PowerShell Function Parameters
- Definine PowerShell Function Parameters
- Named Parameters in PowerShell Function
- Positional Parameters in PowerShell Function
- Switch Parameters in PowerShell Function
- Splatting in PowerShell Function Parameters
- PowerShell Parameter Attributes
- Advanced Function With Named Parameter Syntax
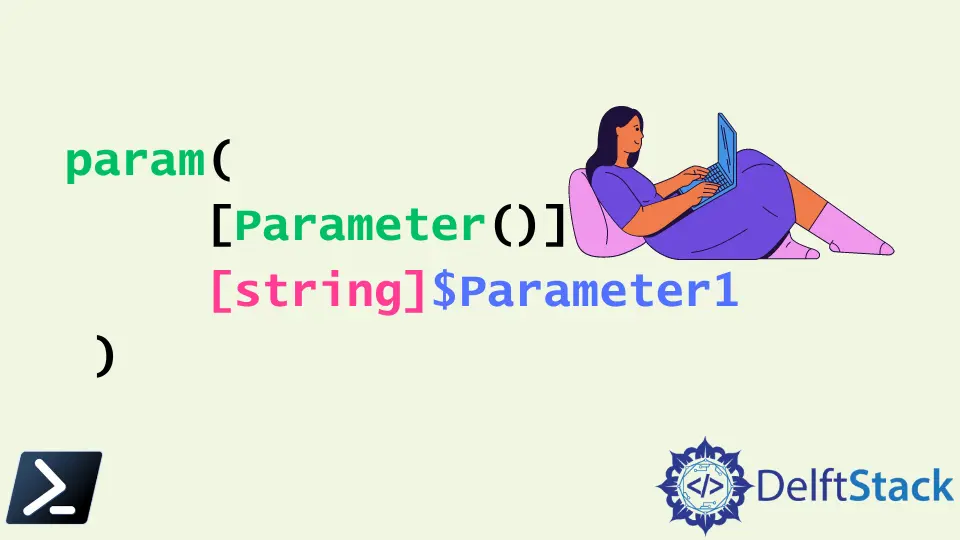
PowerShell function parameters make functions more powerful by leveraging characteristics and arguments to limit users from entering particular values. It adds a few rules and validations to prevent having to write extensive scripts, and they’re simple to use.
Arguments can also be given via the command line once the function parameters have been specified. Named, positional, switch, and dynamic parameters are all examples of function parameters. Function parameters can also be used to turn a basic function into a more complex one.
Definine PowerShell Function Parameters
Parameters are defined as follows.
param(
$Parameter1
)
Best practices recommend adding a type to the parameter and inserting a Parameter()
block to guarantee the parameter takes just the sort of input you need and will enable more features in the future. Therefore it is done as shown below.
param(
[Parameter()]
[string]$Parameter1
)
Even additional parameters can be added to the above syntax, as shown below.
param(
[Parameter()]
[string]$Param1,
[Parameter()]
[string]$Param2
)
Write-Host "Param1 value is $Param1"
Write-Host "Param 2 value is $Param2"
Named Parameters in PowerShell Function
Named Parameters are one technique to use PowerShell function parameters inside a script. Use the complete name of the argument when invoking a script or function with named parameters.
Let’s assume the above PowerShell script is stored in test.ps1
. Here we will be providing values to param1 and param2; you can notice in the example below the full name of the parameter should be passed.
./test.ps1 -Param1 'value' -Param2 'valuenext'
Output:
When you execute this script, you’ll see something like this, where each argument variable is replaced with the value supplied at runtime.
Positional Parameters in PowerShell Function
Passing items by position instead of name is another way to utilize parameters. The parameter’s name isn’t utilized when passing a parameter value via position. Instead, PowerShell compares the values of each argument to its location in the function. PowerShell recognizes that Param1 was specified before Param2 in the code and matches the values in the correct sequence.
./test.ps1 'value' 'valuenext'
Output
Param1 value value
Param 2 value is valuenext
Both param1 and param2 were declared as string types in the above example, but it’s not the only type you may use. Any type from the .NET class library can be used.
