Multiple Values on a Parameter in PowerShell
-
Understanding the
Parameter
Function in PowerShell -
Use the
Named
Parameters in PowerShell - Using Defaults to a Parameter in PowerShell
-
Using
Switch
Parameters in PowerShell -
Use the
Mandatory
Parameters in PowerShell - Using an Unknown Number of Arguments in PowerShell
- Using the Pipeline Parameters in PowerShell
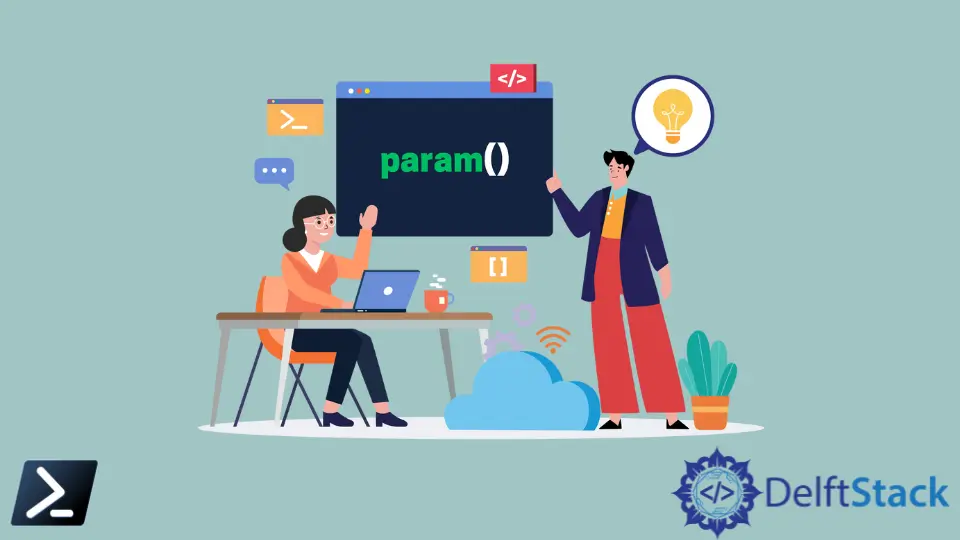
We handle arguments using the PowerShell parameter function param
. It is a fundamental component of any script, enabling developers to provide input at runtime.
In addition, if a script’s behavior needs to change, a parameter can offer an opportunity to do so without changing the underlying code.
This article will discuss the parameter
function, different variables that we can define, handle multiple values in a single parameter, and sample uses.
Understanding the Parameter
Function in PowerShell
Administrators can create parameters for scripts using the parameter function param()
.
Inside, the parameter function contains one or more parameters defined by variables.
param ($myVariable)
However, to ensure that the parameter accepts only the type of input you need, it is best to assign a data type to the parameter by enclosing the data type with square brackets []
before the variable.
param ([String]$myVariable)
It is worth noting that you can also use other data types like integers
, float
, and DateTime
. We can also assign it with a data type Boolean
which we will discuss further.
Use the Named
Parameters in PowerShell
Using a parameter function in a script is via named
parameters.
When passing values to a script or function via named
parameters, we can use the variable’s name as the full name of the parameter.
sample.ps1
:
param ([String]$name)
Write-Output $name
We can then use the named parameters when executing a .ps1
file.
powershell.exe .\sample.ps1 -name "John"
Using Defaults to a Parameter in PowerShell
We can pre-assign a default value to a parameter by giving the parameter a value inside of the script.
In addition, executing the script without passing values from the command line will take the default variable defined inside the script.
param ([String]$name = "John")
Using Switch
Parameters in PowerShell
We can use another parameter type for our scripts: the switch
parameter defined by the [switch]
data type.
This parameter is mainly used for binary or Boolean values that we have discussed before, indicating the value of true
or false
.
param ([switch]$isEnabled)
Use the Mandatory
Parameters in PowerShell
It’s common in some of our use cases to have one or more parameters that must be used when a script is executed.
We can define a mandatory parameter by adding a Mandatory
attribute inside the parameter block [Parameter()]
.
param (
[Parameter(Mandatory)]
[String] $servername
)
The scripting environment will not allow the script to run unless a value has been provided in the command line.
Using an Unknown Number of Arguments in PowerShell
Generally, I find using named parameters far superior to merely reading the arguments from the command line. Reading the arguments is easier when you need to handle an unknown number of arguments.
Let’s take drive letters as an example. Perhaps we need a script to check one or more drives in the machine.
Then, we can use a named parameter and declare it as a String
data type.
checkdrives.ps1
:
param([String]$myDrive)
foreach ($drive in $myDrive) {
Write-Output "Processing drive $drive"
}
However, passing multiple drive letters into the argument, PowerShell will treat it as one object.
powershell .\checkdrives.ps1 -myDrive C, D, E
Output:
Processing drive C D E
PowerShell will replace the commas with spaces and treat them as one variable. Unfortunately, this wouldn’t work since we need to process the drives individually in our use case.
For our interpreter to capture multiple parameters under one argument, we need to change the data type as an array with square brackets []
. Then, when executed, PowerShell will process the arguments listed individually.
checkdrives.ps1
:
param([String[]]$myDrive)
foreach ($drive in $myDrive) {
Write-Output "Processing drive $drive"
}
Example Code:
powershell .\checkdrives.ps1 -myDrive C, D, E
Remember to separate the arguments with a comma.
Output:
Processing drive C
Processing drive D
Processing drive E
Using the Pipeline Parameters in PowerShell
Most PowerShell cmdlets let you use the pipe |
symbol of passing data. We can take advantage of this when it comes to parameters.
For example, we can get the last value stored in the pipeline and pass it to another PowerShell file using the ValueFromPipeline
attribute of the parameter block.
pipeline.ps1
:
param([parameter(ValueFromPipeline)]$pipedVar)
Write-Output $pipedVar
Example Code:
"This string is from the pipeline." | .\pipeline.ps1
Output:
This string is from the pipeline.
Doing this technique opens up many opportunities for running chained PowerShell files.
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn