How to Get IPv4 Address in PowerShell
-
Use
Get-NetIPAddress
to Get IPv4 Address Into a Variable in PowerShell -
Use
Get-NetIPConfiguration
to Get IPv4 Address Into a Variable in PowerShell -
Use
Dns class
to Get IPv4 Address Into a Variable in PowerShell -
Use
Get-WmiObject
to Get IPv4 Address Into a Variable in PowerShell -
Use
Test-Connection
to Get IPv4 Address Into a Variable in PowerShell -
Use
Get-CimInstance
to Get IPv4 Address Into a Variable in PowerShell - Conclusion
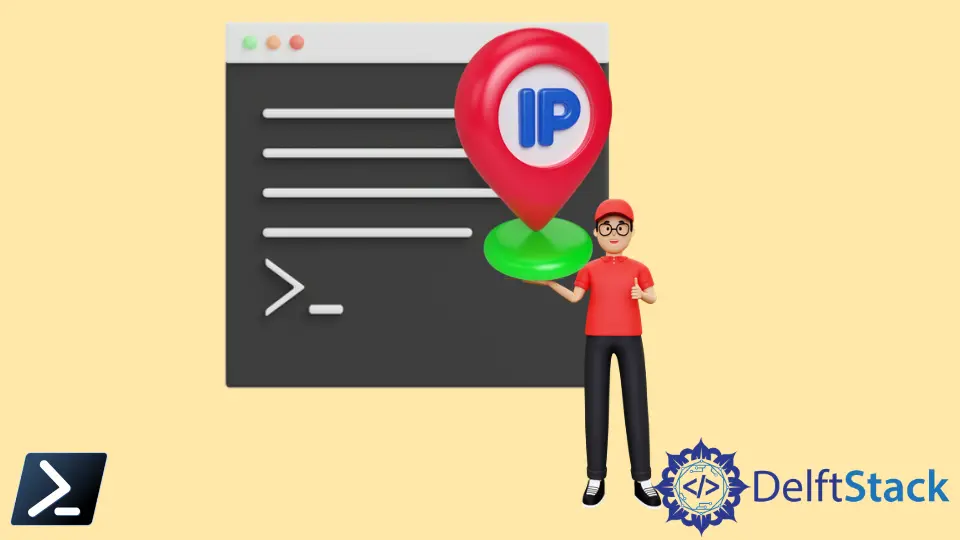
System administrators can perform various tasks in PowerShell, including networking tasks.
For example, PowerShell allows you to list IP addresses and IP configuration, ping a computer, retrieve network adapter properties, perform DHCP configuration tasks, and create/remove a network share.
IP means Internet Protocol, and v4 means Version Four.
IPv4 address is 32-bit integers that identify a network interface on a machine. It is expressed in dotted-decimal notation.
It has four 8-bit fields separated by periods, and each 8-bit field represents a byte of the IPv4 address. This tutorial will teach you to get an IPv4 address into a variable in PowerShell.
Use Get-NetIPAddress
to Get IPv4 Address Into a Variable in PowerShell
The Get-NetIPAddress
cmdlet gets the IP address configuration, such as IPv4 addresses, IPv6 addresses, and the IP interfaces associated with addresses.
When it is run without parameters, it prints the entire IP address configuration for the computer.
The --AddressFamily
parameter is used to specify an array of IP address families. The Get-NetIPAddress
gets the IP address configuration that matches the address families with this parameter.
The accepted values are IPv4
and IPv6
.
For example, the command below gets information about IP address configuration for all IPv4 addresses on the computer.
Get-NetIPAddress -AddressFamily IPv4
This command gets only the computer’s IPv4
address.
