How to Get Exit Code From Start-Process in PowerShell
-
Get the Exit Code From the
Start-Process
Command in PowerShell Using the-Wait
Parameter -
Get the Exit Code From the
Start-Process
Command in PowerShell by Checking the$LastExitCode
Variable -
Get the Exit Code From the
Start-Process
Command in PowerShell Using a Process Object - Conclusion
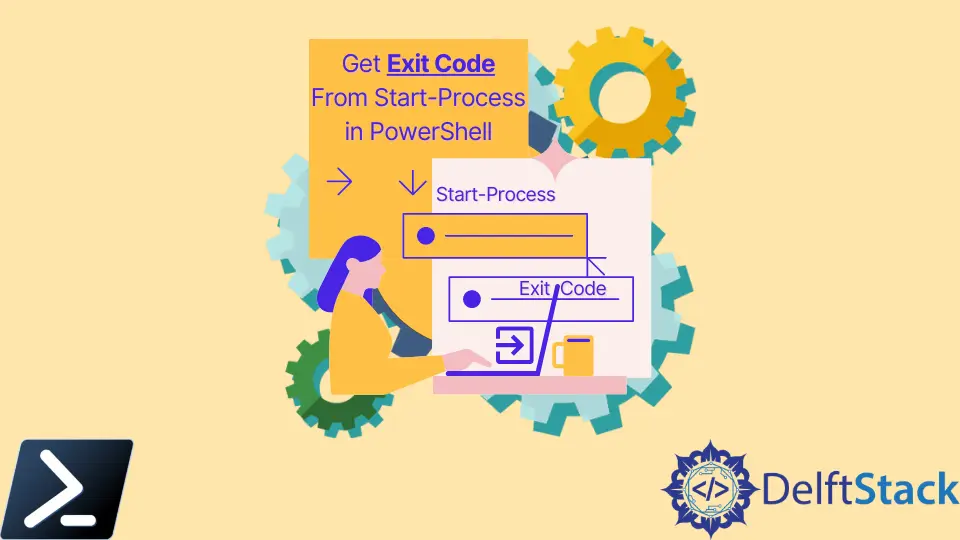
In PowerShell, the Start-Process
cmdlet is used to launch new processes, but obtaining the exit code of a process started with this command is not straightforward. The exit code represents the status of the process after it has been completed, and it can be valuable for scripting purposes or for determining if the process was successful.
In this article, we will explore ways to obtain the exit code from a process initiated using Start-Process
in PowerShell.
Get the Exit Code From the Start-Process
Command in PowerShell Using the -Wait
Parameter
Before diving into how to retrieve the exit code using the -Wait
parameter, let’s briefly discuss what exit codes are. An exit code is a numerical value that a process returns upon completion.
Conventionally, an exit code of 0
indicates successful execution, while non-zero exit codes represent errors or specific conditions defined by the executed application or script.
The -Wait
parameter in the Start-Process
cmdlet instructs PowerShell to wait for the started process to complete before continuing with the script. This is crucial for obtaining the exit code of the process.
Let’s explore a step-by-step approach to achieve this:
-
To start a process and wait for it to complete using the
-Wait
parameter, use the following syntax:Start-Process -FilePath "YourExecutable.exe" -ArgumentList "YourArguments" -NoNewWindow -Wait
Replace
"YourExecutable.exe"
with the path to the executable or script you want to run and"YourArguments"
with any arguments the process requires. -
To capture the process and its exit code, store the
Start-Process
command in a variable:$process = Start-Process -FilePath "YourExecutable.exe" -ArgumentList "YourArguments" -NoNewWindow -Wait -PassThru
The
-PassThru
parameter allows the process object to be saved in the variable$process
. -
Once the process is completed, you can access its exit code using the
ExitCode
property of the process object stored in the variable:$exitCode = $process.ExitCode Write-Host "Exit code: $exitCode"
The
Write-Host
cmdlet is used to display the obtained exit code in the console.
