How to Split Commands to Multiple Lines in PowerShell
- Windows PowerShell Multiline Command
- Breaking Long Lines of Code Using Specific Operators
- Conclusion
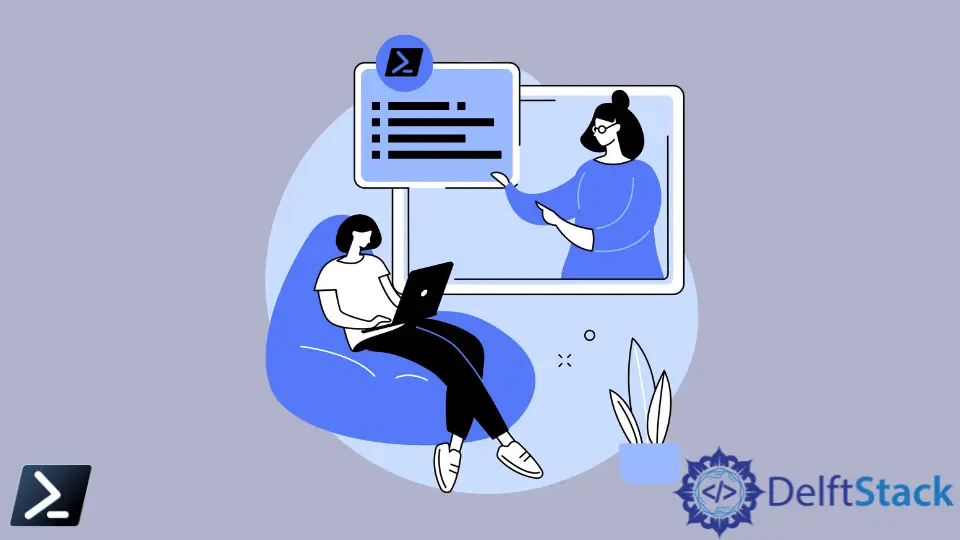
Readable code very easily communicates its purpose of functionality to the users. Variable names and method names should have proper naming conventions for code readability.
Other attributes that contribute to code readability are consistent indentation and formatting style. Windows PowerShell multiline command helps split long command lines into multiple statements for readability.
In Windows PowerShell, multiline commands can be easily created using the backtick character to split long or single-line commands multiline statements.
The backtick character is used as a kind of escape character. It escapes the newline character and results in line continuation.
This article will explain the importance of Windows PowerShell multiline commands to split long commands over multiple lines.
Windows PowerShell Multiline Command
To split a long command into multiple lines, use the backtick character to break it into multiple lines.
For example, we want to get the free disk space information on the local computer. Unfortunately, the script to get this specific information is an extended command, making it difficult to read and manage.
Example Code:
Get-WmiObject -Class win32_logicaldisk | Format-Table DeviceId, MediaType, @{n = "Size"; e = { [math]::Round($_.Size / 1GB, 2) } }, @{n = "FreeSpace"; e = { [math]::Round($_.FreeSpace / 1GB, 2) } }
It may look like the syntax is split in this article, but when copied to the command-line interface, the example above is a very long one-liner script.
We can easily split long commands into multiple lines using Windows PowerShell backtick characters for a line break in a given command.
Example Code:
Get-WmiObject -Class win32_logicaldisk `
| Format-Table DeviceId, `MediaType, @{n = "Size"; e = { [Math]::Round($_.Size / 1GB, 2) } }, `
@{n = "FreeSpace"; e = { [Math]::Round($_.FreeSpace / 1GB, 2) } }
In our code, we utilize backticks as line continuation characters in PowerShell to enhance readability by splitting a long command across multiple lines. We begin with Get-WmiObject -Class win32_logicaldisk
, which fetches information about logical disks on the system.
The backtick at the end of this line indicates that the command continues on the next line. We then pipe (|
) the output to Format-Table
, a cmdlet that formats the output as a table.
Here, we specifically choose to display DeviceId
and MediaType
. For the Size
and FreeSpace
properties, we use calculated fields (noted by @{n = "Name"; e = { Expression }}
syntax).
Within these calculated fields, we employ backticks again for line continuation. The expressions use [Math]::Round($*.Size / 1GB, 2)
and [Math]::Round($*.FreeSpace / 1GB, 2)
to convert the size and free space from bytes to gigabytes and round them to two decimal places, respectively.