(Get-NetIPAddress | Where-Object { $_.AddressState -eq "Preferred" -and $_.ValidLifetime -lt "24:00:00" }).IPAddress
In the provided code snippet, we utilize the Get-NetIPAddress
cmdlet to gather IP address data, followed by the Where-Object
cmdlet to filter results based on specific criteria encapsulated within curly braces. The conditions within the Where-Object
block, denoted by the -eq
and -lt
comparison operators, target IP addresses in the Preferred
state and those with a valid lifetime of less than 24 hours, respectively.
By accessing the IPAddress
property of the filtered results, enclosed within parentheses, we isolate and display the IPv4 addresses meeting the established conditions. This concise analysis highlights the pipeline-driven approach in PowerShell, where each cmdlet efficiently processes data streams to produce refined outputs.
Output:
You can also use the following command to get an IPv4 address in PowerShell.
Get-NetIPAddress -AddressFamily IPv4 -InterfaceIndex $(Get-NetConnectionProfile | Select-Object -ExpandProperty InterfaceIndex) | Select-Object -ExpandProperty IPAddress
In the provided code example, we utilize the Get-NetIPAddress
cmdlet to fetch IP address information. By specifying -AddressFamily IPv4
, we restrict the results to IPv4 addresses.
The -InterfaceIndex
parameter is populated with the interface index retrieved using Get-NetConnectionProfile
, ensuring that IP addresses associated with the current network connection are obtained. Finally, we use Select-Object -ExpandProperty IPAddress
to extract and display the IP addresses from the output.
Output:
Use Get-NetIPConfiguration
to Get IPv4 Address Into a Variable in PowerShell
The Get-NetIPConfiguration
cmdlet is a powerful tool used to retrieve IP address information on Windows systems. This method provides comprehensive data about IP configurations, including IPv4 and IPv6 addresses, subnet masks, default gateways, and network adapter statuses, aiding administrators and scripters in network-related tasks.
Example:
(Get-NetIPConfiguration | Where-Object { $_.IPv4DefaultGateway -ne $null -and $_.NetAdapter.status -ne "Disconnected" }).IPv4Address.IPAddress
In the provided code snippet, we utilize the Get-NetIPConfiguration
cmdlet to gather IP address information, followed by the Where-Object
cmdlet to filter results based on specific criteria encapsulated within curly braces. The conditions within the Where-Object
block, denoted by the -ne
comparison operator, target configurations with a non-null IPv4 default gateway and network adapters that are not in a Disconnected
status, respectively.
By accessing the IPAddress
property of the filtered results, enclosed within parentheses, we isolate and display the IPv4 addresses that meet the established criteria. This concise analysis highlights the PowerShell pipeline’s capability to efficiently process data streams, allowing for refined outputs that aid in network configuration and troubleshooting tasks.
Output:
Use Dns class
to Get IPv4 Address Into a Variable in PowerShell
The Dns class
is a static class that gets information about a specific host from the Internet Domain Name System (DNS). The GetHostAddresses
method returns the IP addresses for the specified host.
This approach can be particularly useful when working in environments where direct access to cmdlets like Get-NetIPConfiguration
or Get-NetIPAddress
is not available or not suitable.
Example:
([System.Net.DNS]::GetHostAddresses($env:computername) | Where-Object { $_.AddressFamily -eq "InterNetwork" } | Select-Object IPAddressToString)[0].IPAddressToString
In the provided code example, we use [System.Net.DNS]::GetHostAddresses
to retrieve the IP addresses associated with the local computer specified by $env:computername
. We then filter the results using Where-Object
to select only IPv4 addresses (AddressFamily -eq "InterNetwork"
).
Next, we use Select-Object IPAddressToString
to extract the IP address as a string representation. Finally, [0].IPAddressToString
selects the first IP address from the result and displays it as a string.
Output:
Use Get-WmiObject
to Get IPv4 Address Into a Variable in PowerShell
The Get-WmiObject
cmdlet gets instances of Windows Management Instrumentation (WMI) classes or information about the available WMI classes.
The Win32_NetworkAdapterConfiguration
is a WMI class that represents the attributes and behaviors of a network adapter.