By employing the -Wait
parameter and accessing the ExitCode
property, you can successfully retrieve the exit code of a process initiated using Start-Process
in PowerShell.
Here’s an example script that illustrates the steps mentioned above:
# Start the process and wait for it to complete
$process = Start-Process -FilePath "notepad.exe" -NoNewWindow -Wait -PassThru
# Retrieve the exit code
$exitCode = $process.ExitCode
Write-Host "Exit code: $exitCode"
In this example, we start the Notepad application, wait for it to complete, and then obtain and display the exit code.
Get the Exit Code From the Start-Process
Command in PowerShell by Checking the $LastExitCode
Variable
PowerShell automatically stores the exit code of the most recently executed process in the $LastExitCode
variable. This variable can be accessed after a process is started using Start-Process
.
Here’s a step-by-step approach to utilizing $LastExitCode
:
-
Begin by starting the desired process using the
Start-Process
cmdlet. Here’s a generic syntax:Start-Process -FilePath "YourExecutable.exe" -ArgumentList "YourArguments" -NoNewWindow
Replace
"YourExecutable.exe"
with the path to the executable or script you want to run and"YourArguments"
with any arguments the process requires. -
After the process completes, access the exit code using the
$LastExitCode
variable:$exitCode = $LastExitCode Write-Host "Exit code: $exitCode"
The
Write-Host
cmdlet is used to display the exit code in the console.
By leveraging the $LastExitCode
variable, you can effortlessly retrieve the exit code of a process initiated using Start-Process
in PowerShell.
Below is a comprehensive example script that illustrates the steps above:
# Start the process
Start-Process -FilePath "notepad.exe" -NoNewWindow
# Access the exit code
$exitCode = $LastExitCode
Write-Host "Exit code: $exitCode"
In this example, we start the Notepad application and then access and display the exit code using $LastExitCode
.
Get the Exit Code From the Start-Process
Command in PowerShell Using a Process Object
When using the -PassThru
parameter with Start-Process
, the cmdlet returns a System.Diagnostics.Process
object representing the newly started process. You can then access the exit code using the ExitCode
property of this object.
Here’s a step-by-step approach to achieving this:
-
Start the desired process and capture the process object using the
-PassThru
parameter:$process = Start-Process -FilePath "YourExecutable.exe" -ArgumentList "YourArguments" -NoNewWindow -PassThru
Replace
"YourExecutable.exe"
with the path to the executable or script you want to run and"YourArguments"
with any arguments the process requires. -
If needed, wait for the process to complete using the
WaitForExit()
method:$process.WaitForExit()
This step ensures that the script waits for the process to finish before proceeding.
-
Once the process is completed, access its exit code using the
ExitCode
property:$exitCode = $process.ExitCode Write-Host "Exit code: $exitCode"
The
Write-Host
cmdlet is used to display the exit code in the console.
By leveraging a process object and accessing the ExitCode
property, you can successfully retrieve the exit code of a process initiated using Start-Process
in PowerShell.
Here’s a comprehensive example script that illustrates the steps mentioned above:
# Start the process and obtain a process object
$process = Start-Process -FilePath "notepad.exe" -NoNewWindow -PassThru
# Wait for the process to complete
$process.WaitForExit()
# Access the exit code
$exitCode = $process.ExitCode
Write-Host "Exit code: $exitCode"
In this example, we start the Notepad application, wait for it to complete, and then obtain and display the exit code using the process object.
Conclusion
Obtaining the exit code from a process started with the Start-Process
cmdlet in PowerShell can be achieved using the -Wait
parameter, the $LastExitCode
variable, or by accessing the ExitCode
property of the System.Diagnostics.Process
object.
Understanding how to retrieve the exit code allows you to programmatically handle and respond to the outcomes of processes, enhancing the automation and reliability of your PowerShell scripts.
John is a Git and PowerShell geek. He uses his expertise in the version control system to help businesses manage their source code. According to him, Shell scripting is the number one choice for automating the management of systems.
LinkedIn