How to Set Environment Variables Using PowerShell
- What Are Environment Variables
-
Set an Environment Variable With
Env:
in PowerShell -
Set the
[System.Environment]
.NET Class in PowerShell - Refresh Environment Variables on Current PowerShell Session
- Conclusion
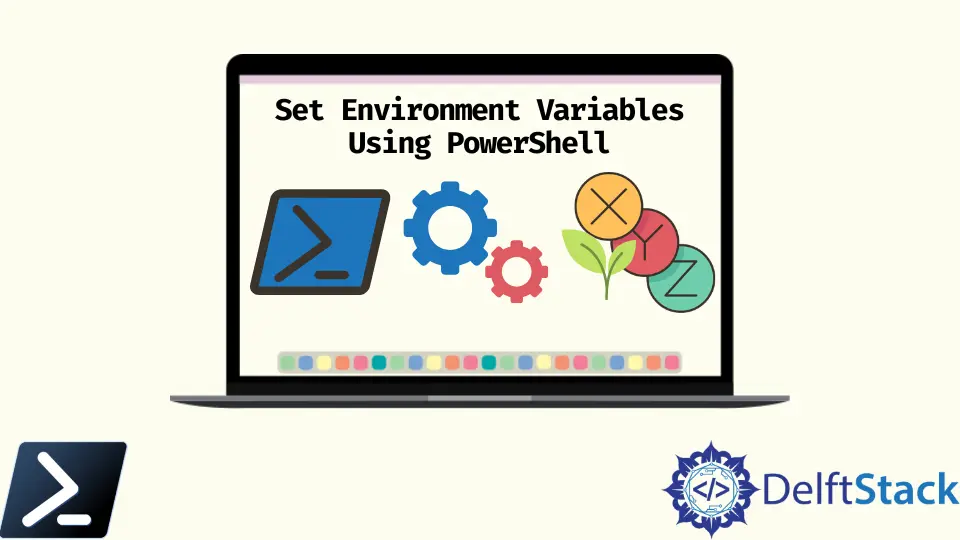
Using Windows PowerShell to set Windows environment variables, read environment variables, and create new environment variables is easy once we know the proper execution of commands.
PowerShell provides many different methods to interact with Windows environment variables from the $env:
PSDrive and the [System.Environment]
.NET class. This article will discuss setting environment variables and refreshing them in our current session using PowerShell.
What Are Environment Variables
As the name suggests, environment variables store information about Windows and applications’ environments.
We can access environment variables through a graphical interface such as Windows Explorer and plain text editors like Notepad
, cmd.exe
, and PowerShell.
Using environment variables helps us avoid hard-coding file paths, user or computer names, and more in our PowerShell scripts or modules.
Set an Environment Variable With Env:
in PowerShell
We can create new environment variables with PowerShell using the New-Item
cmdlet. But, first, provide the environment variable’s name in the Env:\<EnvVarName>
format for the Value
parameter, as shown below.
Example Code:
New-Item -Path Env:\TEST -Value WIN10-DESKTOP
We use the PowerShell cmdlet New-Item
to create or update an environment variable named TEST
within the Env:\
namespace. By specifying -Value WIN10-DESKTOP
, we assign the value WIN10-DESKTOP
to this variable.
This action impacts the current PowerShell session, making the TEST
environment variable accessible to other scripts and commands within the session.
Output:
We can use the Set-Item
cmdlet to set an environment variable or create a new one if it doesn’t already exist. For example, we can see below using the Set-Item
cmdlet.
We can both make or modify an environment variable.
Example Code:
Set-Item -Path Env:TEST -Value "TestValue"
We use the PowerShell cmdlet Set-Item
to modify the value of an existing environment variable named TEST
within the Env:\
namespace. By specifying -Value "TestValue"
, we assign the string "TestValue"
to the TEST
environment variable.
This action directly affects the current PowerShell session, updating the value of TEST
for use by other scripts and commands within the session.
Output:
Set the [System.Environment]
.NET Class in PowerShell
The [System.Environment]
will use a few different .NET static class methods. We don’t need to understand what a static way is.
We only need to understand how to use any of the techniques we are about to learn, and we will need first to reference the class ([System.Environment]
) followed by two colons (::
) then followed by the method.
To set the environment variable using the .NET class stated, use the SetEnvironmentVariable()
function to set the value of an existing environment variable for the given scope or create a new environment variable if it does not already exist.
When setting variables in the process scope, we will find that the process scope is volatile and existing in the current session, while changes to the user and machine scopes are permanent.
Example Code:
[System.Environment]::SetEnvironmentVariable('TestVariable', 'TestValue', 'User')
We use the .NET method [System.Environment]::SetEnvironmentVariable()
to set an environment variable named TestVariable
with the value 'TestValue'
in the user-specific environment. By specifying 'User'
as the third parameter, we ensure that this variable is set within the user’s environment context.
This action impacts the environment settings for the current user, allowing other scripts and applications to access the TestVariable
with its assigned value.
Refresh Environment Variables on Current PowerShell Session
To use our new set of environment variables in our PowerShell session, get the environment variable of the user profile and machine through the .NET class and assign it to the PowerShell environment variable.
Since environment variables are also considered PowerShell variables, we can assign values to them directly more straightforwardly.
Example Code:
$env:PATH = [System.Environment]::GetEnvironmentVariable("Path", "Machine") + ";" + [System.Environment]::GetEnvironmentVariable("Path", "User")
We are updating the $env:PATH
environment variable by concatenating the values of the system and user Path
environment variables retrieved using [System.Environment]::GetEnvironmentVariable()
. We first retrieve the system Path
variable using the "Machine"
parameter and then append the user Path
variable retrieved using the "User"
parameter.
By combining these values with a semicolon separator, we ensure that both sets of paths are included in the updated $env:PATH
.
Output:
Conclusion
In this article, we explored various methods for managing environment variables in PowerShell, focusing on setting and refreshing them using different techniques. We began by demonstrating how to create or modify environment variables using PowerShell cmdlets like New-Item
and Set-Item
, providing clear examples and explanations for each step.
Next, we delved into the use of the [System.Environment]
.NET class to manipulate environment variables. Through the SetEnvironmentVariable()
method, we learned how to set variables with specific scopes, understanding the implications of each scope on variable persistence.
Lastly, we covered the importance of refreshing environment variables within the current PowerShell session, emphasizing the use of [System.Environment]::GetEnvironmentVariable()
to update variables dynamically.
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn