How to Run Exe File With Parameters in PowerShell
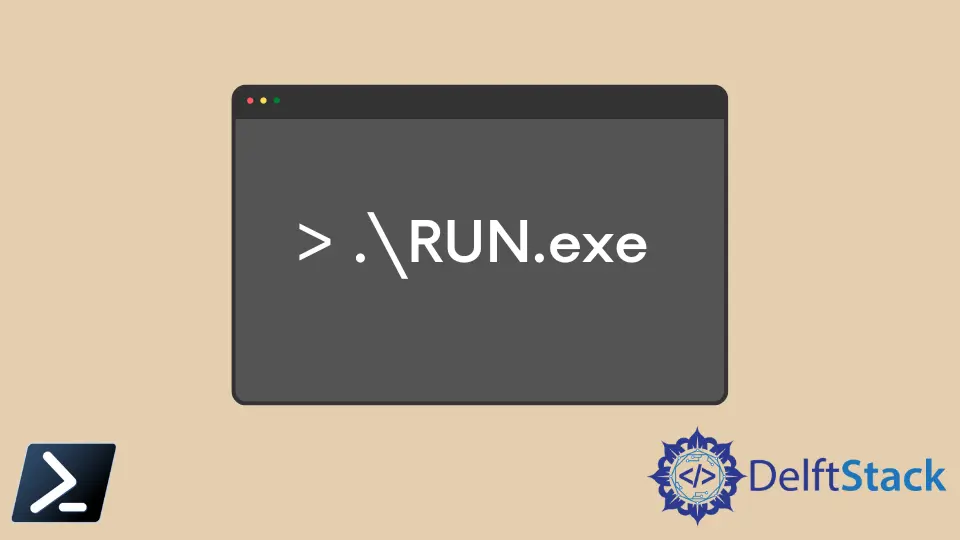
The exe
file type contains a program/application that can be executed in a Windows environment. The extension EXE
is the short form for executable.
When you double click on a .exe
file, it will run some program/application. The same .exe
file can be executed via Windows PowerShell too.
This article only focuses on running exe
files with parameters because the normal exe
file execution (without parameters) is quite straightforward if it is already in the Windows PATH
.
Methods to Run exe
File in PowerShell
There are two scenarios to address here.
- The
exe
file path is already in the WindowsPATH
, and the command name doesn’t contain any spaces. - The
exe
file path is not in the WindowsPATH
, and the command name or parameters contain spaces.
It is quite straightforward to call the exe
file with parameters/arguments for the first scenario. The syntax looks like the following.
<abc.exe> [/parameter1 /parameter2...]
OR
<abc.exe> [-host "host.org" -u "username" -p "password"]
abc.exe
- executable name/parameter1 /parameter2
- Any number of parameters can be included with/
and separated by a space.-host "host.org" -u "username" -p "password"
- Named parameters can be passed like this.
Example:
yourexecutable.exe /parameter1 /parameter2
OR
yourexecutable.exe -host "host.org" -u "username" -p "password"
Meanwhile, the exe
file path is not in the Windows PATH
in the second scenario. Therefore, you should explicitly give the full path to the exe
file/command.
If the path contains spaces, you must wrap it within the quotes (as shown below).
'C:\Program Files (x86)\Windows Media Player\wmplayer.exe'
When PowerShell sees this type of command starting with a string, it evaluates the command as a string and echos to the command window.
C:\Program Files (x86)\Windows Media Player\wmplayer.exe
This is not the intended output. Therefore, for PowerShell to interpret this string as a command name, you need to use the special operator called call operator (&
). We call this an invocation operator as well.
the Call Operator (&
) in PowerShell
The call operator (&
) can be added just before the command name. It will make PowerShell interpret the string next to the call operator (&
) as a command name.
& 'C:\Program Files (x86)\Windows Media Player\wmplayer.exe'
This will open up the Windows Media Player successfully.
Pass Parameters With Call Operator (&
) in PowerShell
You can easily pass the parameters/arguments to the command with the call operator (&
).
& 'C:\Program Files (x86)\Windows Media Player\wmplayer.exe' "D:\music videos\video.mp4" /fullscreen
In the above command:
'C:\Program Files (x86)\Windows Media Player\wmplayer.exe'
is the command name."D:\videos\funny videos\ video.avi"
is the first parameter passed to the above command./fullscreen
- Another argument passed to the command.
There are cleaner ways to execute this type of command when you need to pass several parameters/arguments.
Method 1:
It is cleaner and maintainable to assign each argument/parameter to a variable and reuse it.
$cmd = 'C:\Program Files (x86)\Windows Media Player\wmplayer.exe'
$arg1 = 'D:\music videos\video.mp4'
& $cmd $arg1 /fullscreen
Method 2:
There is another way of passing parameters/arguments to a command as a unit, and it is called splatting.
$cmd = 'C:\Program Files (x86)\Windows Media Player\wmplayer.exe'
$allargs = @('D:\music videos\video.mp4', 'anotherargument', 'nextargument')
& $cmd $allargs /fullscreen
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.