How to Create Tables in PowerShell
- Use Hash Table to Create Tables in PowerShell
-
Use the
DataTable
Object to Create Tables in PowerShell -
Use
Format-Table
to Create Tables in PowerShell - Conclusion
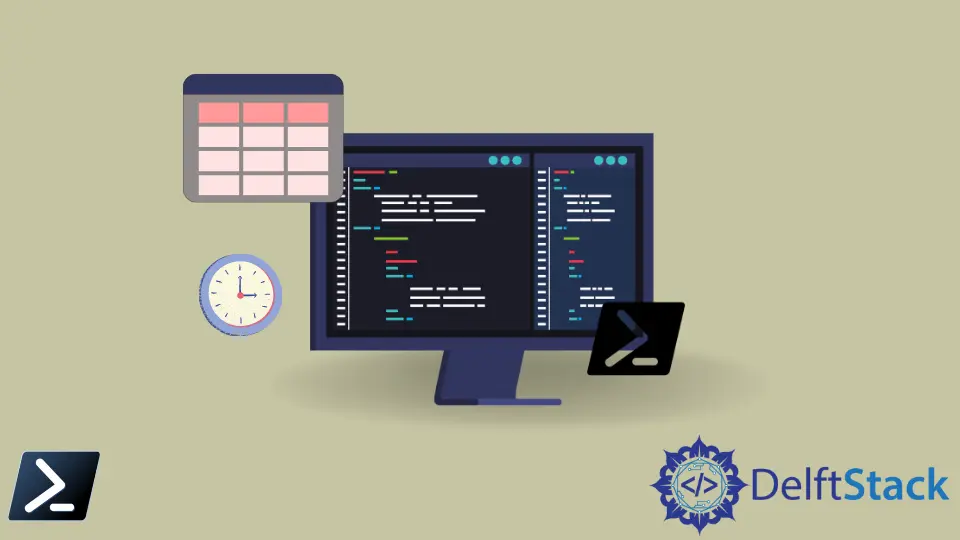
Organizing and displaying data in a clear and structured manner is essential, and tables are a widely recognized format for achieving this. In PowerShell, as in any programming language, the ability to create tables is fundamental.
Creating custom tables is a simple process in PowerShell. This article explores the different methods available for creating tables in PowerShell, making data presentation more effective.
Use Hash Table to Create Tables in PowerShell
A hash table is a compact data structure that stores each value using a key. It is also known as a dictionary or associative array.
In PowerShell, you can create a hash table using the @{}
syntax. The keys and values are enclosed in curly braces {}
.
Code:
$hash = @{Name = "brian"; Age = "23"; Location = "UK" }
In the code above, create a hash table using the @
symbol followed by curly braces { }
. Inside the curly braces, we have three key-value pairs separated by semicolons.
Each key is a string (Name
, Age
, Location
) followed by an equal sign (=
) and corresponding values.
So, this line creates a hash table with the following key-value pairs:
- Key
Name
with the value"brian"
. - Key
Age
with the value"23"
. - Key
Location
with the value"UK"
.
Display the contents of the hash table using the variable $hash
.
$hash
Output:
Name Value
---- -----
Name brian
Age 23
Location UK
A hash table is displayed in the tabular format, having one column for keys and another for values.
However, it’s worth noting that hash tables are limited to displaying only two columns: one for keys and one for values. If your requirements involve more columns or columns with different names, hash tables may not be the most effective choice.
Use the DataTable
Object to Create Tables in PowerShell
The DataTable
object is very useful when working with tables in PowerShell. You can create a DataTable
using the command New-Object System.Data.Datatable
.
You can use $TableName.Columns.Add("ColumnNames")
to add columns and $TableName.Rows.Add("ValueToColumns")
to add rows in the table.
In the following example, we created a new DataTable
($table
) object from the System.Data.DataTable
class.
We then add three columns and three rows to the DataTable
($table
) object. The [void]
is used to suppress the output of these commands.
Lastly, we display the contents of the DataTable
object using the variable $table
.
Code:
$table = New-Object System.Data.DataTable
[void]$table.Columns.Add("Name")
[void]$table.Columns.Add("Age")
[void]$table.Columns.Add("Location")
[void]$table.Rows.Add("brian", "23", "UK")
[void]$table.Rows.Add("sam", "32", "Canada")
[void]$table.Rows.Add("eric", "25", "USA")
$table
Output:
Name Age Location
---- --- --------
brian 23 UK
sam 32 Canada
eric 25 USA
In the output, you’ll see the generated table using the DataTable
object. While this method allows you to create tables with multiple columns and custom column names, you must manually add both the columns and their corresponding values.
Use Format-Table
to Create Tables in PowerShell
You can use the Format-Table
cmdlet to format and display data as a table in the console. While it doesn’t create a table object, it’s useful for presenting data in a tabular format in the console.
Code:
Get-Process | Format-Table -Property Name, Id, CPU
In the example above, we use the Get-Process
to retrieve information about running processes. The output of Get-Process
is a list of running processes.
We then pipe the output of Get-Process
to the Format-Table
cmdlet for formatting. We want to display the specific properties of each process in a table format.
Next, we use the -Property
parameter to specify the properties to include in the table. In this case, we want to include Name
, Id
, and CPU
properties.
Output:
Name Id CPU
---- -- ---
ACCStd 4084 11.15625
ACCSvc 2620
amdfendrsr 2596
AMDRSServ 10772 47.6875
The output displays information about running processes. Each row represents a different process.
Please note that not all processes have a value in the CPU
column. Some processes may not consume CPU resources, so their CPU
column remains empty in the output.
Conclusion
Creating tables in PowerShell is easy and versatile, catering to various data presentation needs. You have options like hash tables for basic key-value pairs, the DataTable
object for more advanced tables with multiple columns and custom names, and the Format-Table
cmdlet for displaying data neatly in the console.
These methods empower you to enhance the readability and organization of your data, ultimately making your PowerShell scripts more effective and user-friendly.