Line Break in PowerShell
- Line Breaks in PowerShell
-
Use
`N
to Break Lines in PowerShell -
Use
[Environment]::NewLine
to Break Lines in PowerShell -
Combine
`N
and[Environment]::NewLine
to Break Lines in PowerShell - Conclusion
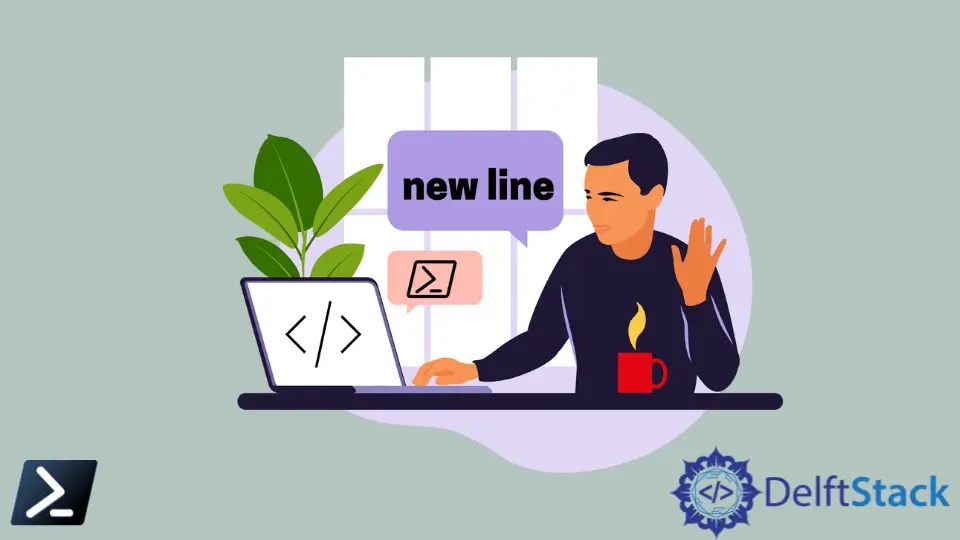
Line breaks are essential when you want to format your output or control the flow of text in your scripts. In PowerShell, you can achieve this using escape sequences and special characters.
In this tutorial, we’ll teach you the way to break lines in PowerShell.
Line Breaks in PowerShell
PowerShell includes a set of special character sequences for representing characters, such as line breaks and tabs. They are also known as escape sequences.
The backslash \
shows a special character in most programming languages. But, PowerShell uses the backtick character `
.
So, escape sequences begin with `
and are case-sensitive. Here are some common escape sequences in PowerShell:
`0 |
Null |
`a |
Alert |
`b |
Backspace |
`e |
Escape |
`f |
Form feed |
`n |
New line |
`r |
Carriage return |
Escape sequences are interpreted only when enclosed in double quotes (" "
).
Use `N
to Break Lines in PowerShell
One of the most common uses of escape sequences in PowerShell is to insert line breaks. We can use the new line `n
character to break lines in PowerShell, and the line break or new line is added after the `n
character.
The following example shows how to use `n
to break lines in the output.
Write-Host "Welcome to the`nPowerShell Tutorial."
Output:
Welcome to the
PowerShell Tutorial.
In the output above, the "Welcome to the"
is displayed on the first line. Then, the `n
character is encountered, causing a line break, and the cursor moves to the beginning of the next line and the "PowerShell Tutorial."
is displayed on the second line.
We can also use multiple `n
characters to create multiple line breaks. In this code example, the `n
character is used to introduce a line break in the output, making it more readable.
Write-Host "Welcome `nto `nthe `nPowerShell Tutorial."
Output:
Welcome
to
the
PowerShell Tutorial.
As we can see in the output, the string has multiple line breaks by using multiple `n
characters.
In this next example, we have an array of fruits stored in the $fruits
variable. Then, we create a list of fruits with each fruit name on a new line, we use the -join
operator, which combines the elements of the array with the specified separator, in this case, the `n
line break character.
$fruits = 'Apple', 'Banana', 'Cherry', 'Date'
$fruitList = $fruits -join "`n"
Write-Host $fruitList
Output:
Apple
Banana
Cherry
Date
This results in a list of fruits, with each one displayed on a new line.
Use [Environment]::NewLine
to Break Lines in PowerShell
Another method to create line breaks in PowerShell is by using the [Environment]::NewLine
object, which is equivalent to (`n`r
). The `r
character moves the cursor to the beginning of the current line.
The following examples show how to use [Environment]::NewLine
to break lines when displaying the array items in the output.
In the first example, we manipulate an array named $number
containing 'one'
, 'two'
, 'three'
, and 'four'
. Our objective is to format the output, displaying each element on a new line.
Next, we create a variable $new
, which holds the platform-specific newline character [Environment]::NewLine
. We then leverage the pipeline operator |
to process each element in $number
using ForEach
.
Then, inside the ForEach
block, we construct a string for each element by combining $_
(the element) and $new
, creating a new line break after each element. This approach results in neatly formatted output, as each element is presented on a new line.
Example Code 1:
$number = 'one', 'two', 'three', 'four'
$new = [Environment]::NewLine
$number | foreach { "$_$new" }
Output:
one
two
three
four
The output showcases each element from the $number
array on a new line.
In the next example, we begin by setting up data, defining an array called $numbers
with values and a variable $newLine
for line breaks. Then, we iterate through $numbers
using ForEach-Object
, calculate each element’s position in the array, and create formatted numbered lists with line breaks.
Next, these lists are stored in $numberedList
. Finally, Write-Host
displays the numbered lists, providing organized and formatted output.
Example Code 2:
$numbers = 'one', 'two', 'three', 'four'
$newLine = [Environment]::NewLine
$numberedList = $numbers | ForEach-Object {
$index = [array]::IndexOf($numbers, $_) + 1
"$index. $_$newLine"
}
Write-Host $numberedList
Output:
1. one
2. two
3. three
4. four
The output is a numbered list with each element from the $numbers
array displayed in sequential order.
In this code example, we utilize the [Environment]::NewLine
object to create line breaks in the output, making it more organized and easier to read.
Combine `N
and [Environment]::NewLine
to Break Lines in PowerShell
We can combine the `n
escape sequence with [Environment]::NewLine
to create custom line breaks.
In the following code, we create a custom two-line output. We define two variables, $line1
and $line2
, each containing a line of text.
Then, to create platform-independent line breaks, we use a variable, $customLineBreak
, which combines the "`n"
newline character and [Environment]::NewLine
. We then utilize Write-Host
to display the content by concatenating $line1
, $customLineBreak
, and $line2
within double quotes, ensuring a clean two-line output.
Example Code:
$line1 = "This is the first line"
$line2 = "This is the second line"
$customLineBreak = "`n" + [Environment]::NewLine
Write-Host "$line1$customLineBreak$line2"
Output:
This is the first line
This is the second line
In this example, a custom line break is created by combining the `n
and [Environment]::NewLine
to separate the two lines.
Conclusion
In conclusion, mastering line breaks and text formatting in PowerShell is essential for creating clear and organized scripts. We explored escape sequences, emphasizing the commonly used `n
character for line breaks.
Additionally, we discussed the usage of [Environment]::NewLine
for precise control over line breaks, exemplifying its application in array displays. By understanding these techniques, scriptwriters can produce well-structured and readable PowerShell scripts, ensuring efficient and effective communication.