Apart from the original order, we can also go forward by giving the position inside the parameter construct as well. It is shown below.
param(
[Parameter(position = 1)]
[string]$Param1,
[Parameter(position = 0)]
[string]$Param2
)
Write-Host "Param1 value is $Param1"
Write-Host "Param 2 value is $Param2"
Here, you can see that the order of the parameters is changed.
./test.ps1 'param2' 'param1'
Switch Parameters in PowerShell Function
This parameter is used to signify whether something is on or off using binary or Boolean values. The switch
type is used to specify it. Continuing with the above example, we will add another switch parameter named DisplayParam2
.
param(
[Parameter()]
[string]$Param1,
[Parameter()]
[string]$Param2,
[Parameter()]
[switch]$DisplayParam2
)
Write-Host "Param1 value is $Param1"
if ($DisplayParam2.IsPresent) {
Write-Host "Param2 value is $Param2"
}
Output:
Splatting in PowerShell Function Parameters
Splatting is mainly used when there are a lot of parameters. Setting up parameters for a script before running a command is referred to as splatting. You’ve been defining and passing values to parameters at runtime throughout up to now. Once you have a collection of parameters that appear below, this can be a problem.
param(
[Parameter()]
[string]$Param1,
[Parameter()]
[string]$Param2,
[Parameter()]
[switch]$DisplayParam2,
[Parameter()]
[string]$Param3,
[Parameter()]
[string]$Param4,
[Parameter()]
[string]$Param5,
[Parameter()]
[string]$Param6,
[Parameter()]
[string]$Param7
)
Write-Host "Param1 value is $Param1"
if ($DisplayParam2.IsPresent) {
Write-Host "Param2 value is $Param2"
}
Write-Host "Param3 value is $Param3"
Write-Host "Param4 value is $Param4"
Write-Host "Param5 value is $Param5"
Write-Host "Param6 value is $Param6"
Write-Host "Param7 value is $Param7"
Rather than sliding to the right, you might use a hashtable to specify the parameter values in a separate phase, as illustrated below. Parameter values may be neatly aligned, making it far easier to understand which ones are in use.
Once the hashtable is established, you can specify the name of the hashtable followed by a @
character to send all of the arguments to the script.
$params = @{
Param1 = 'value'
Param2 = 'valuenext'
Param3 = 'somevalue'
Param4 = 'somevalue'
Param5 = 'somevalue'
Param6 = 'somevalue'
Param7 = 'somevalue'
DisplayParam2 = $true
}
PS> ./test.ps1 @params
PowerShell Parameter Attributes
Parameter characteristics allow you to change parameter functionality in a variety of ways. You may use regular expressions to fit a regular expression, mandate the usage of a given parameter, and verify the values supplied to a parameter.
Mandatory Parameters
In PowerShell, we can mandate one or more attributes running in a function based on our wish. Therefore, we can use the mandatory
attribute inside the Parameter()
construct.
param(
[Parameter(Mandatory)]
[string]$Param1,
[Parameter()]
[string]$Param2,
[Parameter()]
[switch]$DisplayParam2
)
Write-Host "Param1 value is $Param1"
if ($DisplayParam2.IsPresent) {
Write-Host "Param2 value is $Param2"
}
Output:
Parameter Validation
Finally, double-check that the values supplied to parameters are correct. It’s ideal for keeping the possibility of someone giving a unique value to a parameter to a minimum. Therefore, we can specify the inputs we want using the ‘ValidateSet()’ as shown below.
param(
[Parameter(Mandatory)]
[ValidateSet('x', 'y')]
[string]$Param1,
[Parameter()]
[string]$Param2,
[Parameter()]
[switch]$DisplayParam2
)
Write-Host "Param1 value is $Param1"
if ($DisplayParam2.IsPresent) {
Write-Host "Param2 value is $Param2"
}
If we give values other than x and y to param1, an error will pop up.
Advanced Function With Named Parameter Syntax
When the Cmdletbinding attribute is added to a function, it turns it into an advanced function. Other common arguments are used when the advanced function is constructed. The syntax is as follows.
function testfunction {
[CmdletBinding (DefaultParameterSetName = 'Param1')]
Param
(
[Parameter(Mandatory = $true, Position = 0, ParameterSetName = 'Param1')]
[string] Param1,
[Parameter(Mandatory = $true, Position = 0, ParameterSetName = 'Param2')]
[string] Param2,
)
}
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.