How to Execute Commands Using PowerShell Remoting
- What is PowerShell Remoting
-
Configure
WinRM
Listeners in PowerShell - Create a New PowerShell Session
- Use Invoke Methods to Run Quick Commands Remotely in PowerShell
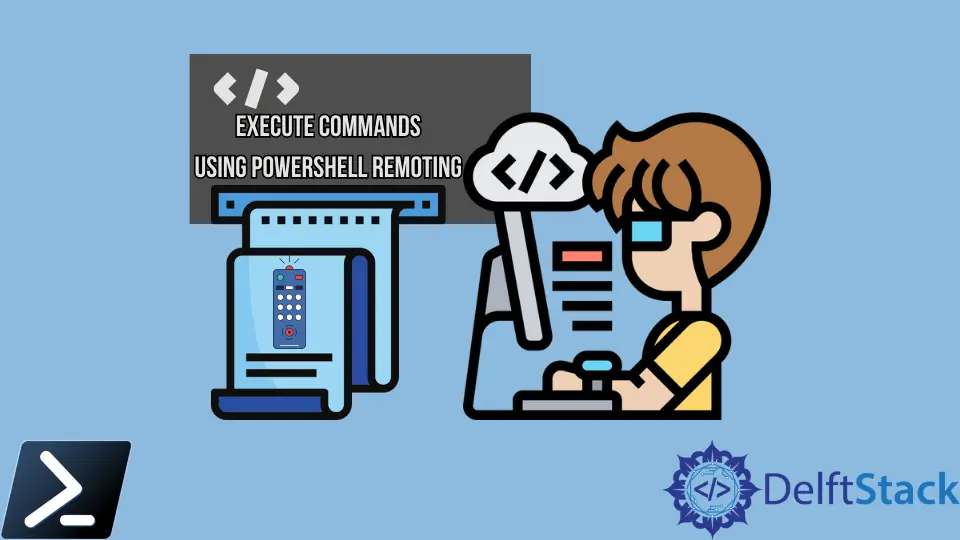
With Windows PowerShell, there are multiple ways to execute a command on local or remote computers. One way is enabling PowerShell remoting and using the PowerShell session commands.
This article will discuss how PowerShell remoting works, configuring WinRM
, a couple of examples of PowerShell session commands, and how we can remote into a computer with administrator access.
What is PowerShell Remoting
PowerShell Remoting (or PSRemoting
) is one of the frequently used features in PowerShell. As a result, we can seamlessly connect to one or more remote computers and execute commands using a single command.
In a nutshell, PSRemoting
allows us to run commands on remote computers just as if we were sitting in front of them. In addition, PSRemoting
provides features that connect and authenticate a user, run remote cmdlets, and displays any output from that cmdlet to the local computer.
Think of PSRemoting
like SSH
or telnet
or even psexec
. It is just a way to run commands on computers within PowerShell.
PSRemoting
heavily relies on running commands in a session. A session is a remote shell that runs commands inside.
Creating one of these sessions goes through many steps in the PowerShell console’s background.
When we initiate a PSRemoting
session, the following rough steps carry out:
-
The user attempts to connect to the destination server on a
WinRM
listener. TheWinRM
listener is a tiny web service that runs on a server.WinRM
is Microsoft’s version of an implementation standard calledWSMan
.WSMan
is an open-sourced standard created with many other large tech companies at the time, like Intel, Dell, and Sun Microsystems. -
The authentication process begins when the client connects to the listener over the HTTP or HTTPS protocol.
-
After the client connects and authenticates to the server,
PSRemoting
generates a session. -
After the
PSRemoting
generates the session, it is open for business. The client can start sending information to the server, returning any necessary output known as serialization.This communication is typically encrypted.
To enable PSRemoting
, type the command below into your PowerShell console.
Enable-PSRemoting –Force
Configure WinRM
Listeners in PowerShell
A client needs somewhere to connect over the network. The client needs to “talk” to something that is “listening” on the other side; the “listening” part is the role of the WinRM
listener.
You can discover all WinRM
listeners running on any Windows computer using the winrm
command below.
winrm e winrm/config/listener
WinRM
listeners have a few essential components.
- Listening address – The IP address they bind to. The listening address is the server IP address that the client connects to.
- Type of transport – Every
WinRM
listener needs a way to communicate with the client; they do this via transport using HTTP or HTTPS. - Optional certificate thumbprint – If a
WinRM
listener uses HTTPS for transport, it must know what private key to authenticate the client against; this key is found utilizing a certificate thumbprint.
To add a remote computer to a list of trusted hosts for the local computer in WinRM
, type in the following command below.
winrm s winrm/config/client '@{TrustedHosts="RemoteComputer"}'
To verify and check for the configuration of WinRM
, type in the following command below.
winrm quickconfig
Create a New PowerShell Session
The New-PSSession
command creates a Windows PowerShell session (PSSession
) on a local or remote computer. When creating a PSSession
, PowerShell establishes a persistent connection to the remote computer.
We can run commands on a remote computer without creating a PSSession
using the –ComputerName
parameters of Enter-PSSession
or Invoke-Command
.
When you use the –ComputerName
parameter, PowerShell creates a temporary connection used for the cmdlet and is then closed afterward.
Starting with Windows PowerShell 6.0, we can use Secure Shell (SSH) to create and establish a session on a remote machine if SSH is enabled on the local computer and the remote computer is configured with a Windows PowerShell SSH endpoint.
One of the benefits of an SSH-based Windows PowerShell remote initiated session is that it can work across multiple platforms (Windows, Linux, macOS) or cross-platform.
For SSH-based sessions, we can use the -HostName
or -SSHConnection
parameters to specify the remote computer and other relevant connection information.
New-PSSession -ComputerName DC01 -Credential (Get-Credential)
Remember that the session generated by the New-PSSession
command is persistent. Meaning we can use it multiple times.
However, we must remember that we should close the session after using the Remove-PSSession
command to avoid security risks.
Use Invoke Methods to Run Quick Commands Remotely in PowerShell
The Invoke-Command
cmdlet is preferable if we write the executed commands now, as we retain IntelliSense in our IDE. Also, Invoke-Command
is advantageous if we run quick and easy, one-time use commands.
The session of any Invoke
methods is non-persistent and doesn’t retain any session that any malicious users can abuse.
The snippet below is an example of Invoke-Command
that passes a username and a secure string password that will enable the execution of commands on the administrator level.
Script Sample:
$username = "Username"
$password = "Password"
$secstr = New-Object -TypeName System.Security.SecureString
$password.ToCharArray() | ForEach-Object { $secstr.AppendChar($_) }
$cred = New-Object -TypeName System.Management.Automation.PSCredential -ArgumentList $username, $secstr
Invoke-Command -ComputerName RemoteServer -ScriptBlock { Get-Process } -Credential $cred
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn