How to Convert XML to JSON in PowerShell
-
Using
XPath Selection
,Hash Table
, andConvertTo-Json
to Convert XML to JSON in PowerShell -
Using
ConvertTo-Json
andConvertFrom-Xml
Cmdlets to Convert XML to JSON in PowerShell - Conclusion
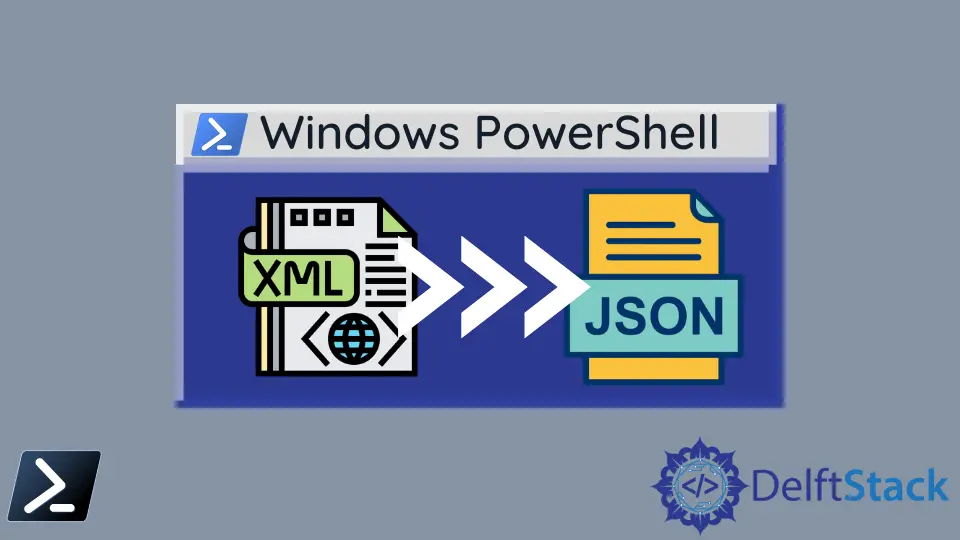
This comprehensive guide delves into the conversion of XML documents to JSON formatted strings using PowerShell, a powerful scripting language and command-line shell. The XML and JSON formats are fundamental in data exchange and storage, especially in environments where configuration and data processing are pivotal.
PowerShell, with its robust set of cmdlets, simplifies this conversion process. We explore two primary methods in this guide: the first involving a combination of XPath Selection
, Hash Table
, and ConvertTo-Json
, and the second utilizing ConvertTo-Json
and ConvertFrom-Xml
cmdlets.
The guide provides a step-by-step approach, starting with accessing XML document keys and values, constructing a PowerShell hash to hold the data, and finally converting the hash to JSON. Each method is illustrated with detailed examples and syntax, ensuring a clear understanding of the processes involved.
Using XPath Selection
, Hash Table
, and ConvertTo-Json
to Convert XML to JSON in PowerShell
Access XML Document Keys and Values
PowerShell enables manipulating XML document nodes using the Select-Xml
cmdlet. We can specify XPath
expressions to find nodes and their text values in an XML document.
Syntax:
Select-Xml
[-XPath] <string>
[-Path] <string[]>
[-Namespace <hashtable>]
[<CommonParameters>]
Let’s create an XML document called employeeinfo.xml
with the following content.
XML - employeeinfo.xml
:
<?xml version="1.0" encoding="utf-8"?>
<Employees>
<Employee>
<EmpId>1</EmpId>
<EmpAge>45</EmpAge>
<EmpDesignation>SSE</EmpDesignation>
</Employee>
<Employee>
<EmpId>2</EmpId>
<EmpAge>34</EmpAge>
<EmpDesignation>Junior SE</EmpDesignation>
</Employee>
</Employees>
Now, we are going to access each XML node EmpId
, EmpAge
, and EmpDesignation
using the Select-Xml
cmdlet as follows.
Code:
$empIds = Select-Xml -Path "D:\codes\employeeinfo.xml" -XPath "//Employee//EmpId" | foreach { $_.node.InnerText }
$empAges = Select-Xml -Path "D:\codes\employeeinfo.xml" -XPath "//Employee//EmpAge" | foreach { $_.node.InnerText }
$empDesigs = Select-Xml -Path "D:\codes\employeeinfo.xml" -XPath "//Employee//EmpDesignation" | foreach { $_.node.InnerText }
In this example, the -Path
is the location where the employeeinfo.xml
is located. Since we need to fetch three nodes per employee object, PowerShell foreach
has been used.
The XPath
expression looks like the following for each node. EmpID
node can be accessed by the XPath
query "//Employee//EmpId"
.
EmpAge
node can be accessed by the XPath
query "//Employee//EmpIAge"
. EmpDesignation
node can be accessed by the XPath
query "//Employee//EmpDesignation"
.
The reference variables, $empIds
, $empAges
, and $empDesigs
, contain an array of values for respective XML nodes. Let’s write the arrays into PowerShell console windows.
Construct PowerShell Hash to Hold Data
We do not have a straightforward approach to converting an XML document to a JSON string. Hence, as shown in the above section, we have to extract XML data and push those data to a PowerShell hash as an intermediate format.
