PowerShell Contains Operator
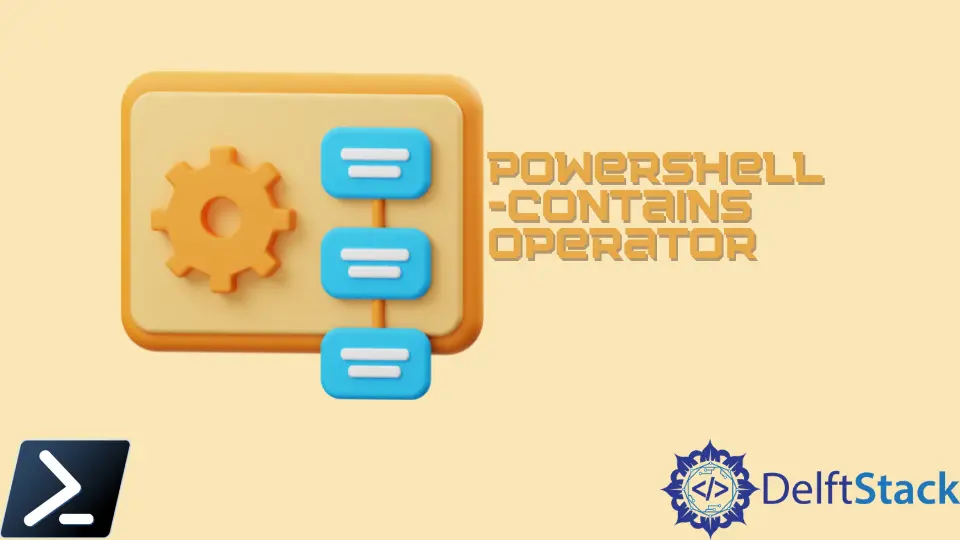
There are different operators available in the PowerShell that can filter/compare or find elements that match a specified input string. The -contains
is one of the major comparison operators, classified as a Containment type operator.
There are four main containment type operators.
-contains
-notcontains
-in
-notin
In this article, we are only focusing on the -contains
operator. This operator always returns a Boolean value (true/false) if there is a match. Also, the performance-wise, -contains
operator is pretty fast to return the results because it stops comparing the input as soon as it finds the first match.
the -contains
Operator in PowerShell
This operator can be used to check whether a collection contains a specific element. Its syntax is as below.
[set / collection] -contains [test-value or test-object]
[set/collection]
can be a set of string values (comma separated), for example, "Hello"
or "FOX", "2ndLane"
.
[test-value or test-object]
can be an element or set of elements (collection), for example, "Hello"
or "Hello", "FOX", "No2"
.
Check for a Certain Element
- Example 01:
"Hello", "FOX", "2ndLane" -contains "2ndLane"
Output:
True
The input element/value is "2ndLane"
and it can be located in the left-hand side collection/set. Therefore, the output/result is obviously True
.
- Example 02:
"Hello", "FOX", "2ndLane" -contains "NotInTheCollection"
Output:
False
The input element/value is "NotInTheCollection"
and it is not included in the right-hand side collection. Therefore, the above command was evaluated to False
.
The important fact about the -contains
operator is that it checks for the exact input element within the given collection/set. The command will be evaluated as False
when a portion/substring has been given as the input element.
- Example 03:
"Hello", "FOX", "FullStringGiven" -contains "StringGiven"
Output:
False
In the above example, the input element is the "StringGiven"
, but it is a substring of the "FullStringGiven"
element of the right-hand side collection. Therefore, the input element doesn’t match an exact element from the right-hand side collection, and the result is False
as expected.
Check for a Set of Elements/Collection With the -contains
Operator in PowerShell
One of the biggest advantages of the -contains
operator is that it can be used to find whether the given collection/set matches an input collection. It is important to keep in mind that the operator checks whether the same instances exist on the left-hand side (given collection) and the right-hand side (input collection/test-collection). That means these containment operators use reference equality when the input object (test-object) is a collection.
Example 01
$leftsideobj = "Hello", "NewString1"
Here, we assign the $leftsideobj
variable to the set of elements (collection).
$leftsideobj, "AnotherString" -contains $leftsideobj
Then, we use -contains operator to find a match.
Output:
True
This command has been evaluated to True
. Because the input collection is $leftsideobj
and the same instance is available in the left-hand side collection. It means the reference equality has been fulfilled. Therefore, the result is True
.
Example 02
$newleftsideobj = "Hello", "Test"
Here, we got the $newleftsideobj
variable assigned to a collection that contains "Hello"
and "Test"
elements.
`"Hello", "Test", "NewString1" -contains $newleftsideobj`
Output:
False
The above command was evaluated to False
. You can see that the input collection (right-hand side) is $newleftsideobj
, which indirectly the two elements "Hello"
and "Test"
. If you notice the left-hand side, we got "Hello"
and "Test"
elements available. But it doesn’t satisfy the reference equality. That is why the output is False
.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.