exception:
try {
# Simulate code that may cause an error
$result = 1 / 0
}
catch {
$errorMessage = "An error occurred: $($_.Exception.Message)"
throw [MyCustomException]::new($errorMessage)
}
Within a try
block, we intentionally create an error by attempting to divide by zero.
In the catch
block, we capture the error, create a custom error message, and then throw a MyCustomException
instance containing this error message.
Handle Custom Exceptions
Handling custom exceptions is similar to handling built-in exceptions in PowerShell. You can use try
, catch
, and finally
blocks to gracefully manage errors.
Here’s an example that demonstrates catching and handling a custom exception:
try {
# Simulate code that may cause an error
$result = 1 / 0
}
catch [MyCustomException] {
Write-Host "Custom Exception Caught: $($_.Exception.Message)"
}
catch {
Write-Host "Other Exception Caught: $($_.Exception.Message)"
}
In this code, we attempt to divide by zero within the try
block. The first catch
block specifically captures the MyCustomException
and displays a message indicating that a custom exception was caught, along with the custom error message we provided.
If any other exception occurs, the second catch
block, which serves as a generic exception handler, catches it and displays a different message.
Let’s execute the script and see the output:
Custom Exception Caught: An error occurred: Attempted to divide by zero.
In this example, we intentionally trigger an error by attempting to divide by zero within the try
block. The catch
block for the MyCustomException
class catches the error and displays a message stating Custom Exception Caught
.
Additionally, it provides the custom error message: An error occurred: Attempted to divide by zero
. If any other exceptions occurred, they would be caught by the generic catch
block, but that is not applicable in this example.
Here’s the complete working PowerShell code for creating and throwing a custom exception, along with an example of how to handle it:
class MyCustomException : [System.Exception] {
MyCustomException() {
}
MyCustomException([string] $message) {
$this.Message = $message
}
[string] GetMessage() {
return "Custom Exception: $($this.Message)"
}
}
try {
# Simulate code that may cause an error (division by zero)
$result = 1 / 0
}
catch {
$errorMessage = "An error occurred: $($_.Exception.Message)"
throw [MyCustomException]::new($errorMessage)
}
try {
# Simulate code that may cause another error (e.g., accessing a non-existent variable)
$nonExistentVariable = $undefinedVariable
}
catch [MyCustomException] {
Write-Host "Custom Exception Caught: $($_.Exception.Message)"
}
catch {
Write-Host "Other Exception Caught: $($_.Exception.Message)"
}
This code will output the result of the exception handling, distinguishing between the custom exception and other exceptions.
Conclusion
Creating and throwing custom exceptions in PowerShell allows you to provide more informative error messages and enhances the reliability and maintainability of your scripts. These custom exceptions help you tailor error handling to your specific script requirements, ensuring a smoother user experience and improved troubleshooting capabilities.