Let’s construct the hash.
$empObjHash1 = @{
EmpId = $empIds[0];
EmpAge = $empAges[0];
EmpDesignation = $empDesigs[0];
}
$empObjHash2 = @{
EmpId = $empIds[1];
EmpAge = $empAges[1];
EmpDesignation = $empDesigs[1];
}
$finalHash = @{}
$finalHash.Add("emp1", $empObjHash1)
$finalHash.Add("emp2", $empObjHash2)
We have extracted the two employee objects from the XML for demonstration purposes and pushed those data to two hash objects, $empObjHash1
and $empObjHash2
. Then, the $finalHash
is created by pushing the two employee objects.
If we print the $finalHash
, it would look like the following.
We can use the foreach
loop to inspect the $finalHash
in more detail.
Code:
foreach ($em in $finalHash.keys) {
foreach ($emp in $finalHash[$em]) {
foreach ($val in $emp.keys) {
Write-Host "Key:" $val "Value:" $emp[$val]
}
}
}
Output:
The $finalHash
has been constructed with the relevant data extracted from the XML.
Convert Hash to JSON
Since we got a PowerShell hash $finalHash
, it is possible to use the ConvertTo-Json
cmdlet to create a JSON out of the hash.
Syntax:
ConvertTo-Json
[-InputObject] <Object>
[-Depth <Int32>]
[-Compress]
[-EnumsAsStrings]
[-AsArray]
[-EscapeHandling <StringEscapeHandling>]
[<CommonParameters>]
Let’s pass the created hash $finalHash
to create the JSON string, as shown in the following.
Code:
$finalHash | ConvertTo-Json
Output:
PowerShell Full Script:
$empIds = Select-Xml -Path "D:\codes\employeeinfo.xml" -XPath "//Employee//EmpId" | foreach { $_.node.InnerText }
$empAges = Select-Xml -Path "D:\codes\employeeinfo.xml" -XPath "//Employee//EmpAge" | foreach { $_.node.InnerText }
$empDesigs = Select-Xml -Path "D:\codes\employeeinfo.xml" -XPath "//Employee//EmpDesignation" | foreach { $_.node.InnerText }
$empObjHash1 = @{
EmpId = $empIds[0];
EmpAge = $empAges[0];
EmpDesignation = $empDesigs[0];
}
$empObjHash2 = @{
EmpId = $empIds[1];
EmpAge = $empAges[1];
EmpDesignation = $empDesigs[1];
}
$finalHash = @{}
$finalHash.Add("emp1", $empObjHash1)
$finalHash.Add("emp2", $empObjHash2)
foreach ($em in $finalHash.keys) {
foreach ($emp in $finalHash[$em]) {
foreach ($val in $emp.keys) {
Write-Host "Key:" $val "Value:" $emp[$val]
}
}
}
$finalHash | ConvertTo-Json
Using ConvertTo-Json
and ConvertFrom-Xml
Cmdlets to Convert XML to JSON in PowerShell
In PowerShell, converting data from XML to JSON format is a common requirement, particularly when interfacing with web APIs or modern web services. PowerShell simplifies this task with its ConvertTo-Json
and ConvertFrom-Xml
cmdlets.
Syntax:
-
Get-Content
: Reads content from a file.-Path <String>
: Specifies the path of the file.
-
ConvertTo-Json
: Converts an object to a JSON-formatted string.-InputObject <Object>
: Specifies the object to convert.-Depth <Int32>
: Specifies the depth of object hierarchies to include.
Example
# Path to the XML file
$xmlFilePath = "employeeinfo.xml"
# Read the content of the XML file and convert to XML object
$xml = New-Object XML
$xml.Load($xmlFilePath)
# Parse the XML and create an object array
$employeeArray = @()
foreach ($employee in $xml.Employees.Employee) {
$empObject = [PSCustomObject]@{
EmpId = $employee.EmpId
EmpAge = $employee.EmpAge
EmpDesignation = $employee.EmpDesignation
}
$employeeArray += $empObject
}
# Convert the array to JSON
$json = $employeeArray | ConvertTo-Json
# Display the result
Write-Output $json
In our script, we first specify the path to the XML file using the $xmlFilePath
variable. Using Get-Content
, we read the contents of this file into the $xmlContent
variable.
This content is then cast to an [xml]
type, creating a structured XML object ($xmlObject
). This object is passed to ConvertTo-Json
to perform the conversion to JSON format.
Finally, we use Write-Output
to print the JSON string.
Output:
Conclusion
In conclusion, this guide has successfully demonstrated the practical and efficient methods of converting XML documents to JSON strings using PowerShell. By showcasing two distinct approaches - one involving the extraction of XML data into a PowerShell hash and another utilizing the ConvertFrom-Xml
cmdlet - the guide provides versatile options catered to different user needs and scenarios.
The step-by-step instructions, coupled with relevant code snippets and outputs, enable readers to grasp the concept readily and apply these methods in real-world situations. The flexibility and power of PowerShell in handling and transforming data formats are evident, making it an indispensable tool for administrators and developers dealing with XML and JSON data transformations.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.