How to Negate a Condition in PowerShell
-
Use the
-not
Operator to Negate a Condition in the PowerShell -
Use the
!
Operator to Negate a Condition in the PowerShell -
Use the
-notmatch
Operator to Negate a Condition in the PowerShell -
Use the
-ne
Operator to Negate a Condition in the PowerShell -
Use the
-notlike
Operator to Negate a Condition in the PowerShell - Conclusion
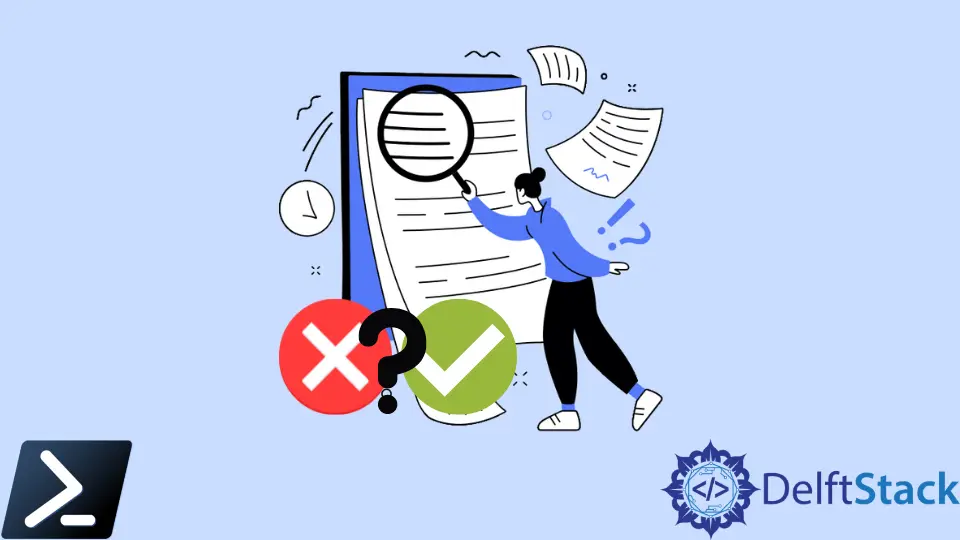
PowerShell has different decision-making statements for executing the codes like other programming languages. You can use conditions for decision-making in the PowerShell scripts.
The scripts perform different actions based on those decisions. If the condition is true
, it will execute one command, and if the condition is false
, it will execute the other command.
One of the most used statements in PowerShell is the If
statement. It has three types: an if
statement, an if-else
statement, and a nested if
statement.
PowerShell also uses a switch
statement as a conditional statement.
Here is a simple example of the if
statement.
if (5 -lt 7) {
Write-Host "5 is less than 7"
}
If 5
is less than 7, it executes the Write-Host
command.
Output:
The logical operators connect the conditional statements in the PowerShell, which allows you to test for multiple conditions. The PowerShell supports -and
, -or
, -xor
, -not
, and !
logical operators.
This tutorial will teach you to negate a condition in the PowerShell.
Use the -not
Operator to Negate a Condition in the PowerShell
In PowerShell, the -not
operator is a powerful tool for negating conditions. It allows you to easily reverse the outcome of a logical expression, making your scripts more dynamic and flexible.
$condition = $true
if (-not $condition) {
Write-Host "Condition is false"
}
In this example, we initialize the $condition
to $true
. When we apply the -not
operator to the $condition
, it evaluates to $false
since the original condition was true. Consequently, the message "Condition is false"
is not printed.
Output:
Now, let’s test another condition.
$value = 5
if (-not ($value -eq 10)) {
Write-Host "Value is not equal to 10"
}
In this example, we set $value
to 5
. We use the -eq
operator to check if $value
equals 10
, but with the -not
operator, we negate this condition. Consequently, the expression ($value -eq 10)
evaluates to $false
, and the -not
operator transforms it into $true
.
As a result, we print the message "Value is not equal to 10"
.
Output:
Use the !
Operator to Negate a Condition in the PowerShell
The !
operator, also known as the logical NOT
operator, is used to negate a condition. It essentially reverses the truth value of a boolean expression.
This operator is commonly used when you need to check if a condition is false rather than true.
$condition = $true
if (!$condition) {
Write-Host "Condition is false"
}
In this example, we initialize the $condition
to $true
. When we apply the !
operator to the $condition
, it evaluates to $false
since the original condition was true. Consequently, the message "Condition is false"
is not printed.
Output:
Use the -notmatch
Operator to Negate a Condition in the PowerShell
In PowerShell, the -notmatch
operator is used to negate a condition based on pattern matching. This operator checks whether a string does not match a specified pattern.
It’s particularly useful when you want to exclude certain strings or patterns from your selection.
$string = "Hello, world!"
if ($string -notmatch "Goodbye") {
Write-Host "String does not contain 'Goodbye'"
}
In this example, we have a string $string
containing the value "Hello, world!"
. We use the -notmatch
operator to check if this string does not match the pattern "Goodbye"
.
Since the string "Hello, world!"
does not contain the substring "Goodbye"
, the condition is true, and the message "String does not contain 'Goodbye'"
is printed.
Output:
Use the -ne
Operator to Negate a Condition in the PowerShell
In PowerShell, the -ne
operator is used to negate a condition based on inequality. It checks whether two values are not equal to each other.
This operator is particularly useful when you need to exclude certain values from your comparisons.
$value = 5
if ($value -ne 10) {
Write-Host "Value is not equal to 10"
}
In this example, we assign the value 5
to the variable $value
. We then use the -ne
operator to check if $value
is not equal to 10
.
Since $value
is indeed 5
, which is not equal to 10
, the condition evaluates to true, and the message "Value is not equal to 10"
is printed.
Output:
Use the -notlike
Operator to Negate a Condition in the PowerShell
In PowerShell, the -notlike
operator is utilized to negate a condition based on wildcard pattern matching. It allows you to check if a string does not match a specified pattern.
This operator is beneficial when you need to exclude certain patterns or substrings from your comparisons.
$string = "Hello, world!"
if ($string -notlike "*Goodbye*") {
Write-Host "String does not contain 'Goodbye'"
}
In this example, we have a string $string
containing the value "Hello, world!"
. We use the -notlike
operator to check if this string does not match the pattern "*Goodbye*"
.
Since the string "Hello, world!"
does not contain the substring "Goodbye"
, the condition is true, and the message "String does not contain 'Goodbye'"
is printed.
Output:
Conclusion
In this article, we explored various methods to negate conditions in PowerShell, providing flexibility and precision in script logic. We began by discussing the -not
operator, which reverses the outcome of a logical expression, followed by the !
operator, known as the "logical NOT"
operator, used for negating conditions directly.
We then delved into the -notmatch
operator, which checks if a string does not match a specified pattern, and the -ne
operator, which evaluates inequality between two values. Lastly, we covered the -notlike
operator, utilized for negating conditions based on wildcard pattern matching.
By understanding and applying these methods, PowerShell users can enhance the robustness and versatility of their scripts.