By breaking down this complex command with backticks, we make the script more manageable and its intent clearer, aiding both in its maintenance and readability.
Output:
The code structure is easily readable and easy to maintain in Windows PowerShell using the multiline command. However, the backtick character is not usually recommended because the character is hard to read and invites bugs.
So, we have an alternate method of breaking long lines of code.
Breaking Long Lines of Code Using Specific Operators
PowerShell is intuitive when it comes to line continuation. Certain operators and syntax structures inherently signal to the interpreter that a command spans multiple lines.
This is particularly useful in complex scripts where readability can significantly impact the ease of maintenance and understanding.
Breaking Long Lines of Code Using Pipeline (|
)
Usually, you get automatic line continuation when a command cannot syntactically be completed at that point.
One example would be starting a new pipeline element (|
). The pipeline will work without problems since, after the pipeline operator, the command cannot be completed since it’s missing another pipeline element.
So, what our interpreter does is look for the next pipeline element in the following command line.
Example Code:
Get-Process |
Where-Object { $_.ProcessName -like "powershell" } |
Select-Object Id, ProcessName, CPU
In our script, we start with Get-Process
, which retrieves all the currently running processes. The output of Get-Process
is then piped into Where-Object
, where we filter the processes whose name includes powershell
.
This is achieved by using the -like
operator in a script block {}
to match the process names.
Following this, we pipe the filtered results into Select-Object
. Here, we specify that we only want to retain the Id
, ProcessName
, and CPU
usage of these filtered processes.
By using the pipeline, we have effectively broken down the command into manageable parts, each on a separate line. This not only makes our script more readable but also simplifies debugging and future modifications.
Output:
Breaking Long Lines of Code Using Comma (,
)
A comma (,
) will also work in some contexts, like the pipeline operator.
In certain contexts, such as within a command or script block, the comma operator can also serve as a line continuation signal. This is especially useful in formatting commands or when passing a list of items.
Example Code:
Get-EventLog -LogName Application -Newest 5 |
Format-Table TimeGenerated,
EntryType,
Source,
@{n = 'Message'; e = { $_.Message.Split("`n")[0] } }
In our script, we use Get-EventLog
to fetch the newest 5 entries from the Application log. This output is piped into Format-Table
for formatting.
We then use the comma method to list the properties we want in the table: TimeGenerated
, EntryType
, Source
, and a custom expression for Message
. The custom expression for Message
uses a calculated property (@{n='Message'; e= { $_.Message.Split("n")[0] } }
) to display only the first line of the message, enhancing readability.
By splitting this command into multiple lines using commas, we have made the script more readable, especially when dealing with multiple properties or complex expressions.
Output:
Breaking Long Lines of Code Using Curly Braces ({}
)
Also, curly braces ({}
) when defining script blocks will allow line continuation directly. They are indispensable in scenarios involving loops, conditional statements, or when passing a block of commands to cmdlets.
Example Code:
$processes = Get-Process
$filteredProcesses = $processes | Where-Object {
$_.WorkingSet -gt 100MB
} | ForEach-Object {
$_.ProcessName
}
$filteredProcesses
We start by getting a list of all processes using the Get-Process
cmdlet. The pipeline then passes these processes to Where-Object
, where a script block filters out processes with a working set greater than 100MB
.
The surviving processes are passed to another script block in ForEach-Object
, which extracts and outputs their names. The use of curly braces for script blocks allows for a clear, concise, and functional flow of the data through the pipeline.
Output:
Conclusion
Throughout this article, we’ve explored various methods to enhance the readability of PowerShell scripts. Readability is crucial for easy understanding and maintenance of scripts, especially in Windows PowerShell, where scripts can often become lengthy and complex.
We started by discussing the common practice of using the backtick character for multiline commands, which, while effective, can sometimes lead to readability issues and potential bugs due to its subtle nature.
However, PowerShell offers more intuitive methods for breaking long lines of code, which we delved into subsequently. The use of specific operators like the pipeline (|
), comma (,
), and curly braces ({}
) offers a more readable and maintainable approach to script writing.
These methods not only improve the visual structure of the code but also enhance the logical flow, making scripts easier to debug and modify.
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn