How to Prevent Idle Mode in PowerShell
- Understanding Idle Mode
- Method 1: Using PowerShell to Change Power Settings
- Method 2: Prevent Idle Mode Using PowerShell Script
- Method 3: Using PowerShell to Create a Scheduled Task
- Conclusion
- FAQ
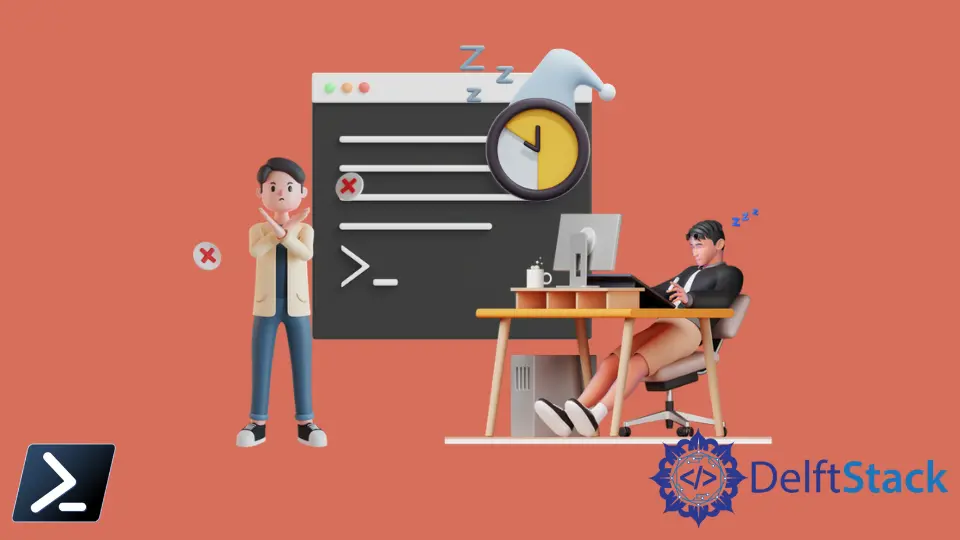
In today’s fast-paced digital world, maintaining productivity is essential. One common issue that users face is their systems entering idle mode, which can disrupt workflows and lead to frustration. Thankfully, PowerShell provides various methods to prevent idle mode, allowing you to keep your system active while working on critical tasks.
In this tutorial, we will explore different techniques to prevent idle mode using PowerShell commands. Whether you’re a seasoned developer or a casual user, these methods will help you stay focused without interruptions. Let’s dive into the world of PowerShell and discover how to keep your system awake!
Understanding Idle Mode
Before we explore the methods to prevent idle mode, it’s essential to understand what idle mode is. Idle mode occurs when a computer system remains inactive for a certain period, leading to energy-saving features kicking in, such as screen dimming or entering sleep mode. This feature is beneficial for conserving energy but can be detrimental when you’re in the middle of critical tasks. PowerShell, a powerful command-line tool, can help you manage your system’s power settings effectively.
Method 1: Using PowerShell to Change Power Settings
One of the most straightforward methods to prevent idle mode is by changing the power settings through PowerShell. You can adjust the power plan settings to prevent the computer from going to sleep. Here’s how to do it:
powercfg -change -standby-timeout-ac 0
This command sets the standby timeout to 0 when connected to AC power, effectively preventing the system from entering idle mode.
By executing this command, you instruct your system not to enter standby mode when it’s plugged in. It’s a simple yet effective way to ensure that your work isn’t interrupted. If you want to revert the settings, you can change the timeout value back to its original setting, usually 15 or 30 minutes, depending on your preferences.
Method 2: Prevent Idle Mode Using PowerShell Script
Another effective method to prevent idle mode is by creating a PowerShell script that continuously simulates user activity. This approach can be particularly useful if you need to keep your session active for extended periods. Here’s a simple script that can do just that:
while ($true) {
Add-Type -AssemblyName System.Windows.Forms
[System.Windows.Forms.SendKeys]::SendWait("{F15}")
Start-Sleep -Seconds 60
}
This script sends a keystroke every minute, keeping your session active.
In this script, we use the Add-Type
cmdlet to load the necessary assembly for sending keystrokes. The SendWait
method sends a simulated key press, which prevents the system from entering idle mode. The Start-Sleep
command ensures that the script waits for 60 seconds before sending the next keystroke. To stop the script, you can simply close the PowerShell window or interrupt the execution.
Method 3: Using PowerShell to Create a Scheduled Task
Creating a scheduled task with PowerShell can also help prevent idle mode. By setting up a task that runs periodically, you can ensure that your system remains active. Here’s how to create a scheduled task that runs a simple command:
$action = New-ScheduledTaskAction -Execute "powershell.exe" -Argument "-Command ""[System.Windows.Forms.SendKeys]::SendWait('{F15}');"""
$trigger = New-ScheduledTaskTrigger -AtStartup -RepeatIndefinitely -RepeatInterval (New-TimeSpan -Minutes 1)
Register-ScheduledTask -Action $action -Trigger $trigger -TaskName "PreventIdleMode" -User "SYSTEM"
This command creates a scheduled task that runs every minute.
Output:
Task 'PreventIdleMode' registered successfully.
In this example, we first define the action to be taken, which is to execute PowerShell and send a keystroke. Then, we set up a trigger that specifies the task should run at startup and repeat every minute. Finally, we register the task using Register-ScheduledTask
. This method is especially useful for users who need to maintain an active session over long periods without manual intervention.
Conclusion
Keeping your system active and preventing idle mode can significantly enhance productivity, especially when working on critical tasks. In this tutorial, we explored several methods using PowerShell, including changing power settings, running scripts, and creating scheduled tasks. Each method offers unique advantages, allowing you to choose the one that best fits your workflow. By implementing these techniques, you can ensure that your computer stays awake and ready for action whenever you need it.
FAQ
-
How can I revert the power settings back to default?
You can reset the power settings using the commandpowercfg -restoredefaultschemes
. -
Will running a PowerShell script to prevent idle mode consume more energy?
Yes, keeping your system active will consume more energy compared to allowing it to enter sleep mode. -
Can I use these methods on any Windows version?
Most of these methods are compatible with Windows 7, 8, 10, and later versions. -
Is it safe to use PowerShell to change system settings?
Yes, as long as you understand the commands you are executing, it is safe to use PowerShell.
- How do I stop a running PowerShell script?
You can stop a running script by closing the PowerShell window or pressing Ctrl+C in the console.