How to Get Disk Space Information Using PowerShell
- Get Disk Space Information Using the PSDrive Library in PowerShell
- Get Disk Space Information Using WMI Object in PowerShell
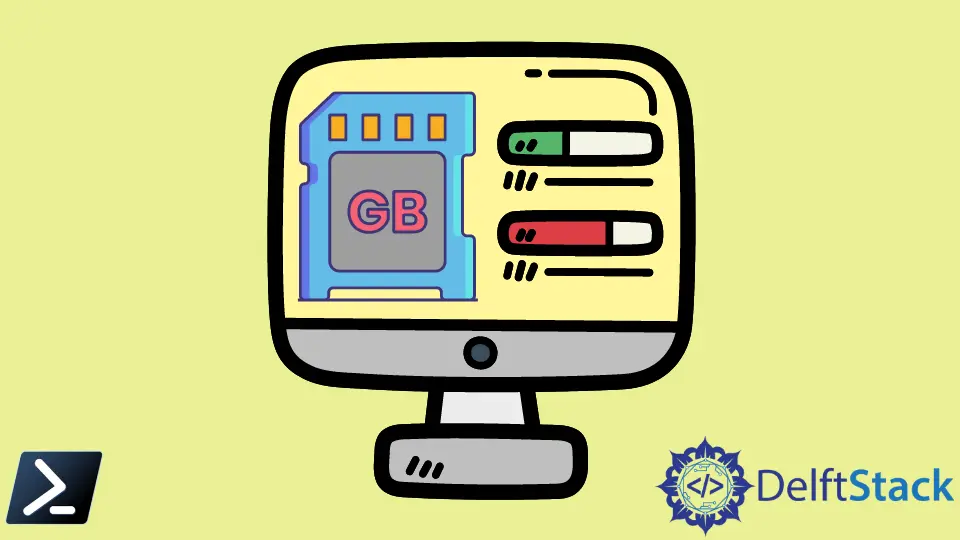
PowerShell can generate comprehensive reporting on our Windows operating system systems. One of the metrics in these reports is the list of our system drives and their drive space information.
In this article, we will learn a couple of commands that will export information on all of our system drives and learn how to determine how much drive space is left in our machine using PowerShell.
Get Disk Space Information Using the PSDrive Library in PowerShell
The PSDrive library is a set of commands in charge of managing, creating, and deleting temporary and persistent drives using PowerShell. This first method will focus on the query command Get-PSDrive
.
By running the Get-PSDrive
command, we can quickly get the information on all our repositories mounted in our system.
Example Code:
Get-PSDrive
Output:
Name Used (GB) Free (GB) Provider Root CurrentLocation
---- --------- --------- -------- ---- ---------------
Alias Alias
C 442.84 21.56 FileSystem C:\ Users\KentMarion
Cert Certificate \
D 3507.38 218.63 FileSystem D:\
Env Environment
Function Function
G 519.22 412.28 FileSystem G:\
HKCU Registry HKEY_CURRENT_USER
HKLM Registry HKEY_LOCAL_MACHINE
Variable Variable
WSMan WSMan
As we have seen from the output above, the Get-PSDrive
command has a Name
attribute in its object. By knowing this information, we can isolate a specific drive by filtering its drive name and selecting another object attribute to determine its free size.
Run the following command to test this out.
Example Code:
Get-PSDrive -Name C | Select-Object Name, Free
Output:
Name Free
---- ----
C 23061344256
However, if we compare our latest output with the output beforehand, we can see that the free size has converted itself into bytes. Therefore, to reconvert the value to gigabytes (GB), we must set a custom expression when selecting the object attribute.
Run the following command to test this out.
Example Code:
Get-PSDrive -Name C | Select-Object Name, @{Name = "Free (GB)"; Expression = { [Math]::Round($_.Free / 1GB, 2) } }
Output:
Name Free (GB)
---- ----
C 21.41
The example code above renames the column to Free (GB)
and rounds the output of the floating value to two decimal places.
Get Disk Space Information Using WMI Object in PowerShell
Another method of getting disk space information is using the WMI Object class. This class functions similarly to PSDrive, but the only difference is that this library can query for remote computers.
Example Code:
Get-WmiObject Win32_LogicalDisk -Filter "DeviceID='C:'" | Select-Object DeviceID, @{Name = "Free (GB)"; Expression = { [Math]::Round($_.FreeSpace / 1GB, 2) } }
Output:
DeviceID Free (GB)
-------- ---------
C: 21.42
To initiate a query on a remote computer, add the ComputerName
parameter inside the Get-WMIObject
command.
Get-WmiObject Win32_LogicalDisk -ComputerName "REMOTE-PC" -Filter "DeviceID='C:'"
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn