How to Upload File to SFTP With PowerShell
- What is SFTP
- Upload a File to an SFTP Site With PowerShell
-
Use
WinSCP
to Upload Files to SFTP Site With PowerShell
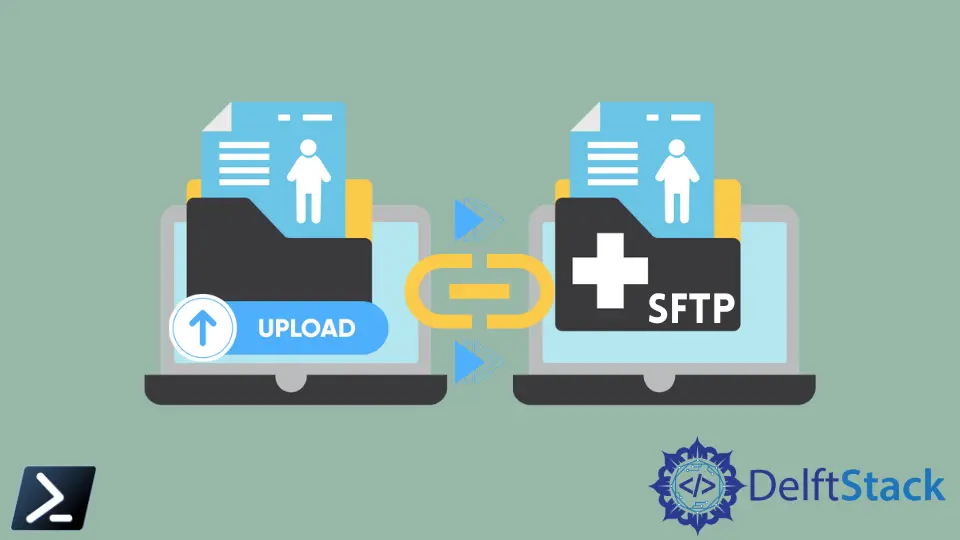
Windows and Mac users find tools that can be run through Command-Line Interfaces very limitedly. Most GUI-based applications provide low scripting and automation capabilities to all users.
PowerShell is used to bridge this gap between Windows users and users of Linux-like systems. PowerShell offers a Command-line interface for scripting purposes, automation requirements, CI/CD systems management, etc.
PowerShell is a product by Microsoft and provides flexibility to Windows and Mac users in fulfilling their scripting requirements. However, in using PowerShell, there are scripting commands that you should be fluent in and commands that are unique to PowerShell.
What is SFTP
SFTP is a protocol that users commonly use to transmit files from one computer/server to another computer/server. It is a secure version of the common FTP protocol, ensuring end-to-end file encryption between sender and receiver.
SFTP stands for Secure File Transfer Protocol and is one of the most frequently used file transfer methods in computing.
Upload a File to an SFTP Site With PowerShell
Our requirement here would be to do a scheduled upload of a file to an SFTP site with PowerShell. PowerShell lets users run scheduled scripts without any issue.
This can be explained in a few steps.
- A file will be scheduled to upload from a database to an SFTP site.
- An authentication is established to access the site.
- Windows Task Scheduler triggers a script to run at a specific time.
- The required file would be grabbed and uploaded to the FTP site by the task scheduler.
Use WinSCP
to Upload Files to SFTP Site With PowerShell
WinSCP
is one of the most popular SFTP clients and an FTP client for Microsoft Windows. This tool can copy files from a computer to a remote file server.
We have to first download WinSCP .NET
assembly for this requirement.
Then the file has to be extracted with the script. Then we will create a script as follows based on the official WinSCP
upload example here.
Add-Type -Path "WinSCPnet.dll"
$sessionOptions = New-Object WinSCP.SessionOptions -Property @{
Protocol = [WinSCP.Protocol]::Sftp
HostName = "example.com"
UserName = "user"
Password = "mypassword"
SshHostKeyFingerprint = "ssh-rsa 2048 xx...="
}
$session = New-Object WinSCP.Session
try {
$session.Open($sessionOptions)
$session.PutFiles("C:\users\export.txt", "/Outbox/").Check()
}
finally {
$session.Dispose()
}
It is important to remember that all these must be done in the WinSCP
GUI, as shown below.
- Logging to
WinSCP
. - Navigate to the target directory of the remote location.
- Choose
upload command
. Transfer Options
>Transfer Settings
>Generate code
>.NET Assembly Code
>PowerShell Language
.
As the output of the code, the file would be uploaded to the place/site you specify and finally cleaned up to dispose of the session.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.