Example:
(Get-WmiObject -Class Win32_NetworkAdapterConfiguration | Where-Object { $_.DHCPEnabled -ne $null -and $_.DefaultIPGateway -ne $null }).IPAddress | Select-Object -First 1
In the provided code snippet, we use Get-WmiObject
to query the Win32_NetworkAdapterConfiguration
class, retrieving network adapter configuration details. We then apply a Where-Object
filter to select configurations where DHCP is enabled and a default gateway is specified.
This ensures we retrieve IP addresses associated with active network connections. Finally, we use Select-Object -First 1
to select the first IP address from the results.
Output:
Use Test-Connection
to Get IPv4 Address Into a Variable in PowerShell
The Test-Connection
cmdlet sends ICMP echo request packets, or pings, to one or more computers and returns the echo response replies. It can also determine whether a specific computer can be contacted across an IP network.
Example:
(Test-Connection -ComputerName (hostname) -Count 1 | select -ExpandProperty IPv4Address).IPAddressToString
In the provided code example, we use Test-Connection
to ping a remote host specified by (hostname)
and retrieve network-related information. The -Count 1
parameter ensures that only a single echo request is sent to the target computer, minimizing network traffic.
We then use Select-Object -ExpandProperty IPv4Address
to extract and display the IPv4 address of the remote host from the output.
If you have any virtual adapters, it returns an IPv4 address of virtual adapters (e.g., VPN, Hyper-V, Docker).
Output:
You can also use this command, which returns the same output as above.
(Test-Connection -ComputerName $env:computername -Count 1).IPv4Address.IPAddressToString
In the provided code example, we utilize Test-Connection
to ping the local system specified by $env:computername
and retrieve network-related information. By specifying -Count 1
, we send a single ping request to the local system, minimizing network traffic.
We then access the IPv4Address
property of the output object and further access the IPAddressToString
property to retrieve the IPv4 address as a string.
Output:
Use Get-CimInstance
to Get IPv4 Address Into a Variable in PowerShell
The Get-CimInstance
cmdlet offers a robust approach to retrieve information from the Windows Management Instrumentation (WMI) repository, allowing administrators to query network adapter configurations easily. By targeting the Win32_NetworkAdapterConfiguration
CIM class, this method enables users to obtain comprehensive details about network settings, including IP addresses, subnet masks, default gateways, and more.
Example:
(Get-CimInstance -ClassName Win32_NetworkAdapterConfiguration | Where-Object { $_.IPAddress -ne $null }).IPAddress[0]
In the provided code example, we utilize Get-CimInstance
to query the Win32_NetworkAdapterConfiguration
CIM class, retrieving information about network adapter configurations. The Where-Object
cmdlet filters the results to select configurations where the IPAddress
property is not null, ensuring that only network adapter configurations with assigned IP addresses are returned.
By accessing the [0]
index, we select the first IP address from the result set, which can be useful when dealing with multiple network adapters or addresses. This approach provides administrators with a concise way to retrieve the primary IP address associated with the system, facilitating network management and troubleshooting tasks.
Output:
Conclusion
In this article, we explored various methods to retrieve the IPv4 address in PowerShell, leveraging different cmdlets and classes available in the PowerShell ecosystem. Starting with Get-NetIPAddress
, we learned how to fetch IP address information with specific filtering options, followed by Get-NetIPConfiguration
, which provides comprehensive data about IP configurations on Windows systems.
We then explored using the Dns
class for scenarios where direct access to cmdlets isn’t available. Additionally, we examined the usage of Get-WmiObject
to query network adapter configurations via WMI and Test-Connection
to ping remote hosts and gather IP address details.
Finally, we delved into utilizing Get-CimInstance
to retrieve network adapter configurations efficiently from the WMI repository. Each method offers unique advantages, catering to different use cases and scenarios in network administration and troubleshooting tasks.