LastExitCode in PowerShell
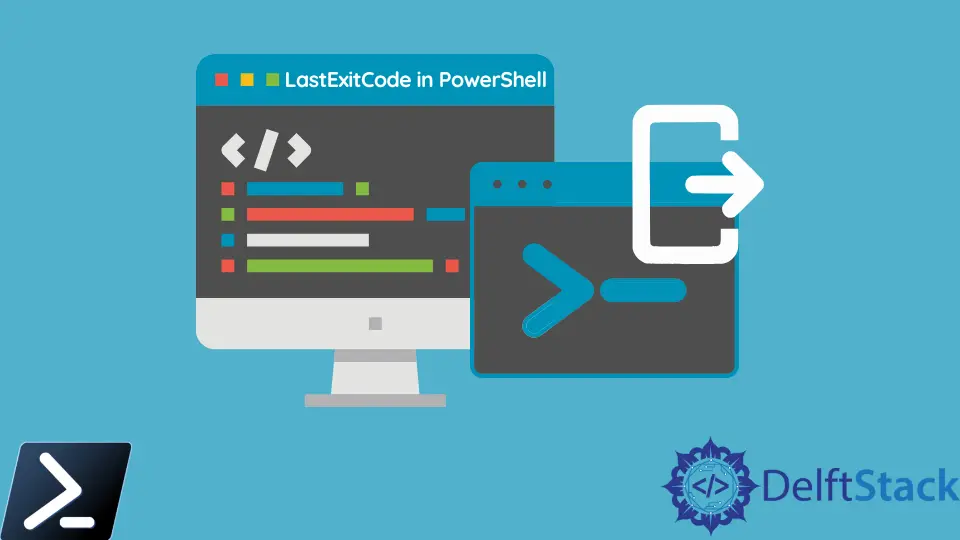
Understanding the intricacies of error handling in PowerShell is essential for effective scripting. Among the various tools available, $LastExitCode
stands out as a powerful mechanism for capturing the exit code of external commands.
This article delves into the nuanced use of $LastExitCode
, exploring its application in different scenarios, from basic error checking to dynamic command execution. By mastering $LastExitCode
, PowerShell users can enhance their scripts’ robustness and reliability.
Use of $?
in a PowerShell Environment
It is important to understand the $?
command before using it here. In PowerShell, $?
is an automatic variable that helps determine the success or failure of the last operation and can be used as an error handler.
Based on the last command executed, it returns a Boolean value, True
or False
. If the last command executed in the script is successful, it returns true
; otherwise, it returns false
.
One execution of this command is shown in the execution code below.
Remove-Item -Path "example.txt"
if ($?) {
Write-Host "File removed successfully."
}
else {
Write-Host "Failed to remove file."
}
In this example, we first attempt to remove a file named example.txt
. After executing the Remove-Item
command, we use the $?
variable in the if
statement to check if the operation was successful.
If $?
evaluates to True
, we print a success message; otherwise, we print a failure message.
There is a difference between the $lastexitcode
and the $?
. However, it is also used for error handling.
$LastExitCode
as an Error Handler in PowerShell
There is a difference in error handling used when the command is internal and when the command is external.
You will use the $lastexitcode
when the command is external. This is because this command applies only to external scripts and commands.
The primary purpose of $LastExitCode
is to provide feedback on the success or failure of external commands executed within a PowerShell session. By accessing $LastExitCode
, users can determine whether the last command executed successfully or encountered an error.
Example:
# Execute an external command (ping)
ping example.test
# Check the value of $LastExitCode
$exitCode = $LastExitCode
# Output the exit code
Write-Host "Exit code: $exitCode"
In this example, we attempt to ping a host named example.test
. After executing the ping
command, we store the value of $LastExitCode
in a variable named $exitCode
.
Finally, we output the exit code using the Write-Host
cmdlet. This approach allows us to access and utilize the exit code of the last command executed directly within our PowerShell script.
Output:
$LastExitCode
With Invoke-Expression
as an Error Handler in PowerShell
In PowerShell, the Invoke-Expression
cmdlet allows for the execution of commands or scripts stored in string variables. When combined with $LastExitCode
, it provides a way to capture the exit code of dynamically executed commands.
Example:
# Define a command as a string
$command = "ping example.test"
# Execute the command using Invoke-Expression
Invoke-Expression -Command $command
# Capture the exit code using $LastExitCode
$exitCode = $LastExitCode
# Output the exit code
Write-Host "Exit code: $exitCode"
In this example, we define a command (ping example.test
) as a string variable $command
. We then use Invoke-Expression
to execute the command stored in the $command
.
After execution, we capture the exit code using $LastExitCode
and store it in the variable $exitCode
. Finally, we output the exit code to the console.
Output:
When the previous script was true
, the $LastExitCode
output would always be 0.
But, when it is unsuccessful, it would be 1 or any other integer returned by the external script because the $LastExitCode
command is not binary, unlike the $?
command.
$LastExitCode
With Conditional Checks as an Error Handler in PowerShell
Conditional statements allow us to make decisions based on the success or failure of commands within a PowerShell script. Let’s see how we can leverage $LastExitCode
within these statements to implement robust error handling and conditional logic.
Example:
# Execute an external command (ping)
ping example.test
# Check the value of $LastExitCode
if ($LastExitCode -eq $null) {
Write-Host "No exit code available. An external command might not have been executed."
}
else {
Write-Host "Exit code: $LastExitCode"
}
In this example, we attempt to ping a host named example.test
. After executing the ping
command, we check the value of $LastExitCode
.
If $LastExitCode
is null, it indicates that the ping
command may not have been executed, possibly due to an invalid host name or network issue. Otherwise, we print the exit code, providing insight into the success or failure of the ping
command.
Output:
Conclusion
This article has provided a comprehensive overview of utilizing $LastExitCode
as an error handler in PowerShell scripting. We explored its use in various contexts, including basic error checking with $?
, error handling for external commands with $LastExitCode
, dynamic command execution with Invoke-Expression
, and conditional checks.
By mastering these techniques, PowerShell users can enhance their scripting capabilities, ensuring robust error detection and handling in their scripts. To further deepen your understanding, consider exploring advanced topics such as error trapping and handling specific error scenarios.
With a solid grasp of error handling techniques in PowerShell, you’ll be well-equipped to develop reliable and resilient scripts for various automation tasks.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.