How to Run PowerShell Script as Administrator
-
Using the
Start-Process
Cmdlet to Run PowerShell Script as Administrator - Run PowerShell Script With Arguments as Administrator
- Run PowerShell Script as Administrator While Preserving the Working Directory
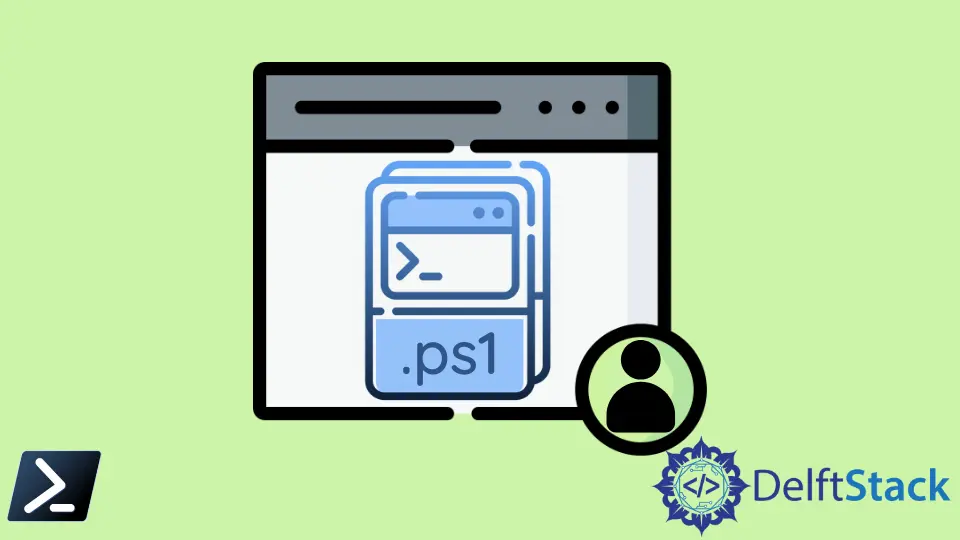
Most scripts that we write and execute will require permissions, leading to an administrator running the scripts from an elevated PowerShell prompt. In PowerShell, we elevate our permissions by running Windows PowerShell with administrator privileges and entering our administrator credentials.
However, we may need to run our scripts seamlessly for specific situations without keying in administrator credentials. Therefore, we can integrate various self-elevating commands at the beginning of our scripts, and we will show you how in this article.
Using the Start-Process
Cmdlet to Run PowerShell Script as Administrator
The Start-Process
cmdlet initiates one or more processes, executable or script files, or any files that an installed software can open on the local computer, including Windows PowerShell.
With this cmdlet and a set of parameters, we can run Windows PowerShell as an administrator.
For this example, we have created a Hello_World.ps1 script, which will output a simple Hello World
string inside our console.
Hello_World.ps1
:
Write-Output 'Hello World!'
We can use the PowerShell file above as an example to check and verify if we are running our scripts in administrator privileges. To start, run the snippet code below.
Start-Process powershell -Verb RunAs -ArgumentList ".\Hello_World.ps1"
Upon running the script above, it will spawn a new instance of Windows PowerShell with administrative rights. The only caveat for the script run above is that if we need to pass arguments to our PowerShell file, we will not carry the arguments over to the newly spawned administrative console.
Run PowerShell Script With Arguments as Administrator
This example can take our previous one-liner script and revise it inside multiple conditional statements.
# Self-elevate the script if required
if (-Not ([Security.Principal.WindowsPrincipal] [Security.Principal.WindowsIdentity]::GetCurrent()).IsInRole([Security.Principal.WindowsBuiltInRole] 'Administrator')) {
if ([int](Get-CimInstance -Class Win32_OperatingSystem | Select-Object -ExpandProperty BuildNumber) -ge 6000) {
$Command = "-File `"" + $MyInvocation.MyCommand.Path + "`" " + $MyInvocation.UnboundArguments
Start-Process -FilePath PowerShell.exe -Verb RunAs -ArgumentList $Command
Exit
}
}
# Place your script here
Write-Output 'Hello World!'
Previously, we ran our PowerShell script by calling a separate file, but we can simply place our script (eg. Hello_World.ps1) below this snippet for this example.
Here’s how the snippet works.
- The first
if
statement checks if the executed script is already running in Windows PowerShell with administrative privileges. - The second
if
statement checks if the Windows Operating System build number is 6000 or greater. (Windows Vista or Windows Server 2008, or later) - The
$Command
variable retrieves and saves the command used to run the script, including arguments. - The
Start-Process
starts a new instance of Windows PowerShell with elevated privileges and reruns the script just like our previous script.
Run PowerShell Script as Administrator While Preserving the Working Directory
We may need to preserve the script’s working directory for specific situations. So, here’s a self-elevating snippet that will maintain the working directory:
if (-Not ([Security.Principal.WindowsPrincipal][Security.Principal.WindowsIdentity]::GetCurrent()).IsInRole([Security.Principal.WindowsBuiltInRole]::Administrator)) {
if ([int](Get-CimInstance -Class Win32_OperatingSystem | Select-Object -ExpandProperty BuildNumber) -ge 6000) {
Start-Process PowerShell -Verb RunAs -ArgumentList "-NoProfile -ExecutionPolicy Bypass -Command `"cd '$pwd'; & '$PSCommandPath';`"";
Exit;
}
}
# Place your script here
Write-Output 'Hello World!'
In this snippet, we passed $PSCommandPath
as one of the arguments inside the Start-Process
cmdlet to preserve the working directory on where the script has been executed.
Preserving the working directory is vital for performing path-relative operations. Unfortunately, the previous couple of snippets that we showed earlier will not maintain their path, which can cause unexpected errors. Therefore you can use the revised syntax above.
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn